Run this JAVA code in netbeans and take screenshots as proof of its implementation: / Java Program to demonstrate the// working of Abstract Factory Pattern enum CarType{ MICRO, MINI, LUXURY} abstract class Car{ Car(CarType model, Location location) { this.model = model; this.location = location; } abstract void construct(); CarType model = null; Location location = null; CarType getModel() { return model; } void setModel(CarType model) { this.model = model; } Location getLocation() { return location; } void setLocation(Location location) { this.location = location; } @Override public String toString() { return "CarModel - "+model + " located in "+location; }} class LuxuryCar extends Car{ LuxuryCar(Location location) { super(CarType.LUXURY, location); construct(); } @Override protected void construct() { System.out.println("Connecting to luxury car"); }} class MicroCar extends Car{ MicroCar(Location location) { super(CarType.MICRO, location); construct(); } @Override protected void construct() { System.out.println("Connecting to Micro Car "); }} class MiniCar extends Car{ MiniCar(Location location) { super(CarType.MINI,location ); construct(); } @Override void construct() { System.out.println("Connecting to Mini car"); }} enum Location{DEFAULT, USA, INDIA} class INDIACarFactory{ static Car buildCar(CarType model) { Car car = null; switch (model) { case MICRO: car = new MicroCar(Location.INDIA); break; case MINI: car = new MiniCar(Location.INDIA); break; case LUXURY: car = new LuxuryCar(Location.INDIA); break; default: break; } return car; }} class DefaultCarFactory{ public static Car buildCar(CarType model) { Car car = null; switch (model) { case MICRO: car = new MicroCar(Location.DEFAULT); break; case MINI: car = new MiniCar(Location.DEFAULT); break; case LUXURY: car = new LuxuryCar(Location.DEFAULT); break; default: break; } return car; }} class USACarFactory{ public static Car buildCar(CarType model) { Car car = null; switch (model) { case MICRO: car = new MicroCar(Location.USA); break; case MINI: car = new MiniCar(Location.USA); break; case LUXURY: car = new LuxuryCar(Location.USA); break; default: break; } return car; }} class CarFactory{ private CarFactory() { } public static Car buildCar(CarType type) { Car car = null; // We can add any GPS Function here which // read location property somewhere from configuration // and use location specific car factory // Currently I'm just using INDIA as Location Location location = Location.INDIA; switch(location) { case USA: car = USACarFactory.buildCar(type); break; case INDIA: car = INDIACarFactory.buildCar(type); break; default: car = DefaultCarFactory.buildCar(type); } return car; }} class AbstractDesign{ public static void main(String[] args) { System.out.println(CarFactory.buildCar(CarType.MICRO)); System.out.println(CarFactory.buildCar(CarType.MINI)); System.out.println(CarFactory.buildCar(CarType.LUXURY)); }}
Run this JAVA code in netbeans and take screenshots as proof of its implementation: / Java Program to demonstrate the// working of Abstract Factory Pattern enum CarType{ MICRO, MINI, LUXURY} abstract class Car{ Car(CarType model, Location location) { this.model = model; this.location = location; } abstract void construct(); CarType model = null; Location location = null; CarType getModel() { return model; } void setModel(CarType model) { this.model = model; } Location getLocation() { return location; } void setLocation(Location location) { this.location = location; } @Override public String toString() { return "CarModel - "+model + " located in "+location; }} class LuxuryCar extends Car{ LuxuryCar(Location location) { super(CarType.LUXURY, location); construct(); } @Override protected void construct() { System.out.println("Connecting to luxury car"); }} class MicroCar extends Car{ MicroCar(Location location) { super(CarType.MICRO, location); construct(); } @Override protected void construct() { System.out.println("Connecting to Micro Car "); }} class MiniCar extends Car{ MiniCar(Location