I'm trying to output a Mad Lib story, but I keep getting an "Exception in thread" error. How do I fix my code?
I'm trying to output a Mad Lib story, but I keep getting an "Exception in thread" error. How do I fix my code?
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
I'm trying to output a Mad Lib story, but I keep getting an "Exception in thread" error. How do I fix my code?
![### Java Program to Take User Input
The following Java code snippet demonstrates how to use the `Scanner` class from the `java.util` package to take user inputs.
```java
import java.util.Scanner;
public class LabProgram {
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
String firstName;
String genericLocation;
int wholeNumber;
String pluralNoun;
firstName = scnr.next();
genericLocation = scnr.next();
wholeNumber = scnr.nextInt();
pluralNoun = scnr.next();
}
}
```
**Explanation:**
1. **Imports:**
```java
import java.util.Scanner;
```
- This line imports the `Scanner` class which is necessary for taking input from the user.
2. **Class Declaration:**
```java
public class LabProgram {
```
- This declares a public class named `LabProgram`.
3. **Main Method:**
```java
public static void main(String[] args) {
```
- This is the entry point of the Java program. The `main` method is where the program execution begins.
4. **Creating `Scanner` Object:**
```java
Scanner scnr = new Scanner(System.in);
```
- Creates a `Scanner` object named `scnr` which takes input from standard input (keyboard).
5. **Variable Declarations:**
```java
String firstName;
String genericLocation;
int wholeNumber;
String pluralNoun;
```
- Declares four variables: `firstName` and `genericLocation` of type `String`, `wholeNumber` of type `int`, and `pluralNoun` of type `String`.
6. **Taking Inputs:**
```java
firstName = scnr.next();
genericLocation = scnr.next();
wholeNumber = scnr.nextInt();
pluralNoun = scnr.next();
```
- This block of code takes user input for each variable:
- `firstName` uses `scnr.next()` to read a `String`.
- `genericLocation` uses `scnr.next()` to read a `String`.
- `wholeNumber` uses `scnr.nextInt()` to read an `int`.
- `pluralNoun` uses `scnr.next()` to](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fbf698295-04c9-431c-9153-2f08912ee134%2F57781f80-931a-4231-875c-d2bb91ff16e6%2F22jxzi_processed.jpeg&w=3840&q=75)
Transcribed Image Text:### Java Program to Take User Input
The following Java code snippet demonstrates how to use the `Scanner` class from the `java.util` package to take user inputs.
```java
import java.util.Scanner;
public class LabProgram {
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
String firstName;
String genericLocation;
int wholeNumber;
String pluralNoun;
firstName = scnr.next();
genericLocation = scnr.next();
wholeNumber = scnr.nextInt();
pluralNoun = scnr.next();
}
}
```
**Explanation:**
1. **Imports:**
```java
import java.util.Scanner;
```
- This line imports the `Scanner` class which is necessary for taking input from the user.
2. **Class Declaration:**
```java
public class LabProgram {
```
- This declares a public class named `LabProgram`.
3. **Main Method:**
```java
public static void main(String[] args) {
```
- This is the entry point of the Java program. The `main` method is where the program execution begins.
4. **Creating `Scanner` Object:**
```java
Scanner scnr = new Scanner(System.in);
```
- Creates a `Scanner` object named `scnr` which takes input from standard input (keyboard).
5. **Variable Declarations:**
```java
String firstName;
String genericLocation;
int wholeNumber;
String pluralNoun;
```
- Declares four variables: `firstName` and `genericLocation` of type `String`, `wholeNumber` of type `int`, and `pluralNoun` of type `String`.
6. **Taking Inputs:**
```java
firstName = scnr.next();
genericLocation = scnr.next();
wholeNumber = scnr.nextInt();
pluralNoun = scnr.next();
```
- This block of code takes user input for each variable:
- `firstName` uses `scnr.next()` to read a `String`.
