So I need help with a peculiar assignment. I have 3 files, "MainClass.java", "Human.java", & "Student.java". In my Human File, I have the following code.... "public abstract class Human { private String name; private String address; private short age; // constructor that takes only two paras public Human(String name, short age) { this.name = name; this.age = age; } public String getName() { return name; } public void setName(String name) { this.name = name; } public abstract String getAddress() ; public abstract void setAddress(String address); public short getAge() { return age; } public void setAge(short age) { this.age = age; } }" AND in my Student File, I have " public class Student extends Human { // private instance variables for GPA and address private double gpa; private String address; // constructor that takes only two parameters: name and age public Student(String name, short age) { super(name, age); // call the constructor of the parent class } // implementation of abstract getAddress method from Human class @Override public String getAddress() { return address; } // implementation of abstract setAddress method from Human class @Override public void setAddress(String address) { this.address = address; } // getter method for GPA public double getGpa() { return gpa; } // setter method for GPA public void setGpa(double gpa) { this.gpa = gpa; } }". The code in those 2 are all set, But what i really need help with is completeing the following steps in my MainClass file " public class MainClass { public static void main(String[] args) { // ToDo 5: Fix the error // ToDo 6: Fix the constructor of Student class // Todo 7: Create two classes for Freshman and Senior // ToDo 8: The senior class should have a minimum of 85 credits // ToDo 9: Add a toString method for Freshman class // ToDo 10: Add a toString method for Senior class Freshman std1= new Student("James", 20, 12); // name, age, credits Senior std2 = new Student("John", 30, 90); // ToDo 11: Set the gpa of the student using the scanner and user // input and then print the output. System.out.println(std1); System.out.println(std2); // ToDo 12: add comments and explain your code // ToDo 13: submit using a pull request. } }". Can someone type in the correct code for the to-do's?
So I need help with a peculiar assignment. I have 3 files, "MainClass.java", "Human.java", & "Student.java".
In my Human File, I have the following code....
"public abstract class Human {
private String name;
private String address;
private short age;
// constructor that takes only two paras
public Human(String name, short age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public abstract String getAddress() ;
public abstract void setAddress(String address);
public short getAge() {
return age;
}
public void setAge(short age) {
this.age = age;
}
}"
AND in my Student File, I have
"
public class Student extends Human {
// private instance variables for GPA and address
private double gpa;
private String address;
// constructor that takes only two parameters: name and age
public Student(String name, short age) {
super(name, age); // call the constructor of the parent class
}
// implementation of abstract getAddress method from Human class
@Override
public String getAddress() {
return address;
}
// implementation of abstract setAddress method from Human class
@Override
public void setAddress(String address) {
this.address = address;
}
// getter method for GPA
public double getGpa() {
return gpa;
}
// setter method for GPA
public void setGpa(double gpa) {
this.gpa = gpa;
}
}".
The code in those 2 are all set, But what i really need help with is completeing the following steps in my MainClass file
"
public class MainClass {
public static void main(String[] args) {
// ToDo 5: Fix the error
// ToDo 6: Fix the constructor of Student class
// Todo 7: Create two classes for Freshman and Senior
// ToDo 8: The senior class should have a minimum of 85 credits
// ToDo 9: Add a toString method for Freshman class
// ToDo 10: Add a toString method for Senior class
Freshman std1= new Student("James", 20, 12); // name, age, credits
Senior std2 = new Student("John", 30, 90);
// ToDo 11: Set the gpa of the student using the scanner and user
// input and then print the output.
System.out.println(std1);
System.out.println(std2);
// ToDo 12: add comments and explain your code
// ToDo 13: submit using a pull request.
}
}". Can someone type in the correct code for the to-do's?

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

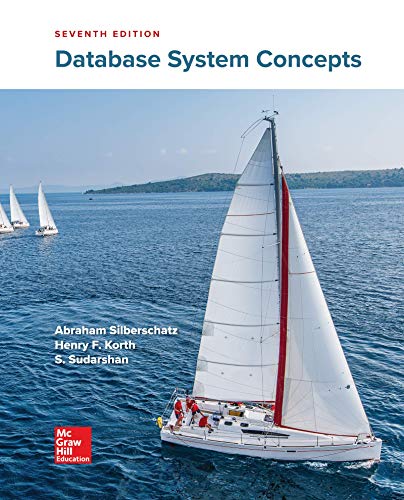
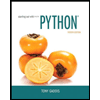
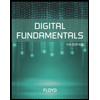
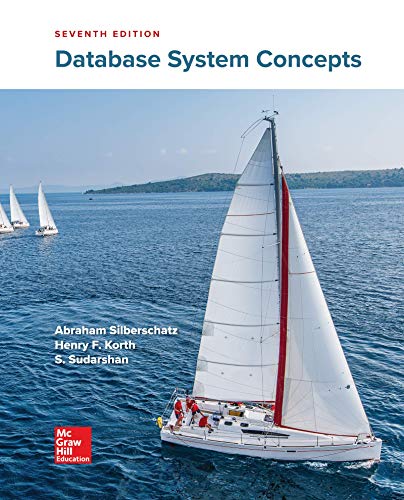
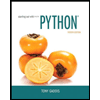
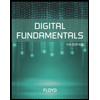
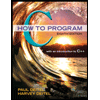
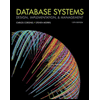
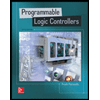