HW 4 Code (In Java) import java.util.Scanner; class Movie { private String name; private String mipaaRating; private int terrible; private int bad; private int ok; private int good; private int great; public Movie(String name, String mipaaRating) { this.name = name; this.mipaaRating = mipaaRating; this.terrible = 0; this.bad = 0; this.ok = 0; this.good = 0; this.great = 0; } //Adding the ratings for Terrible to Excellent rating public void addRating(int rating) { if (rating >= 1 && rating <= 5) { switch (rating) { case 1: this.terrible++; break; case 2: this.bad++; break; case 3: this.ok++; break; case 4: this.good++; break; case 5: this.great++; break; } } } // Finds the average for every single rating when given public double getAverage() { int total = this.terrible + this.bad + this.ok + this.good + this.great; if (total == 0) { return 0; } else { double sum = this.terrible + (2 * this.bad) + (3 * this.ok) + (4 * this.good) + (5 * this.great); double avg = sum / total; return Math.round(avg * 100.0) / 100.0; } } public String getName() { return this.name; } public String getMipaaRating() { return this.mipaaRating; } // Prints out the # of ratings public void printRatings() { System.out.println("Number of people rating the movie as 1 (Terrible): " + this.terrible); System.out.println("Number of people rating the movie as 2 (Bad): " + this.bad); System.out.println("Number of people rating the movie as 3 (OK): " + this.ok); System.out.println("Number of people rating the movie as 4 (Good): " + this.good); System.out.println("Number of people rating the movie as 5 (Great): " + this.great); } } //Main code for the processing of the coding public class TestMovieRating { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); System.out.print("Enter the name of the movie: "); String name = scanner.nextLine(); System.out.print("Enter the MIPAA rating of the movie: "); String mipaaRating = scanner.nextLine(); Movie movie = new Movie(name, mipaaRating); //For implementing the ratings int rating = 0; while (rating != -1) { System.out.print("Enter a rating between 1 and 5 (-1 to stop): "); rating = scanner.nextInt(); //When to stop the rating if all are the ratings are filled in if (rating == -1) { break; } else { movie.addRating(rating); } } System.out.println("Name: " + movie.getName()); System.out.println("MIPAA rating: " + movie.getMipaaRating()); movie.printRatings(); System.out.println("Average rating: " + movie.getAverage()); } }
HW 4 Code (In Java) import java.util.Scanner; class Movie { private String name; private String mipaaRating; private int terrible; private int bad; private int ok; private int good; private int great; public Movie(String name, String mipaaRating) { this.name = name; this.mipaaRating = mipaaRating; this.terrible = 0; this.bad = 0; this.ok = 0; this.good = 0; this.great = 0; } //Adding the ratings for Terrible to Excellent rating public void addRating(int rating) { if (rating >= 1 && rating <= 5) { switch (rating) { case 1: this.terrible++; break; case 2: this.bad++; break; case 3: this.ok++; break; case 4: this.good++; break; case 5: this.great++; break; } } } // Finds the average for every single rating when given public double getAverage() { int total = this.terrible + this.bad + this.ok + this.good + this.great; if (total == 0) { return 0; } else { double sum = this.terrible + (2 * this.bad) + (3 * this.ok) + (4 * this.good) + (5 * this.great); double avg = sum / total; return Math.round(avg * 100.0) / 100.0; } } public String getName() { return this.name; } public String getMipaaRating() { return this.mipaaRating; } // Prints out the # of ratings public void printRatings() { System.out.println("Number of people rating the movie as 1 (Terrible): " + this.terrible); System.out.println("Number of people rating the movie as 2 (Bad): " + this.bad); System.out.println("Number of people rating the movie as 3 (OK): " + this.ok); System.out.println("Number of people rating the movie as 4 (Good): " + this.good); System.out.println("Number of people rating the movie as 5 (Great): " + this.great); } } //Main code for the processing of the coding public class TestMovieRating { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); System.out.print("Enter the name of the movie: "); String name = scanner.nextLine(); System.out.print("Enter the MIPAA rating of the movie: "); String mipaaRating = scanner.nextLine(); Movie movie = new Movie(name, mipaaRating); //For implementing the ratings int rating = 0; while (rating != -1) { System.out.print("Enter a rating between 1 and 5 (-1 to stop): "); rating = scanner.nextInt(); //When to stop the rating if all are the ratings are filled in if (rating == -1) { break; } else { movie.addRating(rating); } } System.out.println("Name: " + movie.getName()); System.out.println("MIPAA rating: " + movie.getMipaaRating()); movie.printRatings(); System.out.println("Average rating: " + movie.getAverage()); } }