location) { super(CarType.MINI,location ); construct(); } @Override void construct() { System.out.println("Connecting to Mini car"); }} enum Location{DEFAULT, USA, INDIA} class INDIACarFactory{ static Car buildCar(CarType model) { Car car = null; switch (model) { case MICRO: car = new MicroCar(Location.INDIA); break; case MINI: car = new MiniCar(Location.INDIA); break; case LUXURY: car = new LuxuryCar(Location.INDIA); break; default: break; } return car; }} class DefaultCarFactory{ public static Car buildCar(CarType model) { Car car = null; switch (model) { case MICRO: car = new MicroCar(Location.DEFAULT); break; case MINI: car = new MiniCar(Location.DEFAULT); break; case LUXURY: car = new LuxuryCar(Location.DEFAULT); break; default: break; } return car; }} class USACarFactory{ public static Car buildCar(CarType model) { Car car = null; switch (model) { case MICRO: car = new MicroCar(Location.USA); break; case MINI: car = new MiniCar(Location.USA); break; case LUXURY: car = new LuxuryCar(Location.USA); break; default: break; } return car; }} class CarFactory{ private CarFactory() { } public static Car buildCar(CarType type) { Car car = null; // We can add any GPS Function here which // read location property somewhere from configuration // and use location specific car factory // Currently I'm just using INDIA as Location Location location = Location.INDIA; switch(location) { case USA: car = USACarFactory.buildCar(type); break; case INDIA: car = INDIACarFactory.buildCar(type); break; default: car = DefaultCarFactory.buildCar(type); } return car; }} class AbstractDesign{ public static void main(String[] args) { System.out.println(CarFactory.buildCar(CarType.MICRO)); System.out.println(CarFactory.buildCar(CarType.MINI)); System.out.println(CarFactory.buildCar(CarType.LUXURY)); }}
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Concept explainers
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Question
100%
Run this JAVA code in netbeans and take screenshots as proof of its implementation:
/ Java Program to demonstrate the
// working of Abstract Factory Pattern
// working of Abstract Factory Pattern
enum CarType
{
MICRO, MINI, LUXURY
}
{
MICRO, MINI, LUXURY
}
abstract class Car
{
Car(CarType model, Location location)
{
this.model = model;
this.location = location;
}
{
Car(CarType model, Location location)
{
this.model = model;
this.location = location;
}
abstract void construct();
CarType model = null;
Location location = null;
Location location = null;
CarType getModel()
{
return model;
}
{
return model;
}
void setModel(CarType model)
{
this.model = model;
}
{
this.model = model;
}
Location getLocation()
{
return location;
}
{
return location;
}
void setLocation(Location location)
{
this.location = location;
}
{
this.location = location;
}
@Override
public String toString()
{
return "CarModel - "+model + " located in "+location;
}
}
public String toString()
{
return "CarModel - "+model + " located in "+location;
}
}
class LuxuryCar extends Car
{
LuxuryCar(Location location)
{
super(CarType.LUXURY, location);
construct();
}
@Override
protected void construct()
{
System.out.println("Connecting to luxury car");
}
}
{
LuxuryCar(Location location)
{
super(CarType.LUXURY, location);
construct();
}
@Override
protected void construct()
{
System.out.println("Connecting to luxury car");
}
}
class MicroCar extends Car
{
MicroCar(Location location)
{
super(CarType.MICRO, location);
construct();
}
@Override
protected void construct()
{
System.out.println("Connecting to Micro Car ");
}
}
{
MicroCar(Location location)
{
super(CarType.MICRO, location);
construct();
}
@Override
protected void construct()
{
System.out.println("Connecting to Micro Car ");
}
}
class MiniCar extends Car
{
MiniCar(Location location)
{
super(CarType.MINI,location );
construct();
}
@Override
void construct()
{
System.out.println("Connecting to Mini car");
}
}
{
MiniCar(Location location)
{
super(CarType.MINI,location );
construct();
}
@Override
void construct()
{
