Requirement: In this assignment, you are going to handle exceptional cases in the bank account class for foreign currencies. In the attached files, source codes for ForeignCurrencyAccount and Account classes are given. There is also Test3.java and Transaction.java that run operations on the bank accounts. Please complete the implementation of ForeignCurrencyAccount and Account classes, so that following exceptional cases are handled by them. Do not add new attributes or methods to ForeignCurrencyAccount and Account classes. Just add code to throw exceptions or handle exceptions. 1. Whenever a transaction amount given to "withdraw" method is greater than account's balance, an exception of class InsufficientFundsException should be thrown. 2. Whenever InsufficientFundsException is caught within buyForeignCurrency or sellForeignCurrency methods, an Exception should be thrown with description "Foreign currency buy/sell transaction failed because of insufficient funds". 3. Whenever setCurrencyCode method is called with a different currency code than the account's currency code and account has non-zero balance, an Exception should be thrown with description "Currency code cannot be changed for an account that has balance". 4. Whenever dailyExchangeRate is zero and a method that uses dailyExchangeRate (for example, convertToCAD method) is called, an Exception should be thrown with description "Daily exchange rate not set". Expected output: Account: 1122 my checking account Balance: 3200.0 CAD Account: 3984 my USD account Balance: 4000.0 USD Account: 4597 my EUR account Balance: 12000.0 EUR Account: 5921 my Australian Dollar account Balance: 1000.0 AUD assignment4.InsufficientFundsException at assignment4.Account.withdraw(Account.java:51) at assignment4.Test3.main(Test3.java:46) java.lang.Exception: Currency code cannot be changed for an account that has balance. at assignment4.ForeignCurrencyAccount.setCurrencyCode(ForeignCurrencyAccount.java:33) at assignment4.Test3.main(Test3.java:58) java.lang.Exception: Daily exchange rate not set. at assignment4.ForeignCurrencyAccount.convertToCAD(ForeignCurrencyAccount.java:25) at assignment4.ForeignCurrencyAccount.getBalanceInCAD(ForeignCurrencyAccount.java:44) at assignment4.Test3.main(Test3.java:66) Sum of all my accounts on day 1: 26360.0 CAD Changing exchange rates for day 2 java.lang.Exception: Daily exchange rate not set. at assignment4.ForeignCurrencyAccount.convertToCAD(ForeignCurrencyAccount.java:25) at assignment4.ForeignCurrencyAccount.getBalanceInCAD(ForeignCurrencyAccount.java:44) at assignment4.Test3.main(Test3.java:84) Sum of all my accounts on day 2: 25480.0 CAD Buying 100 USD Selling 50 EUR Buying 100000 JPY java.lang.Exception: Foreign currency buy transaction failed because of insufficient funds at assignment4.Transaction.buyForeignCurrency(Transaction.java:20) at assignment4.Test3.main(Test3.java:108) Account: 1122 my checking account Balance: 3140.0 CAD Account: 3984 my USD account Balance: 4100.0 USD Account: 4597 my EUR account Balance: 11950.0 EUR java.lang.Exception: Daily exchange rate not set. at assignment4.ForeignCurrencyAccount.convertToCAD(ForeignCurrencyAccount.java:25) at assignment4.ForeignCurrencyAccount.getBalanceInCAD(ForeignCurrencyAccount.java:44) at assignment4.Test3.main(Test3.java:116) Account: 5921 my Australian Dollar account Balance: 1000.0 AUD Account: 6568 my Japanese Yen account Balance: 0.0 JPY Sum of all my accounts after buying / selling foreign currency: 25480.0 CAD
Requirement:
In this assignment, you are going to handle exceptional cases in the bank account class for foreign currencies.
In the attached files, source codes for ForeignCurrencyAccount and Account classes are given. There is also Test3.java and Transaction.java that run operations on the bank accounts.
Please complete the implementation of ForeignCurrencyAccount and Account classes, so that following exceptional cases are handled by them.
Do not add new attributes or methods to ForeignCurrencyAccount and Account classes. Just add code to throw exceptions or handle exceptions.
1. Whenever a transaction amount given to "withdraw" method is greater than account's balance, an exception of class InsufficientFundsException should be thrown.
2. Whenever InsufficientFundsException is caught within buyForeignCurrency or sellForeignCurrency methods, an Exception should be thrown with description "Foreign currency buy/sell transaction failed because of insufficient funds".
3. Whenever setCurrencyCode method is called with a different currency code than the account's currency code and account has non-zero balance, an Exception should be thrown with description "Currency code cannot be changed for an account that has balance".
4. Whenever dailyExchangeRate is zero and a method that uses dailyExchangeRate (for example, convertToCAD method) is called, an Exception should be thrown with description "Daily exchange rate not set".
Expected output:

Step by step
Solved in 2 steps

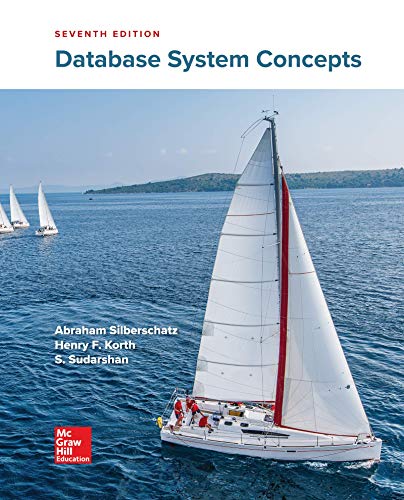
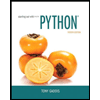
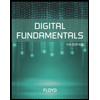
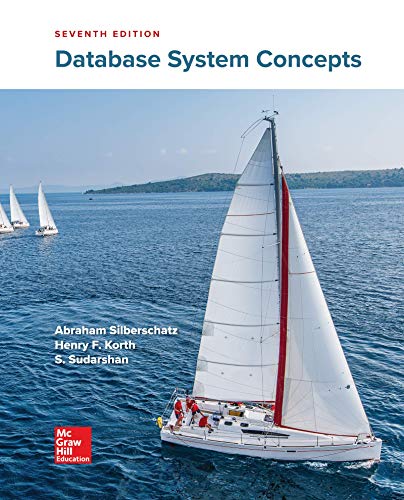
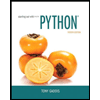
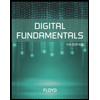
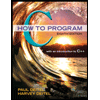
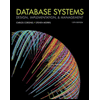
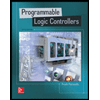