This is a C program. I want you to convert it to a C++. Also provide a screenshot that it is working here is the program to be converted: #include #include #include #define Pi 3.1416 int main() { float R,Result; char Resp,temp; clrscr(); printf("Enter Radius of the Circle: "); scanf("%f",&R); printf("Computing for any of the following:\n"); printf("\t[D] Diameter\n\t[C] Circumference\n\t[A] Area\n\nEnter choice: "); scanf("%c",&temp); //to catch the null value being returned by scanf() scanf("%c",&Resp); Resp=toupper(Resp); if (Resp == 'D') { Result=R*2; printf("The Diameter of the Circle is %0.2f\n",Result); } else if (Resp == 'C') { Result=2*Pi*R; printf("The Circumference of the Circle is %0.2f\n",Result); } else if (Resp == 'A') { Result=Pi*R*R; printf("The Area of the Circle is %0.2f\n",Result); } else printf("Invalid Response!!!"); getch(); return(0); }
This is a C program. I want you to convert it to a C++. Also provide a screenshot that it is working
here is the program to be converted:
#include <stdio.h>
#include <conio.h>
#include <ctype.h>
#define Pi 3.1416
int main()
{
float R,Result;
char Resp,temp;
clrscr();
printf("Enter Radius of the Circle: ");
scanf("%f",&R);
printf("Computing for any of the following:\n");
printf("\t[D] Diameter\n\t[C] Circumference\n\t[A] Area\n\nEnter choice: ");
scanf("%c",&temp); //to catch the null value being returned by scanf()
scanf("%c",&Resp);
Resp=toupper(Resp);
if (Resp == 'D')
{
Result=R*2;
printf("The Diameter of the Circle is %0.2f\n",Result);
}
else if (Resp == 'C')
{
Result=2*Pi*R;
printf("The Circumference of the Circle is %0.2f\n",Result);
}
else if (Resp == 'A')
{
Result=Pi*R*R;
printf("The Area of the Circle is %0.2f\n",Result);
}
else
printf("Invalid Response!!!");
getch();
return(0);
}

Step by step
Solved in 3 steps with 1 images

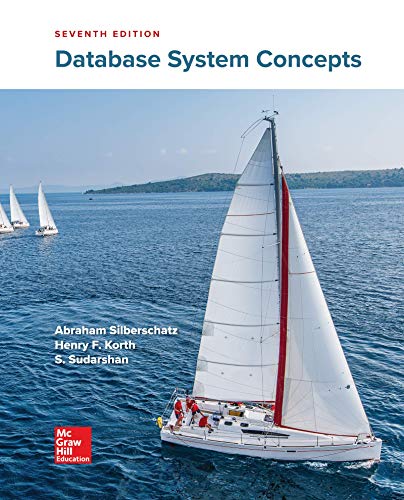
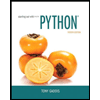
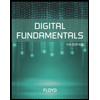
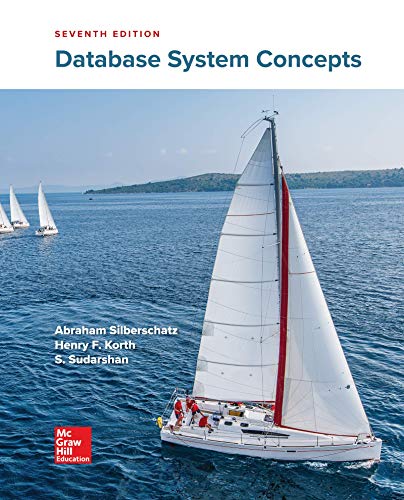
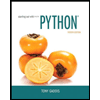
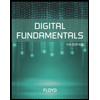
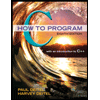
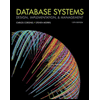
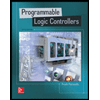