// This main method provides code to run tests on the play() method. // You are free to add code, delete code, change code, etc., in main // as long as it compiles.
Solution in Java:
The play() method comes from a word game. Implement the play() method as described in code comments.
import java.util.LinkedList;
import java.util.ListIterator;
public class WordGamePlay {
public static void main(String[] args) {
// This main method provides code to run tests on the play() method.
// You are free to add code, delete code, change code, etc., in main
// as long as it compiles.
LinkedList<String> words = new LinkedList<String>();
words.add("file");
words.add("edit");
words.add("refactor");
words.add("free");
words.add("source");
words.add("sleeps");
String word;
ListIterator<String> it;
System.out.println();
word = "rampages";
System.out.println(" List: " + words);
System.out.println(" Testing: " + word);
it = words.listIterator();
play(it, word);
System.out.println(" Result: " + words);
System.out.println("Expected: [file, edit, free, source, sleeps]");
System.out.println();
word = "pounce";
System.out.println(" List: " + words);
System.out.println(" Testing: " + word);
it = words.listIterator();
play(it, word);
System.out.println(" Result: " + words);
System.out.println("Expected: [pounce, file, edit, free, source, sleeps]");
System.out.println();
word = "flay";
System.out.println(" List: " + words);
System.out.println(" Testing: " + word);
it = words.listIterator();
play(it, word);
System.out.println(" Result: " + words);
System.out.println("Expected: [pounce, edit, source, sleeps]");
System.out.println();
word = "stay";
System.out.println(" List: " + words);
System.out.println(" Testing: " + word);
it = words.listIterator();
play(it, word);
System.out.println(" Result: " + words);
System.out.println("Expected: [stay, pounce, edit, source, sleeps]");
System.out.println();
word = "sprays";
System.out.println(" List: " + words);
System.out.println(" Testing: " + word);
it = words.listIterator();
play(it, word);
System.out.println(" Result: " + words);
System.out.println("Expected: [stay, pounce, edit]");
}
// play method
// inputs:
// - an iterator guaranteed to be positioned at the beginning of a list of words
// - a word (String)
// output: none (void method)
// actions:
// - determine if there are any words in the list with all these criteria:
// - same length as input word
// - starts with same letter as input word
// - if so : remove all such words from the list
// - if not: add input word at beginning of list
public static void play(ListIterator<String> iter, String word) {
}
}

Step by step
Solved in 3 steps with 2 images

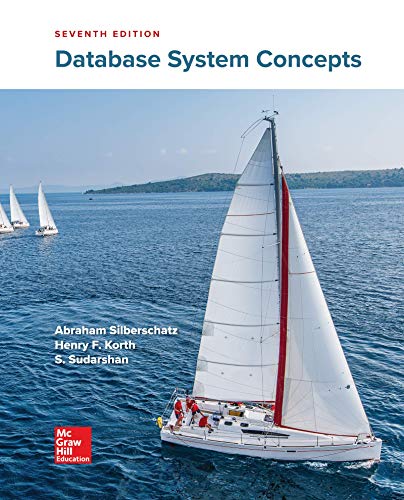
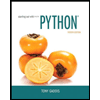
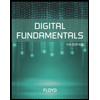
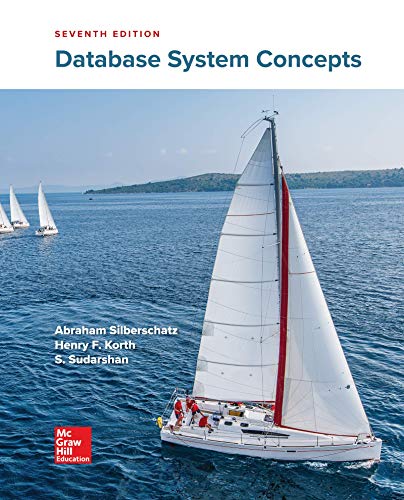
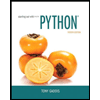
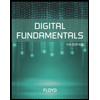
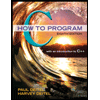
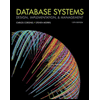
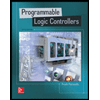