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
HW 4 Code (In Java)
import java.util.Scanner;
class Movie {
private String name;
private String mipaaRating;
private int terrible;
private int bad;
private int ok;
private int good;
private int great;
public Movie(String name, String mipaaRating) {
this.name = name;
this.mipaaRating = mipaaRating;
this.terrible = 0;
this.bad = 0;
this.ok = 0;
this.good = 0;
this.great = 0;
}
//Adding the ratings for Terrible to Excellent rating
public void addRating(int rating) {
if (rating >= 1 && rating <= 5) {
switch (rating) {
case 1:
this.terrible++;
break;
case 2:
this.bad++;
break;
case 3:
this.ok++;
break;
case 4:
this.good++;
break;
case 5:
this.great++;
break;
}
}
}
// Finds the average for every single rating when given
public double getAverage() {
int total = this.terrible + this.bad + this.ok + this.good + this.great;
if (total == 0) {
return 0;
} else {
double sum = this.terrible + (2 * this.bad) + (3 * this.ok) + (4 * this.good) + (5 * this.great);
double avg = sum / total;
return Math.round(avg * 100.0) / 100.0;
}
}
public String getName() {
return this.name;
}
public String getMipaaRating() {
return this.mipaaRating;
}
// Prints out the # of ratings
public void printRatings() {
System.out.println("Number of people rating the movie as 1 (Terrible): " + this.terrible);
System.out.println("Number of people rating the movie as 2 (Bad): " + this.bad);
System.out.println("Number of people rating the movie as 3 (OK): " + this.ok);
System.out.println("Number of people rating the movie as 4 (Good): " + this.good);
System.out.println("Number of people rating the movie as 5 (Great): " + this.great);
}
}
//Main code for the processing of the coding
public class TestMovieRating {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter the name of the movie: ");
String name = scanner.nextLine();
System.out.print("Enter the MIPAA rating of the movie: ");
String mipaaRating = scanner.nextLine();
Movie movie = new Movie(name, mipaaRating);
//For implementing the ratings
int rating = 0;
while (rating != -1) {
System.out.print("Enter a rating between 1 and 5 (-1 to stop): ");
rating = scanner.nextInt();
//When to stop the rating if all are the ratings are filled in
if (rating == -1) {
break;
} else {
movie.addRating(rating);
}
}
System.out.println("Name: " + movie.getName());
System.out.println("MIPAA rating: " + movie.getMipaaRating());
movie.printRatings();
System.out.println("Average rating: " + movie.getAverage());
}
}
class Movie {
private String name;
private String mipaaRating;
private int terrible;
private int bad;
private int ok;
private int good;
private int great;
public Movie(String name, String mipaaRating) {
this.name = name;
this.mipaaRating = mipaaRating;
this.terrible = 0;
this.bad = 0;
this.ok = 0;
this.good = 0;
this.great = 0;
}
//Adding the ratings for Terrible to Excellent rating
public void addRating(int rating) {
if (rating >= 1 && rating <= 5) {
switch (rating) {
case 1:
this.terrible++;
break;
case 2:
this.bad++;
break;
case 3:
this.ok++;
break;
case 4:
this.good++;
break;
case 5:
this.great++;
break;
}
}
}
// Finds the average for every single rating when given
public double getAverage() {
int total = this.terrible + this.bad + this.ok + this.good + this.great;
if (total == 0) {
return 0;
} else {
double sum = this.terrible + (2 * this.bad) + (3 * this.ok) + (4 * this.good) + (5 * this.great);
double avg = sum / total;
return Math.round(avg * 100.0) / 100.0;
}
}
public String getName() {
return this.name;
}
public String getMipaaRating() {
return this.mipaaRating;
}
// Prints out the # of ratings
public void printRatings() {
System.out.println("Number of people rating the movie as 1 (Terrible): " + this.terrible);
System.out.println("Number of people rating the movie as 2 (Bad): " + this.bad);
System.out.println("Number of people rating the movie as 3 (OK): " + this.ok);
System.out.println("Number of people rating the movie as 4 (Good): " + this.good);
System.out.println("Number of people rating the movie as 5 (Great): " + this.great);
}
}
//Main code for the processing of the coding
public class TestMovieRating {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter the name of the movie: ");
String name = scanner.nextLine();
System.out.print("Enter the MIPAA rating of the movie: ");
String mipaaRating = scanner.nextLine();
Movie movie = new Movie(name, mipaaRating);
//For implementing the ratings
int rating = 0;
while (rating != -1) {
System.out.print("Enter a rating between 1 and 5 (-1 to stop): ");
rating = scanner.nextInt();
//When to stop the rating if all are the ratings are filled in
if (rating == -1) {
break;
} else {
movie.addRating(rating);
}
}
System.out.println("Name: " + movie.getName());
System.out.println("MIPAA rating: " + movie.getMipaaRating());
movie.printRatings();
System.out.println("Average rating: " + movie.getAverage());
}
}

Transcribed Image Text:Consider the class Movie in Homework #4.
1. Define a class Documentary that is derived from the Movie class. Documentary inherits
the name, MIPAA rating from the Movie class. In addition, Documentary contains TV rating
of the type String. For the Documentary class, implement:
A constructor that creates an instance of a documentary by accepting inputs for all the
instance variables: -- name, MIPAA rating, TV rating.
Accessor and mutator methods for all the instance variables. Bear in mind that some
instance variables and methods could be inherited from the class Movie.
writeOutput () method that prints the values of all the instance variables for a given
documentary.
2. Extend the class Documentary to Documentary Series. Documentary series inherits
the name, MIPAA rating from the Movie class, and TV rating from the Documentary class. In
addition, documentary series adds two variables -- number of seasons, and number of episodes
of type int. For the DocumentarySeries class, implement:
A constructor that creates an instance of a documentary Series by accepting inputs for all
the instance variables: -- name, MIPAA rating, TV rating, number of seasons, and number
of episodes.
Accessor and mutator methods for all the instance variables. Bear in mind that some
instance variables and methods could be inherited from the classes Movie and
Documentary.
writeOutput () method that prints the values of all the instance variables for a given
documentary series.
Write a test client TestDocumetaries.java that tests the implementation of the
Documentary and Documentary Series classes. This test client prompts for a data file
which contains the details of each documentary or a documentary series; one per line. The
attributes on each line are separated by commas. There is no special indicator to distinguish
between a documentary and a documentary series, except that the documentary series will have
two extra attributes. For example, considering the following two lines, Alone is a documentary
series due to the presence of two extra attributes - it has 9 seasons and 107 episodes, whereas
The Tindler Swindler is a documentary due to the absence of these two attributes.
Alone, R, TV-14, 9, 107
The Tindler Swindler, R, TV-MA
The test client prints an aggregate report summarizing the contents of the data file. This
aggregate report will print the total number of documentaries which are not documentary series,
the total number of documentary series, the total numbers for each MPAA and TV-Ratings,
documentary series with the greatest number of seasons, and documentary series with the
greatest number of episodes. The set of MPAA and TV ratings are not known in advance, and will
be discovered on the fly from the contents of the data file. For example, the data file above results
in the following output:

Transcribed Image Text:Number of Documentaries: 1
Number of Documentary Series: 1
Number of R Documentaries: 2
Number of TV-14 Documentaries: 1
Number of TV-MA Documentaries: 1
Alone is the documentary series with highest number of seasons - 9
Alone is the documentary series with highest number of episodes - 107
OUTPUT:
Output - Movie (run)
D
D
run:
Number of Documentaries: 1
Number of Documentary Series: 1
Number of R Documentaries: 2
Number of TV-14 Documentaries: 1
Number of TV-MA Documentaries: 1
Alone is the documentary series with highest number of seasons -9
Alone is the documentary series with highest number of seasons 107
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
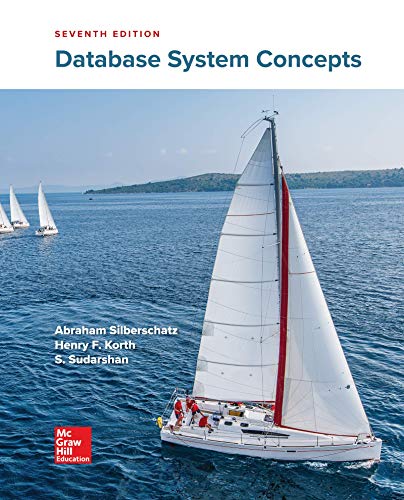
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
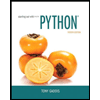
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
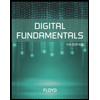
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
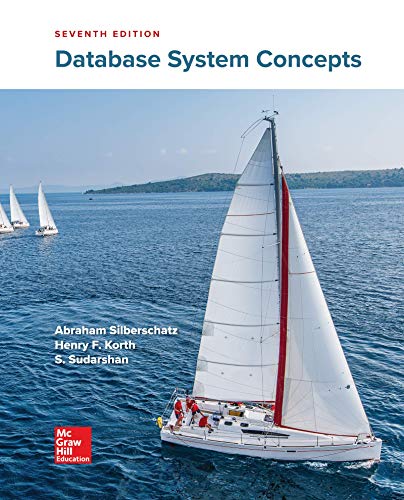
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
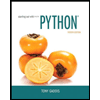
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
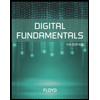
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
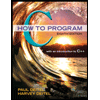
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
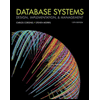
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
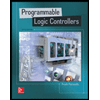
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education