Suppose that you've been hired to produce a program that draws an image of an archery target-or, if you prefer commercial applications, a logo for a national department store chain-that looks like this: This figure is simply three GOval objects, two red and one white, drawn in the correct order. The outer circle should have a radius of 200 pixels. The white circle has a radius 65% of the outer circle radius, and the inner red circle has a radius of 30% of the outer circle radius. The figure should be centered in the window of a TextGraphicsProgram. A skeleton program has been provided for you in Target.java.
Java- Please Do the following way. Don't change anything. Need only 6 lines to compelete this. if you can not do following way then please reject this question. I will attached the question.
import acm.graphics.*;
import acm.program.*;
import java.awt.*;
import tsl.TextGraphicsProgram;
public class Target extends TextGraphicsProgram {
/** Size of the centered rect */
private static final double OUTER_RADIUS_PIXELS = 200;
private static final double OUTER_RADIUS_RATIO = 1.0;
private static final double MIDDLE_RADIUS_RATIO = 0.65;
private static final double INNER_RADIUS_RATIO = 0.3;
/**
* Returns a circle.
* This method is defined for you and it takes 4 arguments:
* centerX: the x coordinate of the center of the circle.
* centerY: the y coordinate of the center of the circle.
* radius: the radius of the circle, in pixels.
* color: the color of the circle.
*/
private GOval getCircle(double centerX, double centerY, double radius, Color color)
{
// You do not need to modify this method, but you should read
// it to understand what it does.
GOval oval = new GOval(2 * radius, 2 * radius);
oval.setFillColor(color);
oval.setFilled(true);
// Here we calculate the location of the upper-left
// corner based on the center point and the radius.
double upperLeftX = centerX - radius;
double upperLeftY = centerY - radius;
oval.setLocation(upperLeftX, upperLeftY);
return oval;
}
public void run()
{
double centerX = getWidth() / 2;
double centerY = getHeight() / 2;
// Here we call our getCircle method.
// I did it once for you -- look at the arguments
// that are passed in, and the result that is
// drawn to the screen.
// Note that we set the location of the object
// when we created it in getCircle, so here we can just
// "add" it without passing the coordinates.
double outerRadius = OUTER_RADIUS_RATIO * OUTER_RADIUS_PIXELS;
GOval outerOval = getCircle(centerX, centerY, outerRadius, Color.RED);
add(outerOval);
// If you aren't sure what's happening with the code above,
// play with it a bit to see how things change:
// * Try changing the values of centerX and centerY.
// * Try changing the value passed in for radius.
// * Try changing the color that is passed in.
// Ok, your turn:
// Do the above steps again for the next circle,
// with slightly different arguments.
// YOUR CODE GOES HERE.
// And call it one more time for the last circle,
// with slightly different argumnts.
// YOUR CODE GOES HERE.
}
}


Trending now
This is a popular solution!
Step by step
Solved in 2 steps

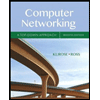
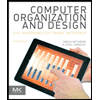
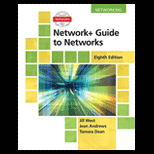
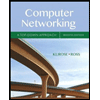
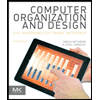
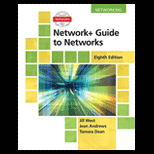
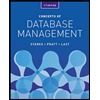
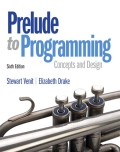
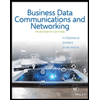