Sample Valid Passwords (with valid message): ABC2%def (valid) A3b$cd$2*efg (valid) Sample Invalid Passwords (with invalid error message) : Abcdel# (invalid - must be between 8 and 12 characters) aBc3$defGhijk (invalid - must be between 8 and 12 characters) ABC2%DEF (invalid - must contain at least one lowercase letter) abc2%def (invalid - must contain at least one uppercase letter) ABC$$ DEF (invalid - must contain at least one numerical digit) 3ABC2%def (invalid - cannot begin or end with numeric digit) ABC2%def4 (invalid - cannot begin or end with numeric digit) A3b$cd#25^ef (invalid - cannot contain consecutive numeric digits) aBC2defg (invalid - must contain at least one special character) ABC2%d$^ef (invalid - cannot contain more than three special characters) aBC24d%f (invalid - cannot have repeated special characters)
This is a
public static void main(String[] args) {
Scanner console= new Scanner(System.in);
welcomeMenu();
boolean finished = false;
while(!finished) {
displayMenu();
String response= userInput(console);
finished= getOption(console, response);
}
System.out.println("You are exiting this program. Thanks for usign it. See you later!");
}
//method that takes the user-entered password
public static void gettingPassword(Scanner console) {
System.out.println("Please enter password: ");
String password = console.next();
passwordLength(password);
validatePassword(password);
}
//method that welcomes the user and explain the task achieved by the program
public static void welcomeMenu() {
System.out.println("Welcome to password verification!");
System.out.println("This program determines if your password meet all requirements!");
System.out.println();
}
//method that dispalys the requirements of the password
public static void passwordRequirements() {
System.out.println("\tIt must be in between 8 to 12 characters.");
System.out.println("\tIt must have at least one lower case letter (a to z).");
System.out.println("\tIt must have at least one uppercase letter (A to Z).");
System.out.println("\tIt must contain at least one numerical digit.");
System.out.println("\tIt cannot begin or end with a numertical digit.");
System.out.println("\tIt must contain at least one of these special "
+ "characters (@, #, $, %, ^).");
System.out.println("\tNo more than 3 special characters are allowed.");
System.out.println("\tYou cannot include a special character more than once.");
System.out.println();
}
public static void displayMenu() {
System.out.println("Please enter one of these three options:");
System.out.println("\tD for Displaying Password Requirements. ");
System.out.println("\tV for Verifying New Password.");
System.out.println("\tE to Exist The Program.");
System.out.println();
}
private static String userInput(Scanner console) {
String selection = console.nextLine();
return selection;
}
public static boolean getOption(Scanner console, String optionSelected) {
boolean finished= false;
if (!optionSelected.equalsIgnoreCase("E")) {
if (optionSelected.equalsIgnoreCase("D")) {
passwordRequirements();
}
else if(optionSelected.equalsIgnoreCase("V")) {
gettingPassword(console);
}
else {
System.out.println("Option entered invalid, please try again!");
}
}
else {
finished= true;
}
return finished;
}
public static boolean passwordLength(String password) {
if (password.length() >= 8 && password.length() <=12) {
return true;
}
else {
System.out.println("Invalid password.\nIt must be in between 8 to 12 characters");
System.out.println();
}
return false;
}
// method that checks if the string (password) meets all requirements
public static void validatePassword(String str){
boolean flag =true;
int upperCaseCount = 0, lowerCaseCount = 0, digitCount = 0, specialCharCount = 0;
char ch1 =str.charAt((str.length()-1));
char c = ' ';
char ch = str.charAt(0);
if ((ch >= '0' && ch <= '9') || (ch1 >= '0' && ch1 <= '9')){
System.out.println("Invalid.");
flag = false;
}
else{
for(int i = 0; i < str.length(); i++) {
ch = str.charAt(i);
if (ch >= 'A' && ch <= 'Z'){
upperCaseCount++;
}
else if (ch >= 'a' && ch <= 'z'){
lowerCaseCount++;
}
else if (ch >= '0' && ch <= '9'){
if(i != (str.length()-1))
{
ch1 = str.charAt((i + 1));
if ((ch >= '0' && ch <= '9') && (ch1 >= '0' && ch1 <= '9')){
System.out.println("A");
flag = false;
break;
}
}
digitCount++;
}
else{
if (c==ch){
System.out.println("Invalid");
flag = false;
break;
}
else{
c=ch;
}
specialCharCount++;
}
}
if( upperCaseCount == 0 || lowerCaseCount == 0 || digitCount==0 || specialCharCount==0 || specialCharCount > 3)
{
System.out.println("Invalid");
flag = false;
}
}
if (flag)
{
System.out.println("Invalid");
}
}


Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 10 images

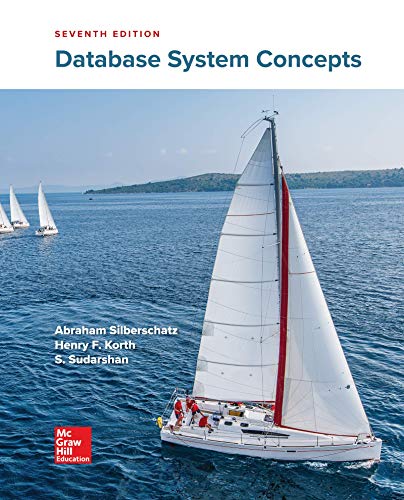
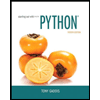
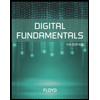
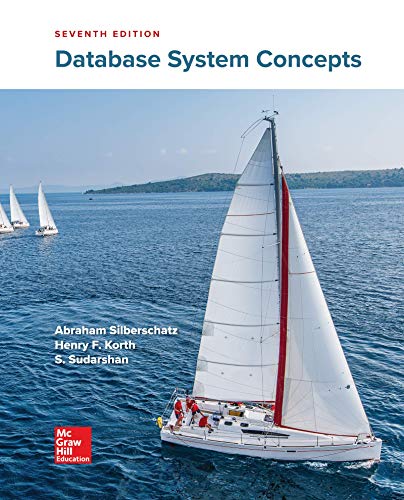
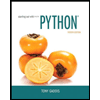
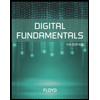
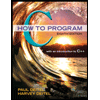
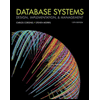
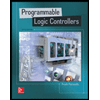