Task 2: Comparable Interface and Record (10 Points) 1. You are tasked with creating a Java record of Dog (UML is shown below). The dog record should include the dog's name, breed, age, and weight. You are required to implement the Comparable interface for the Dog record so that you can sort the records based on the dogs' ages. Create a Java record named Dog.java. name: String breed: String age: int weight: double + toString(): String <> Dog + compareTo(otherDog: Dog): int <> Comparable 2. In the Dog record, establish a main method and proceed to generate an array named dogList containing three Dog objects, each with the following attributes: Dog1: name: "Buddy", breed: "Labrador Retriever", age: 5, weight: 25.5 Dog2: name: "Max", breed: "Golden Retriever", age: 3, weight: 30 Dog3: name: "Charlie", breed: "German Shepherd", age: 2, weight: 22 3. Print the dogs in dogList before sorting the dogList by age. (Please check the example output for the format). • 4. Sort the dogList using Arrays.sort(). 5. Print the dogs in dogList after sorting. Note: 1. Implement the Comparable interface for the Dog record so that dogs can be compared based on their ages. The compareTo() method should compare dogs based on their ages in ascending order. 2. Use Arrays.toString() to print the array of Dogs. 3. Use Arrays.sort() to sort the dogList array. 4. Override the toString() method in the Dog record to print the three dogs in the example format: Example: Before sorting: [Dog: age=5, Dog: age=3, Dog: age=2] After sorting: [Dog: age=2, Dog: age=3, Dog: age=5]
Task 2: Comparable Interface and Record (10 Points) 1. You are tasked with creating a Java record of Dog (UML is shown below). The dog record should include the dog's name, breed, age, and weight. You are required to implement the Comparable interface for the Dog record so that you can sort the records based on the dogs' ages. Create a Java record named Dog.java. name: String breed: String age: int weight: double + toString(): String <> Dog + compareTo(otherDog: Dog): int <> Comparable 2. In the Dog record, establish a main method and proceed to generate an array named dogList containing three Dog objects, each with the following attributes: Dog1: name: "Buddy", breed: "Labrador Retriever", age: 5, weight: 25.5 Dog2: name: "Max", breed: "Golden Retriever", age: 3, weight: 30 Dog3: name: "Charlie", breed: "German Shepherd", age: 2, weight: 22 3. Print the dogs in dogList before sorting the dogList by age. (Please check the example output for the format). • 4. Sort the dogList using Arrays.sort(). 5. Print the dogs in dogList after sorting. Note: 1. Implement the Comparable interface for the Dog record so that dogs can be compared based on their ages. The compareTo() method should compare dogs based on their ages in ascending order. 2. Use Arrays.toString() to print the array of Dogs. 3. Use Arrays.sort() to sort the dogList array. 4. Override the toString() method in the Dog record to print the three dogs in the example format: Example: Before sorting: [Dog: age=5, Dog: age=3, Dog: age=2] After sorting: [Dog: age=2, Dog: age=3, Dog: age=5]
Chapter10: Introduction To Inheritance
Section: Chapter Questions
Problem 14RQ
Related questions
Question

Transcribed Image Text:Task 2: Comparable Interface and Record (10 Points)
1. You are tasked with creating a Java record of Dog (UML is shown below). The dog record
should include the dog's name, breed, age, and weight. You are required to implement the
Comparable interface for the Dog record so that you can sort the records based on the dogs'
ages. Create a Java record named Dog.java.
name: String
breed: String
age: int
weight: double
+ toString(): String
<<Record>>
Dog
+ compareTo(otherDog: Dog): int
<<interface>>
Comparable<Dog>
2. In the Dog record, establish a main method and proceed to generate an array named
dogList containing three Dog objects, each with the following attributes:
Dog1: name: "Buddy", breed: "Labrador Retriever", age: 5, weight: 25.5
Dog2: name: "Max", breed: "Golden Retriever", age: 3, weight: 30
Dog3: name: "Charlie", breed: "German Shepherd", age: 2, weight: 22
3. Print the dogs in dogList before sorting the dogList by age. (Please check the example
output for the format).
•
4. Sort the dogList using Arrays.sort().
5. Print the dogs in dogList after sorting.
Note:
![1. Implement the Comparable interface for the Dog record so that dogs can be
compared based on their ages. The compareTo() method should compare dogs
based on their ages in ascending order.
2. Use Arrays.toString() to print the array of Dogs.
3. Use Arrays.sort() to sort the dogList array.
4. Override the toString() method in the Dog record to print the three dogs in the
example format:
Example:
Before sorting:
[Dog: age=5, Dog: age=3, Dog: age=2]
After sorting:
[Dog: age=2, Dog: age=3, Dog: age=5]](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F1f1cff89-d0c3-401a-ba77-d9b5f81fcda4%2F16b6aeb8-e072-4276-92a6-3716001114e1%2F7sbdi39_processed.png&w=3840&q=75)
Transcribed Image Text:1. Implement the Comparable interface for the Dog record so that dogs can be
compared based on their ages. The compareTo() method should compare dogs
based on their ages in ascending order.
2. Use Arrays.toString() to print the array of Dogs.
3. Use Arrays.sort() to sort the dogList array.
4. Override the toString() method in the Dog record to print the three dogs in the
example format:
Example:
Before sorting:
[Dog: age=5, Dog: age=3, Dog: age=2]
After sorting:
[Dog: age=2, Dog: age=3, Dog: age=5]
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 1 images

Recommended textbooks for you
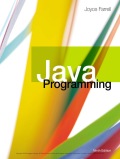
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
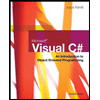
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
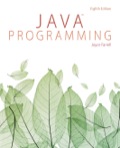
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781305480537
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
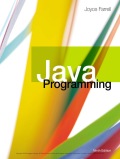
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
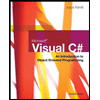
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
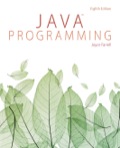
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781305480537
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
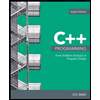
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning