This is the error message I get: Exception in thread "main" java.lang.IndexOutOfBoundsException: Index 7 out of bounds for length 7 at java.base/jdk.internal.util.Preconditions.outOfBounds(Preconditions.java:64) at java.base/jdk.internal.util.Preconditions.outOfBoundsCheckIndex(Preconditions.java:70) at java.base/jdk.internal.util.Preconditions.checkIndex(Preconditions.java:266) at java.base/java.util.Objects.checkIndex(Objects.java:359) at java.base/java.util.ArrayList.get(ArrayList.java:427) at radixsort.RadixSort.radixSort(RadixSort.java:82) at radixsort.RadixSort.main(RadixSort.java:26) Code: package radixsort; public class RadixSort { public static void main(String[] args) { // Create a new list int[]mylist = new int[100]; // Loop that iterates for the entire list of elements for(int i=0; i[]newbucket=new java.util.ArrayList[10]; // Loop that iterates for the list of elements for(int i=0; i(); } // Loop that iterates for the number of digits for (int position = 0; position<=digit_count; position++) { // Loop that iterates to clear the bucket for(int i=0; i
This is the error message I get:
Exception in thread "main" java.lang.IndexOutOfBoundsException: Index 7 out of bounds for length 7
at java.base/jdk.internal.util.Preconditions.outOfBounds(Preconditions.java:64)
at java.base/jdk.internal.util.Preconditions.outOfBoundsCheckIndex(Preconditions.java:70)
at java.base/jdk.internal.util.Preconditions.checkIndex(Preconditions.java:266)
at java.base/java.util.Objects.checkIndex(Objects.java:359)
at java.base/java.util.ArrayList.get(ArrayList.java:427)
at radixsort.RadixSort.radixSort(RadixSort.java:82)
at radixsort.RadixSort.main(RadixSort.java:26)
Code:
package radixsort;
public class RadixSort {
public static void main(String[] args) {
// Create a new list
int[]mylist = new int[100];
// Loop that iterates for the entire list of elements
for(int i=0; i<mylist.length; i++)
// Create a random numbers
mylist[i]= (int)(Math.random()*1000);
// Perform Sort
radixSort(mylist,3);
// Loop that interates over the entire elements
for(int i=0; i<mylist.length; i++)
// Display the elements
System.out.print(mylist[i]+"");
}
// Method definition to sort the elements
public static void radixSort(int[]list, int digit_count)
{
// Create the new bucket
java.util.ArrayList<Integer>[]newbucket=new java.util.ArrayList[10];
// Loop that iterates for the list of elements
for(int i=0; i<newbucket.length; i++)
{
// Add elements into the bucket
newbucket[i]= new java.util.ArrayList<Integer>();
}
// Loop that iterates for the number of digits
for (int position = 0; position<=digit_count; position++)
{
// Loop that iterates to clear the bucket
for(int i=0; i<newbucket.length; i++)
{
// Clear the bucket
newbucket[i].clear();
}
// Loop that iterates to distribute the elements into the bucket
for(int i=0; i<list.length; i++)
{
// Position the bucket
int key = getKey(list[i],position);
// Add the elements into the list
newbucket[key].add(list[i]);
}
// Elements are moved back to the bucket
int k =0;
// Loop that iterates the value of the elements
for(int i=0; i<newbucket.length; i++)
{
// Loop that iterates the value of the elements
for(int j=0; j<newbucket[i].size(); j++)
// Add the elements into the bucket
list[k++]=newbucket[i].get(j);
}
}
}
// Method to return the key elements
public static int getKey(int number, int position)
{
// Declare the required variables
int result=1;
// Loop that iterates to add the desired position
for(int i=0; i<position; i++)
// Calculate the result value
result*=10;
// Return the result
return(number/result)%10;
}
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

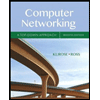
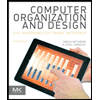
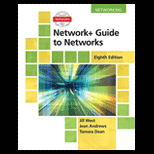
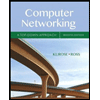
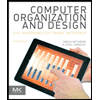
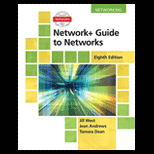
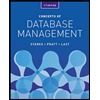
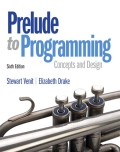
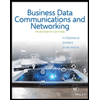