Getting this error when trying to run this program - java.lang.ArrayIndexOutOfBoundsException: Index 1 out of bounds for length 1 here is the program below. import java.util.ArrayList; import java.util.Scanner; import java.io.File; import java.io.FileNotFoundException; public class Largest_Country { public static ArrayList readCountry(String filename) throws FileNotFoundException { // Create an arraylist of the country class ArrayList countries = new ArrayList(); // Create a scanner object to open the input file Scanner scan = new Scanner(new File(filename)); // Initialize a string variable to read the file String line = ""; while(scan.hasNextLine()) { // Read the file line by line line = scan.nextLine(); // Split the line at spaces, and assign the values to // a string array String[] details = line.split(" "); // Create object of the class Country, and pass the values // to the object Country country_info = new Country(details[0], Long.parseLong(details[1]), Long.parseLong(details[2])); // Add the object to the arraylist countries.add(country_info); } // Return the arraylist return countries; } // Define the countryWithLargestArea() method public static Country countryWithLargestArea(ArrayList countries) { // Create an object of the class, and assign the // first value from the arraylist Country largest_area_country = countries.get(0); for(int x = 0; x < countries.size(); x++) { // Check if the value of area at index x is greater than // the value at largest_area_country if(countries.get(x).getCountryArea() > largest_area_country.getCountryArea()) { // Assign the values to largest_area_country largest_area_country = countries.get(x); } } // Return the country details return largest_area_country; } // Define the countryWithLargestPopulation() method public static Country countryWithLargestPopulation(ArrayList countries) { // Create an object of the class, and assign the // first value from the arraylist Country largest_population_country = countries.get(0); for(int x = 0; x < countries.size(); x++) { // Check if the value of area at index x is greater than // the value at largest_population_country if(countries.get(x).getCountryPopulation() > largest_population_country.getCountryPopulation()) { // Assign the values to largest_population_country largest_population_country = countries.get(x); } } // Return the country details return largest_population_country; } // Define the countryWithLargestPopulationDensity() method public static Country countryWithLargestPopulationDensity(ArrayList countries) { // Create an object of the class, and assign the // first value from the arraylist Country largest_population_density_country = countries.get(0); for(int x = 0; x < countries.size(); x++) { // Check if the value of area at index x is greater than // the value at largest_population_density_country if(countries.get(x).getPopulationDensity() > largest_population_density_country.getPopulationDensity()) { // Assign the values to largest_population_density_country largest_population_density_country = countries.get(x); } } // Return the country details return largest_population_density_country; } // Define the main() method public static void main(String[] args) { try { // Create an arraylist, and call the readCountry() method ArrayList countries = readCountry("countrydata.txt"); // Call the countryWithLargestArea(), and display the // country having largest area System.out.println("The country with the largest area:\n" + countryWithLargestArea(countries).printCountry()); // Call the countryWithLargestArea(), and display the // country having largest population System.out.println("\nThe country with the largest population:\n" + countryWithLargestPopulation(countries).printCountry()); // Call the countryWithLargestPopulationDensity(), and display the // country having largest population density System.out.println("\nThe country with the largest population density:\n" + countryWithLargestPopulationDensity(countries).printCountry()); } catch(FileNotFoundException e) { // Display file not found exception System.out.println("File not found!!"); } } }
Getting this error when trying to run this program - java.lang.ArrayIndexOutOfBoundsException: Index 1 out of bounds for length 1
here is the program below.
import java.util.ArrayList;
import java.util.Scanner;
import java.io.File;
import java.io.FileNotFoundException;
public class Largest_Country
{
public static ArrayList<Country> readCountry(String filename) throws FileNotFoundException
{
// Create an arraylist of the country class
ArrayList<Country> countries = new ArrayList<Country>();
// Create a scanner object to open the input file
Scanner scan = new Scanner(new File(filename));
// Initialize a string variable to read the file
String line = "";
while(scan.hasNextLine())
{
// Read the file line by line
line = scan.nextLine();
// Split the line at spaces, and assign the values to
// a string array
String[] details = line.split(" ");
// Create object of the class Country, and pass the values
// to the object
Country country_info = new Country(details[0], Long.parseLong(details[1]), Long.parseLong(details[2]));
// Add the object to the arraylist
countries.add(country_info);
}
// Return the arraylist
return countries;
}
// Define the countryWithLargestArea() method
public static Country countryWithLargestArea(ArrayList<Country> countries)
{
// Create an object of the class, and assign the
// first value from the arraylist
Country largest_area_country = countries.get(0);
for(int x = 0; x < countries.size(); x++)
{
// Check if the value of area at index x is greater than
// the value at largest_area_country
if(countries.get(x).getCountryArea() > largest_area_country.getCountryArea())
{
// Assign the values to largest_area_country
largest_area_country = countries.get(x);
}
}
// Return the country details
return largest_area_country;
}
// Define the countryWithLargestPopulation() method
public static Country countryWithLargestPopulation(ArrayList<Country> countries)
{
// Create an object of the class, and assign the
// first value from the arraylist
Country largest_population_country = countries.get(0);
for(int x = 0; x < countries.size(); x++)
{
// Check if the value of area at index x is greater than
// the value at largest_population_country
if(countries.get(x).getCountryPopulation() > largest_population_country.getCountryPopulation())
{
// Assign the values to largest_population_country
largest_population_country = countries.get(x);
}
}
// Return the country details
return largest_population_country;
}
// Define the countryWithLargestPopulationDensity() method
public static Country countryWithLargestPopulationDensity(ArrayList<Country> countries)
{
// Create an object of the class, and assign the
// first value from the arraylist
Country largest_population_density_country = countries.get(0);
for(int x = 0; x < countries.size(); x++)
{
// Check if the value of area at index x is greater than
// the value at largest_population_density_country
if(countries.get(x).getPopulationDensity() > largest_population_density_country.getPopulationDensity())
{
// Assign the values to largest_population_density_country
largest_population_density_country = countries.get(x);
}
}
// Return the country details
return largest_population_density_country;
}
// Define the main() method
public static void main(String[] args)
{
try
{
// Create an arraylist, and call the readCountry() method
ArrayList<Country> countries = readCountry("countrydata.txt");
// Call the countryWithLargestArea(), and display the
// country having largest area
System.out.println("The country with the largest area:\n" + countryWithLargestArea(countries).printCountry());
// Call the countryWithLargestArea(), and display the
// country having largest population
System.out.println("\nThe country with the largest population:\n" + countryWithLargestPopulation(countries).printCountry());
// Call the countryWithLargestPopulationDensity(), and display the
// country having largest population density
System.out.println("\nThe country with the largest population density:\n" + countryWithLargestPopulationDensity(countries).printCountry());
}
catch(FileNotFoundException e)
{
// Display file not found exception
System.out.println("File not found!!");
}
}
}


Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 7 images

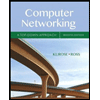
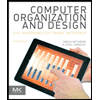
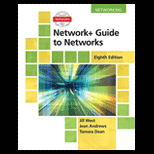
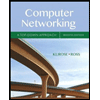
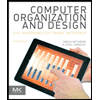
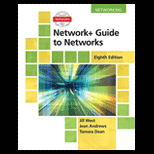
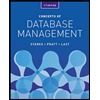
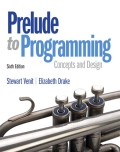
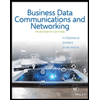