This part is very similar to the first one. So, if you got that, this should be straightforward. A Car is composed of four Wheel thingies. If a car is moving, all its wheels are moving (if nothing else has gone wrong). 2.1 Wheels for CarsLet’s first do wheels! You need to define a Wheel class. At the moment, this class should have just a single variable state of type string. Make sure this variable is hidden from those outside the class! Now, within this class, define a public function with the following declaration: void set_wheel_state (string s); This function should take set the state of the wheel to the value passed in as s. Write another function in this class with the following declaration: string get_wheel_state () This should simply return the state of the wheel. After this, you should be able to run Part -3 of the main function in the starter file. 2.2 Cars on Wheels Once we have a Wheel class, we can use it within our Car class. Define a new class Car. This should have an array of wheels – the size of the array should be 4 since we have only four wheels. Make sure this array is not visible from outside the class. Next, write a function set_car_to_moving within this class. This function should set the states of all four wheels to "Turning". That’s it! Write another function set_car_to_stopped same as above except the state of all four wheels should be set to "Stopped". Finally, write a function print_car_wheels_state in this class which outputs the state of all four wheels in the following format : Car state: - Wheel 0 is Stopped Wheel 1 is Stopped Wheel 2 is Stopped Wheel 3 is Stopped Of course, if the car is moving, all output lines should have the word Turning there. Make sure you use the get_wheel_state function of the wheel you defined earlier for this. After this, you should be able to run Part -4 of the main function in the starter file.
This part is very similar to the first one. So, if you got that, this should be straightforward. A Car is composed of four Wheel thingies. If a car is moving, all its wheels are moving (if nothing else has gone wrong).
2.1 Wheels for CarsLet’s first do wheels!
- You need to define a Wheel class. At the moment, this class should have just a single variable state of type string. Make sure this variable is hidden from those outside the class!
- Now, within this class, define a public function with the following declaration:
void set_wheel_state (string s);
This function should take set the state of the wheel to the value passed in as s.
- Write another function in this class with the following declaration:
string get_wheel_state ()
This should simply return the state of the wheel. After this, you should be able to run Part -3 of the main function in the starter file.
2.2 Cars on Wheels
Once we have a Wheel class, we can use it within our Car class.
- Define a new class Car. This should have an array of wheels – the size of the array should be 4 since we have only four wheels. Make sure this array is not visible from outside the class.
- Next, write a function set_car_to_moving within this class. This function should set the states of all four wheels to "Turning". That’s it!
- Write another function set_car_to_stopped same as above except the state of all four wheels should be set to "Stopped".
- Finally, write a function print_car_wheels_state in this class which outputs the state of all four wheels in the following format :
Car state: -
Wheel 0 is Stopped
Wheel 1 is Stopped
Wheel 2 is Stopped
Wheel 3 is Stopped
Of course, if the car is moving, all output lines should have the word Turning there. Make sure you use the get_wheel_state function of the wheel you defined earlier for this. After this, you should be able to run Part -4 of the main function in the starter file.

Step by step
Solved in 3 steps with 1 images

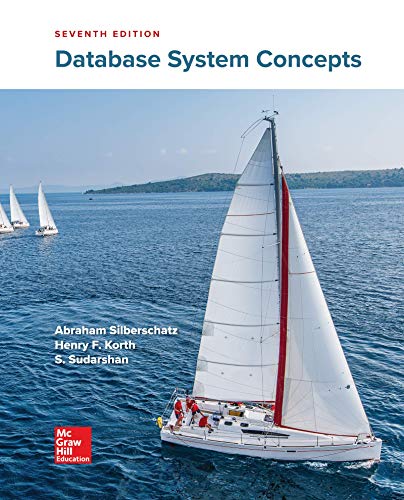
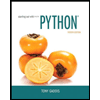
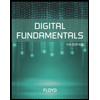
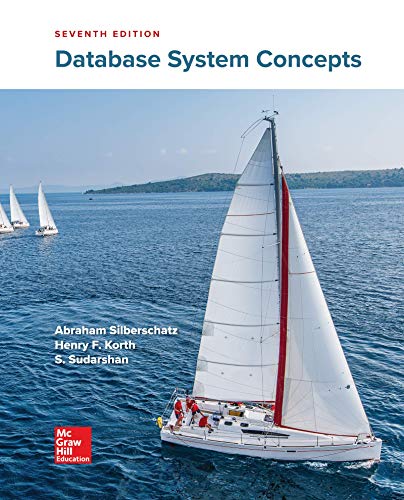
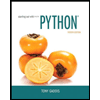
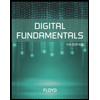
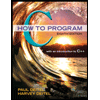
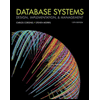
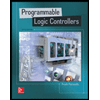