SUBJECT: OOP Programming Language: C++ Write a function to search for student details from file using the enrollment of student. Code: #include #include using namespace std; #define MAX 10
SUBJECT: OOP
Programming Language: C++
Write a function to search for student details from file using the enrollment of student.
Code:
#include <iostream>
#include <conio.h>
using namespace std;
#define MAX 10
class student
{
private:
char name[30];
char sec[30];
char sem[30];
int course1M, course2M, course3M;
int EnrollNo;
int total;
float avg;
char Grade;
public:
student()
{
EnrollNo = 0;
course1M = 0;
course2M = 0;
course3M = 0;
total = 0;
avg = 0;
}
void SetDetails();
void GetDetails();
float grade(float avg);
};
void student::SetDetails()
{
cout << "Enter student name: ";
cin >> name;
cout << "Enter Enrollment number: ";
cin >> EnrollNo;
cout << "Enter semester: ";
cin >> sem;
cout << "Enter Section: ";
cin >> sec;
cout << "Enter course 1 mark out of 100: ";
cin >> course1M;
cout << "Enter course 2 mark out of 100: ";
cin >> course2M;
cout << "Enter course 3 mark out of 100: ";
cin >> course3M;
total = course1M + course2M + course3M;
avg = total / 3;
Grade = grade(avg);
}
float student::grade(float avg)
{
if (avg >= 90 && avg <= 100)
return 'A';
else if (avg >= 80 && avg <= 89)
return 'B';
if (avg >= 70 && avg <= 79)
return 'C';
if (avg >= 60 && avg <= 69)
return 'D';
if (avg >= 50 && avg <= 59)
return 'E';
if (avg <= 49)
return 'F';
}
void student::GetDetails(){
cout << "\nStudent details:";
cout << "\nName:" << name;
cout << "\nEnrollment Number:" << EnrollNo;
cout << "\nSection:" << sec;
cout << "\nSemester:" << sem;
cout << "\nTotal:" << total;
cout << "\nAverage:" << avg;
cout << "\nGrade: " << Grade;
}
int main()
{
student stu[MAX];
int n;
cout << "Enter total number of students: ";
cin >> n;
for (int i = 0; i< n; i++){
cout << "Enter details of student " << i + 1 << ":\n";
stu[i].SetDetails();
}
cout << endl;
for (int i = 0; i< n; i++){
cout << "Details of student " << (i + 1) << ":\n" << endl;
stu[i].GetDetails();
}
_getch();
return 0;
}

Step by step
Solved in 3 steps with 3 images

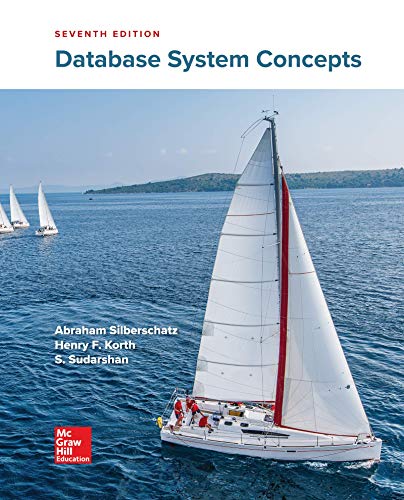
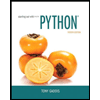
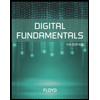
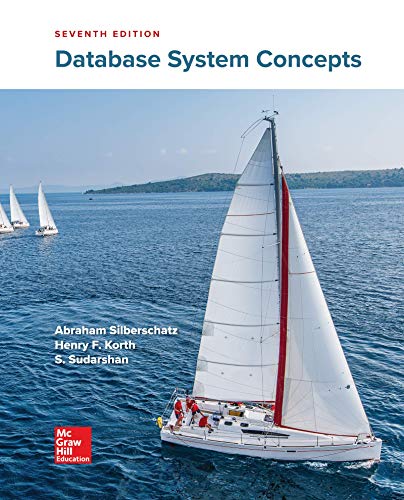
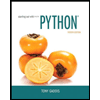
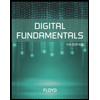
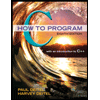
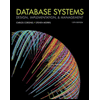
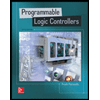