//I have a line of Java code that is giving me an error I do not understand. Below is an excerpt that includes the issue: class Person { // superclass private String name; private String address; private int phoneNum; // data members, privatized by request Person(){} Person(String lname, String laddress, int lphoneNum) { name = lname; address = laddress; phoneNum = lphoneNum; } // constructor that allows the main class to call this method for processing public String getName() {return name;} public String getAddress() {return address;} public int getPhoneNum() {return phoneNum;} //methods for returning requested parameters @Override public String toString() { return ("Person: "+name); // the output of this class, which will display the requested information as the output of this program. } } class Student extends Person { private String grade; // data member, privatized by request Student(){} Student(String lname, String laddress, int lphoneNum, String lgrade) { super(lname, laddress, lphoneNum); lgrade = grade; } // constructor that allows the main class to call this method for processing private String getGrade() {return grade;} //method for returning requested parameter @Override public String toString() { return ("Student: "+name+"("+grade+")"); // the output of this class, which will display the requested information as the output of this program. } } //The system tells me that 'name' has private access in Person. I know that, but I assumed that by extending Person into Student, that would not be an issue. What is the problem??
//I have a line of Java code that is giving me an error I do not understand. Below is an excerpt that includes the issue:
class Person { // superclass
private String name;
private String address;
private int phoneNum; // data members, privatized by request
Person(){}
Person(String lname, String laddress, int lphoneNum) {
name = lname;
address = laddress;
phoneNum = lphoneNum;
} // constructor that allows the main class to call this method for processing
public String getName() {return name;}
public String getAddress() {return address;}
public int getPhoneNum() {return phoneNum;} //methods for returning requested parameters
@Override
public String toString() {
return ("Person: "+name); // the output of this class, which will display the requested information as the output of this
}
}
class Student extends Person {
private String grade; // data member, privatized by request
Student(){}
Student(String lname, String laddress, int lphoneNum, String lgrade) {
super(lname, laddress, lphoneNum);
lgrade = grade;
} // constructor that allows the main class to call this method for processing
private String getGrade() {return grade;} //method for returning requested parameter
@Override
public String toString() {
return ("Student: "+name+"("+grade+")"); // the output of this class, which will display the requested information as the output of this program.
}
}
//The system tells me that 'name' has private access in Person. I know that, but I assumed that by extending Person into Student, that would not be an issue. What is the problem??

Step by step
Solved in 3 steps

I did use a getter function in the Person class, as shown above:
public String getName() {return name;}
But I still got this error. Why is this, and what do I need to change?
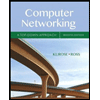
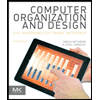
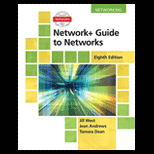
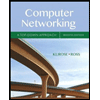
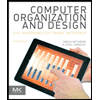
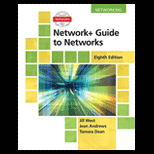
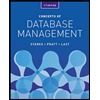
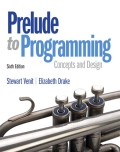
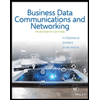