System.out.println("Connecting to Mini car");
}
}
enum Location
{
DEFAULT, USA, INDIA
}
{
DEFAULT, USA, INDIA
}
class INDIACarFactory
{
static Car buildCar(CarType model)
{
Car car = null;
switch (model)
{
case MICRO:
car = new MicroCar(Location.INDIA);
break;
case MINI:
car = new MiniCar(Location.INDIA);
break;
case LUXURY:
car = new LuxuryCar(Location.INDIA);
break;
default:
break;
}
return car;
}
}
{
static Car buildCar(CarType model)
{
Car car = null;
switch (model)
{
case MICRO:
car = new MicroCar(Location.INDIA);
break;
case MINI:
car = new MiniCar(Location.INDIA);
break;
case LUXURY:
car = new LuxuryCar(Location.INDIA);
break;
default:
break;
}
return car;
}
}
class DefaultCarFactory
{
public static Car buildCar(CarType model)
{
Car car = null;
switch (model)
{
case MICRO:
car = new MicroCar(Location.DEFAULT);
break;
case MINI:
car = new MiniCar(Location.DEFAULT);
break;
case LUXURY:
car = new LuxuryCar(Location.DEFAULT);
break;
default:
break;
}
return car;
}
}
{
public static Car buildCar(CarType model)
{
Car car = null;
switch (model)
{
case MICRO:
car = new MicroCar(Location.DEFAULT);
break;
case MINI:
car = new MiniCar(Location.DEFAULT);
break;
case LUXURY:
car = new LuxuryCar(Location.DEFAULT);
break;
default:
break;
}
return car;
}
}
class USACarFactory
{
public static Car buildCar(CarType model)
{
Car car = null;
switch (model)
{
case MICRO:
car = new MicroCar(Location.USA);
break;
case MINI:
car = new MiniCar(Location.USA);
break;
case LUXURY:
car = new LuxuryCar(Location.USA);
break;
default:
break;
}
return car;
}
}
class CarFactory
{
private CarFactory()
{
}
public static Car buildCar(CarType type)
{
Car car = null;
// We can add any GPS Function here which
// read location property somewhere from configuration
// and use location specific car factory
// Currently I'm just using INDIA as Location
Location location = Location.INDIA;
switch(location)
{
case USA:
car = USACarFactory.buildCar(type);
break;
case INDIA:
car = INDIACarFactory.buildCar(type);
break;
default:
car = DefaultCarFactory.buildCar(type);
{
private CarFactory()
{
}
public static Car buildCar(CarType type)
{
Car car = null;
// We can add any GPS Function here which
// read location property somewhere from configuration
// and use location specific car factory
// Currently I'm just using INDIA as Location
Location location = Location.INDIA;
switch(location)
{
case USA:
car = USACarFactory.buildCar(type);
break;
case INDIA:
car = INDIACarFactory.buildCar(type);
break;
default:
car = DefaultCarFactory.buildCar(type);
}
return car;
return car;
}
}
}
class AbstractDesign
{
public static void main(String[] args)
{
System.out.println(CarFactory.buildCar(CarType.MICRO));
System.out.println(CarFactory.buildCar(CarType.MINI));
System.out.println(CarFactory.buildCar(CarType.LUXURY));
}
}
{
public static void main(String[] args)
{
System.out.println(CarFactory.buildCar(CarType.MICRO));
System.out.println(CarFactory.buildCar(CarType.MINI));
System.out.println(CarFactory.buildCar(CarType.LUXURY));
}
}
Expert Solution

Trending now
This is a popular solution!
Step by step
Solved in 9 steps with 9 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
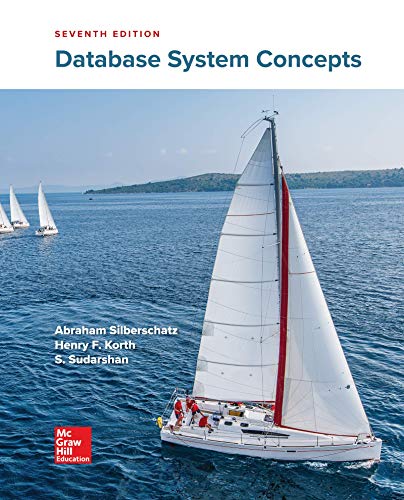
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
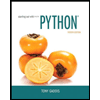
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
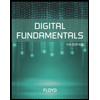
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
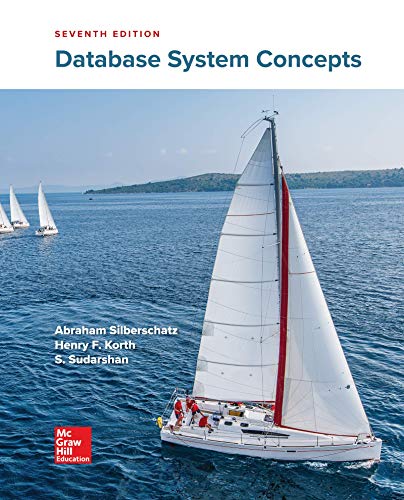
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
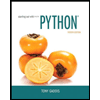
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
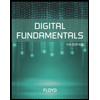
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
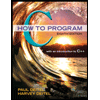
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
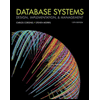
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
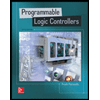
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education