All vehicles used for transportation in the U.S. must have identification, which varies according to the type of vehicle. For example, all automobiles have a unique Vehicle Identification Number (VIN) assigned by the manufacturer, plus a license plate number assigend by the state in which the auto is registerd. Modify the Auto class to include an instance variable for the license plate number. Implement the constructor so that an Auto can be constructed with a VIN and a license plate number. Override the getID() method to return the id of the auto as shown in this format: VIN=1234567890,plate=ABC123 (without any spaces). ** Represents an automobile. */ public class Auto // TODO: Inherit from Vehicle { // TODO: Declare instance variables public Auto(String vin, String plate) { // TODO: Complete the constructor } // TODO: implement the getID() method for autos }
All vehicles used for transportation in the U.S. must have identification, which varies according to the type of vehicle. For example, all automobiles have a unique Vehicle Identification Number (VIN) assigned by the manufacturer, plus a license plate number assigend by the state in which the auto is registerd.
Modify the Auto class to include an instance variable for the license plate number. Implement the constructor so that an Auto can be constructed with a VIN and a license plate number.
Override the getID() method to return the id of the auto as shown in this format: VIN=1234567890,plate=ABC123 (without any spaces).
**
Represents an automobile.
*/
public class Auto // TODO: Inherit from Vehicle
{
// TODO: Declare instance variables
public Auto(String vin, String plate)
{
// TODO: Complete the constructor
}
// TODO: implement the getID() method for autos
}
![```java
/**
Represents a vehicle of any type.
*/
public class Vehicle
{
private String id;
private double mileage;
public Vehicle(String anId)
{
id = anId;
mileage = 0;
}
public void move(double milesMoved)
{
mileage = mileage + milesMoved;
}
public String getID()
{
return id;
}
public double getMileage()
{
return mileage;
}
}
```
```java
public class VehicleTester
{
public static void main(String[] args)
{
Vehicle myVehicle = new Auto("0149162536496481100", "5ZN090");
myVehicle.move(1000);
myVehicle.move(2000);
System.out.println(myVehicle.getID());
System.out.println("Expected: VIN=0149162536496481100,plate=5ZN090");
System.out.println(myVehicle.getMileage());
System.out.println("Expected: 3000.0");
}
}
```
### Explanation:
This code consists of two Java classes: `Vehicle` and `VehicleTester`.
1. **Vehicle Class:**
- **Purpose:** Represents a generic vehicle with an identifier and mileage.
- **Attributes:**
- `id`: A `String` representing the vehicle's unique identifier.
- `mileage`: A `double` representing the vehicle's accumulated mileage.
- **Constructor:**
- Initializes the vehicle's `id` and sets the initial `mileage` to 0.
- **Methods:**
- `move(double milesMoved)`: Increments the `mileage` by the specified `milesMoved`.
- `getID()`: Returns the vehicle's `id`.
- `getMileage()`: Returns the vehicle's `mileage`.
2. **VehicleTester Class:**
- **Purpose:** Tests the functionality of the `Vehicle` class.
- **Main Method:**
- Creates a new `Vehicle` object named `myVehicle` using a hypothetical `Auto` constructor (parameters imply it is a subclass of `Vehicle`).
- Moves `myVehicle` by 1000 and then 2000 miles.
- Prints the vehicle's `ID` and mileage, followed by the expected output for verification.
### Notes:
- In `VehicleTester`, `Auto` is assumed to](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F1580620b-ec3d-48da-8d5f-e0e1d6dd1757%2Fcfaf30a5-052a-425a-897a-6596d79f4bcb%2Fb6bxv6l_processed.png&w=3840&q=75)

Step by step
Solved in 2 steps

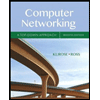
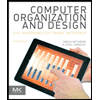
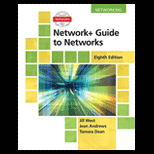
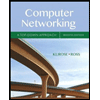
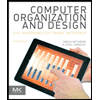
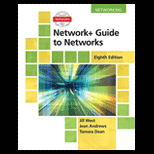
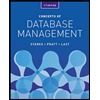
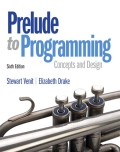
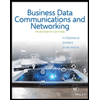