Consider the following Rectangle Class: public class Rectangle { private int width; private int length; public void Rectangle (int w, int len) { width = w; length = len; } /** * if width or length is less than 1 return -1 otherwise return the width * length */ public double findArea() { // code to implement findArea //.... } } What is the output of the following code snippet? Explain your answer. Rectangle r1 = new Rectangle (3, 0); System.out.println(r1.findArea());
Consider the following Rectangle Class: public class Rectangle { private int width; private int length; public void Rectangle (int w, int len) { width = w; length = len; } /** * if width or length is less than 1 return -1 otherwise return the width * length */ public double findArea() { // code to implement findArea //.... } } What is the output of the following code snippet? Explain your answer. Rectangle r1 = new Rectangle (3, 0); System.out.println(r1.findArea());
Chapter2: Using Data
Section: Chapter Questions
Problem 14RQ
Related questions
Question
java

Transcribed Image Text:### QUESTION 17
Consider the following `Rectangle` Class:
```java
public class Rectangle {
private int width;
private int length;
public void Rectangle(int w , int len) {
width = w;
length = len;
}
/**
* if width or length is less than 1 return -1
* otherwise return the width * length
*/
public double findArea() {
// code to implement findArea
// ..............
}
}
```
**What is the output of the following code snippet? Explain your answer.**
```java
Rectangle r1 = new Rectangle(3, 0);
System.out.println(r1.findArea());
```
### Explanation:
In this question, we have a `Rectangle` class with two private data members `width` and `length`. The constructor, named `Rectangle`, initializes these data members. The `findArea` method is supposed to calculate the area of the rectangle and return it. If either `width` or `length` is less than 1, it should return `-1`, otherwise, it should return the product of `width` and `length`.
However, there is an issue:
1. **Constructor Implementation Issue**:
- The constructor is defined as `public void Rectangle(int w, int len)` which makes it a method, not a constructor. Constructors do not have a return type.
- To correct it, the constructor should be defined as:
```java
public Rectangle(int w, int len) {
width = w;
length = len;
}
```
2. **In the `findArea` method**, the implementation for calculating and returning the area is missing. It should be implemented as per the given specification:
```java
public double findArea() {
if (width < 1 || length < 1) {
return -1;
} else {
return width * length;
}
}
```
Given the current state of the `Rectangle` class in the question, attempting to instantiate it and call `findArea` using the following code:
```java
Rectangle r1 = new Rectangle(3, 0);
System.out.println(r1.findArea());
```
This would lead to errors because:
- `Rectangle` is not properly instantiated due to the incorrect constructor.
- `findArea` method is not implemented.
**Correcting the
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
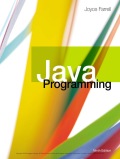
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
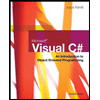
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
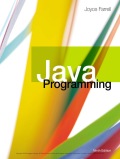
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
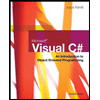
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
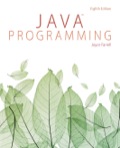
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781305480537
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
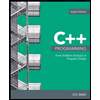
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
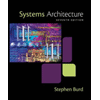
Systems Architecture
Computer Science
ISBN:
9781305080195
Author:
Stephen D. Burd
Publisher:
Cengage Learning