{ public static void main(String[] args) throws IOException { // Create a new LinkedList object for holding the ValueData object: LinkedList linkedList =
When I attempt to run this program, I am getting an ArrayIndexOutOfBounds Exception on line 21 of the given Main. What is causing this to occur and what can be done to fix it?
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
import java.util.LinkedList;
public class Main{
public static void main(String[] args) throws IOException {
// Create a new LinkedList object for holding the ValueData object:
LinkedList<ValueData> linkedList = new LinkedList<>();
// Create a new ObjectStack object
ObjectStack objectStack = new ObjectStack();
// Read a text file containing lines (records) of ValueData.
// This is in order to fill the LinkedList with ValueData from the file.
BufferedReader reader = new BufferedReader(new FileReader("values.txt"));
String line = reader.readLine();
while (line != null) {
String[] parts = line.split(",");
String name = parts[0].trim();
int value = Integer.parseInt(parts[1].trim());
ValueData data = new ValueData(name, value);
linkedList.add(data);
objectStack.push(data);
line = reader.readLine();
}
reader.close();
// Use the linkedList and objectStack objects as needed.
}
}
___________________________________________________________________________________________
ObjectStack.java:
import java.util.EmptyStackException;
public class ObjectStack extends LinkedList implements Stack {
private int size;
// Creates a new instance of ObjectStack:
public ObjectStack() {
super();
}
@Override
public void push(Object item) {
// Method body for push()
}
@Override
public Object pop() throws EmptyStackException {
// Method body for pop()
return null;
}
@Override
public Object top() throws EmptyStackException {
// Method body for top()
return null;
}
@Override
public boolean isEmpty() {
// Method body for isEmpty()
return false;
}
@Override
public int size() {
// Method body for size()
return 0;
}
}
__________________________________
Stack.java (interface):
import java.util.EmptyStackException;
public interface Stack {
public int size();
public boolean isEmpty();
public Object top () throws EmptyStackException;
public Object pop () throws EmptyStackException;
public void push (Object item);
}
___________________________________
LinkedListNode.java:
public class LinkedListNode
{
private Object data;
private LinkedListNode next;
// Constructor:
public LinkedListNode(Object data) {
this.data = data;
this.next = null;
}
// Getter and setter:
public LinkedListNode getNext() {
return next;
}
public void setNext(LinkedListNode next) {
this.next = next;
}
public Object getData() {
return data;
}
public void setData(Object data) {
this.data = data;
}
public Object getItem() {
return null;
}
}
__________________________________________
LinkedList.java:
public class LinkedList{
private Node head; // head node of the list
/* Default constructor that creates an empty list */
public LinkedList() {
head = null;
}
//inserts new node at beginning of list
public void insert(Node v){
v.setNext(head);
head = v;
}
//searches elements of linked list for a match
public String search (String target) {
Node temp = head;
while(temp != null){
if(target.equals(temp.getElement())){
return "Found the target";
}
temp = temp.getNext();
}
return "Target not found";
}
//remove the first node of the array
public void removeFirstNode( ) {
if(head != null){
head = head.getNext();
}
}
//removes node with element matching the string target
public void removeNode( String target) {
Node prev = null;
Node curr = head;
while(curr != null){
if(target.equals(curr.getElement())){
break;
}
prev = curr;
curr = curr.getNext();
}
if(curr == null)
return;
if(prev == null){
removeFirstNode();
return;
}
prev.setNext(curr.getNext());
}
//loop from first to last element and prints them all
public void printList(){
Node temp = head;
while(temp != null){
System.out.print(temp.getElement() + " ");
temp = temp.getNext();
}
System.out.println();
}
}//end of class
______________________________________
Node.java:
public class Node {
private String element; // we assume elements are character strings
private Node next;
/* Creates a node with the given element and next node. */
public Node(String s, Node n) {
element = s;
next = n;
}
/* Returns the element of this node. */
public String getElement() {
return element;
}
/* Returns the next node of this node. */
public Node getNext() {
return next;
}
// Modifier methods:
/* Sets the element of this node. */
public void setElement(String newElem) {
element = newElem;
}
/* Sets the next node of this node. */
public void setNext(Node newNext) {
next = newNext;
}
}
__________________________________________
ValueData.java:
public class ValueData {
private String variable;
private double value;
// Define the constructor:
public ValueData(String variable, double value) {
this.variable = variable;
this.value = value;
}
// Define the getter and setter:
public String getVariable() {
return variable;
}
public void setVariable(String variable) {
this.variable = variable;
}
public double getValue() {
return value;
}
public void setValue(double value) {
this.value = value;
}
// Define the toString method:
@Override
public String toString() {
return "Variable: " + variable + ", Value: " + value;
}
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

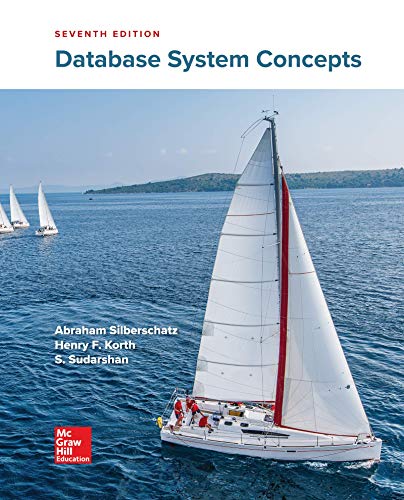
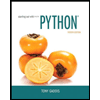
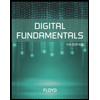
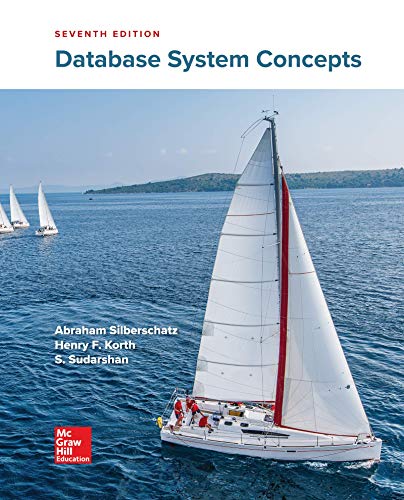
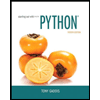
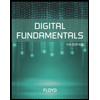
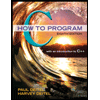
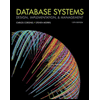
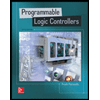