In java demonstrate the producer/consumer problem by setting up two threads that use a shared buffer. Use Threads and/or Runnable objects. Do not use synchronized, wait, notifyAll or a boolean to control access to the shared buffer. use Rect.java
In java demonstrate the producer/consumer problem by setting up two threads that use a shared buffer. Use Threads and/or Runnable objects. Do not use synchronized, wait, notifyAll or a boolean to control access to the shared buffer. use Rect.java
Create classes as follows:
RectangleBuffer – class to represent one Rect object with a get and set method
Producer class – produces 10 Rect objects and writes them successively to a RectangleBuffer, which is a data member of the class
Consumer class – consumes (reads) 10 Person objects successively from a RectangleBuffer, which is a data member of the class
ProducerConsumerTest – sets up a producer and consumer thread to execute using a shared RectangleBuffer
Rect.java
public class Rect implements Comparable<Rect>
{
private double length;
private double width;
public Rect()
{
length = 0.0;
width = 0.0;
}
public Rect(double l, double w)
{
length = l;
width = w;
}
public Rect(Rect r) {
this.length = r.length;
this.width = r.width;
}
public double getLength()
{
return length;
}
public double getWidth()
{
return width;
}
public void setLength(double l)
{
length = l;
}
public void setWidth(double w)
{
width = w;
}
public double calcArea()
{
return length * width;
}
public boolean equals(Rect r)
{
boolean temp = false;
if ((this.length == r.getLength()) && (this.width == r.getWidth()))
temp = true;
return temp;
}
public int compareTo(Rect r) {
if (this.calcArea() < r.calcArea())
return -1;
else if (this.calcArea() > r.calcArea())
return 1;
else
return 0;
}
public String toString()
{
return "[Length = " + length + " Width = " + width+ "]";
}
}

Step by step
Solved in 2 steps

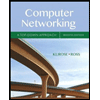
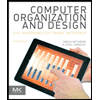
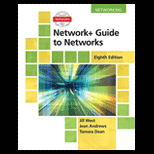
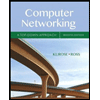
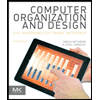
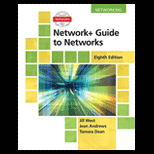
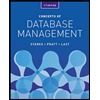
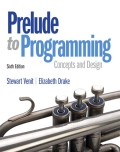
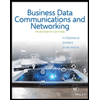