- `genericLocation` uses `scnr.next()` to read a `String`.
- `wholeNumber` uses `scnr.nextInt()` to read an `int`.
- `pluralNoun` uses `scnr.next()` to

Transcribed Image Text:### Program Errors and Debugging
#### Exception in Java
When running a Java program, an exception error may occur if the run-time environment detects an issue. An example of such an error is shown below:
```
Exception in thread "main" java.util.NoSuchElementException
at java.base/java.util.Scanner.throwFor(Scanner.java:937)
at java.base/java.util.Scanner.next(Scanner.java:1478)
at LabProgram.main(LabProgram.java:15)
```
This error message indicates that a `NoSuchElementException` has been thrown. This typically occurs when one tries to access an element that does not exist. In this case, it’s happening within the `Scanner.next()` method. The error trace pinpoints the exact locations in the code where problems occurred:
1. **java.util.Scanner.throwFor** - Located at line 937 in `Scanner.java`.
2. **java.util.Scanner.next** - Located at line 1478 in `Scanner.java`.
3. **LabProgram.main** - Located at line 15 in `LabProgram.java`.
#### Coding Trail of Your Work
Below the error message, there appears to be a coding trail tracker:
```
6/8 W-----------4-----------3----------- min:68
```
This coding trail shows the progress of your work and might represent various checkpoints, changes, and possibly the time spent coding.
1. **6/8**: Possibly represents the number of completed tasks or levels out of a total of 8.
2. **W**: The letter might indicate a specific milestone or a point where significant work was done.
3. **4 and 3**: These numbers could represent different stages or steps in the coding process.
This tracker can help you visualize and keep track of your coding progress and milestones.
#### Icons at the Bottom
At the bottom of the screen, various application icons are displayed, which include:
- A web browser icon.
- File explorer.
- Email application.
- Cloud storage service.
- Office tools.
These icons suggest that you might have multiple tools at your disposal to assist with coding, documentation, and communication.
### Summary
Understanding and interpreting error messages are crucial for debugging. The `NoSuchElementException` in this example provides precise locations where the error occurs. Additionally, using coding trail features and tools can assist in maintaining an organized approach to coding and error resolution.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
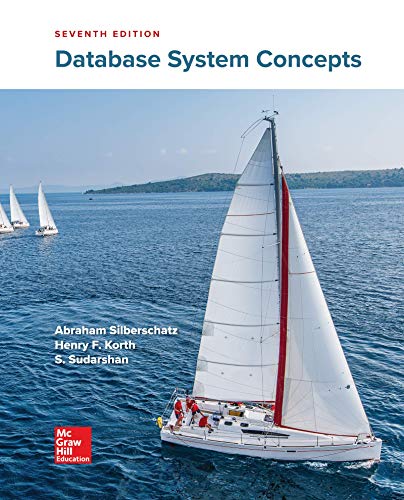
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
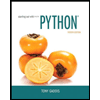
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
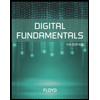
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
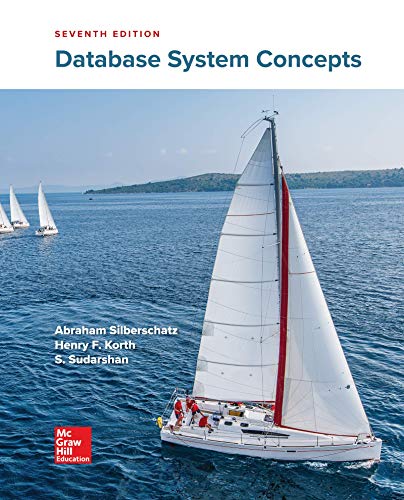
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
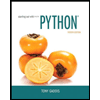
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
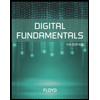
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
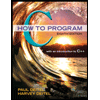
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
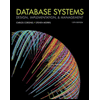
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
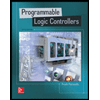
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education