the code that I have, I need to change the endpont /specialOperation to asynchronously (with your utility module) get the grade result and rectangle result like this( promises required):
the code that I have, I need to change the endpont /specialOperation to asynchronously (with your utility module) get the grade result and rectangle result like this( promises required):
{"gradeStats":{"average":86.25,"minimum":73,"maximum":97},"rectangle":{"area":8,"perimeter":12}}
1)

Here's how you can modify the specialOperation
function to use promises and make the necessary calls to gradeStats
and rectangle
asynchronously:
async function specialOperation(gradeString, length, width) {
const promise1 = new Promise((resolve, reject) => {
if (!gradeString) {
reject(new Error("Grades parameter is missing."));
return;
}
const grade = String(gradeString).split(",").map(Number);
calculator.gradeStats(grade)
.then((result) => resolve(result))
.catch((error) => reject(error));
});
const promise2 = new Promise((resolve, reject) => {
calculator.rectangle(length, width)
.then((result) => resolve(result))
.catch((error) => reject(error));
});
const [gradeStatsResult, rectangleResult] = await Promise.all([promise1, promise2]);
const result = { gradeStats: gradeStatsResult, rectangle: rectangleResult };
return result;
}
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

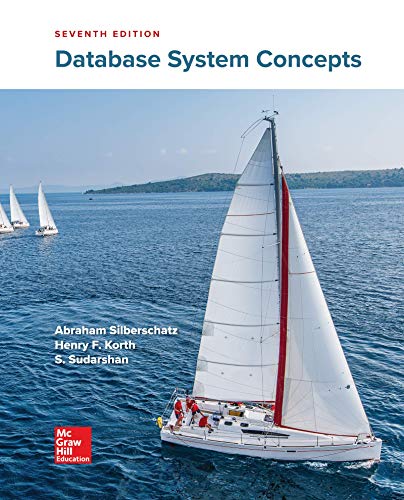
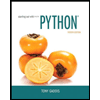
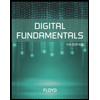
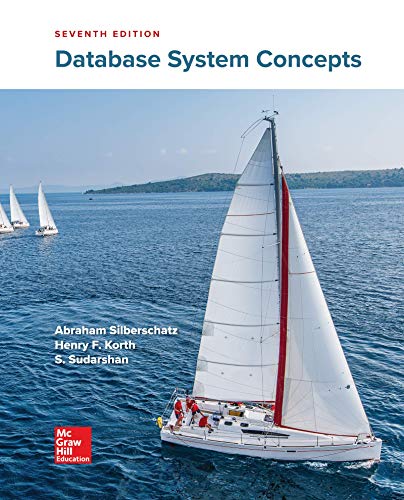
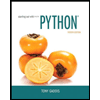
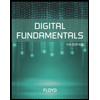
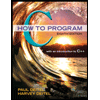
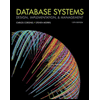
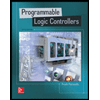