Consider the following three Java classes: public abstract class Toy { public void makeNoise() { System.out.println("DEFAULT SOUND"); } } public class Doll extends Toy { public void makeNoise() { System.out.println("Mama!"); } }public class ToyRobot extends Toy { private int volume; public ToyRobot() { this.volume = 5; } public void makeNoise() { System.out.println("BEEP"); } public int getVolume() { return volume; } public void setVolume(int volume) { this.volume = volume; } } Given these classes, determine what will be printed when each of the code snippets below are copied into the following main method at the indicated location. public static void main(String[] args) { Toy toy; Doll doll; ToyRobot robot1; ToyRobot robot2; doll = new Doll(); robot1 = new ToyRobot(); robot2 = robot1; toy = robot1; // INSERT CODE HERE. } A) doll.makeNoise(); ["", "", "", ""] B) toy.makeNoise(); ["", "", "", ""] C) robot1.setVolume(11); System.out.print(robot1.getVolume() + " "); System.out.print(robot2.getVolume()); ["", "", "", "", ""] D) toy.setVolume(11); System.out.print(toy.getVolume()); ["", "", ""] E) toy = new Toy(); toy.makeNoise(); ["", "", "", ""] F) robot2 = toy; toy.makeNoise(); ["", "", "", ""] The options for ["", "", "", ""] are Default sound, This code will not compile, Beep, Mama!
Consider the following three Java classes:
public abstract class Toy {
public void makeNoise() { System.out.println("DEFAULT SOUND"); }
} public class Doll extends Toy {
public void makeNoise() { System.out.println("Mama!"); }
}public class ToyRobot extends Toy {
private int volume; public ToyRobot() { this.volume = 5; } public void makeNoise() { System.out.println("BEEP"); } public int getVolume() { return volume; } public void setVolume(int volume) { this.volume = volume; }
}
Given these classes, determine what will be printed when each of the code snippets below are copied into the following main method at the indicated location.
public static void main(String[] args) { Toy toy; Doll doll; ToyRobot robot1; ToyRobot robot2; doll = new Doll(); robot1 = new ToyRobot(); robot2 = robot1; toy = robot1; // INSERT CODE HERE. }
A)
doll.makeNoise();
["", "", "", ""]
B)
toy.makeNoise();
["", "", "", ""]
C)
robot1.setVolume(11); System.out.print(robot1.getVolume() + " "); System.out.print(robot2.getVolume());
["", "", "", "", ""]
D)
toy.setVolume(11); System.out.print(toy.getVolume());
["", "", ""]
E)
toy = new Toy(); toy.makeNoise();
["", "", "", ""]
F)
robot2 = toy; toy.makeNoise();
["", "", "", ""]
The options for ["", "", "", ""] are
Default sound, This code will not compile, Beep, Mama!

Trending now
This is a popular solution!
Step by step
Solved in 6 steps

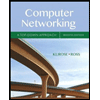
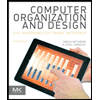
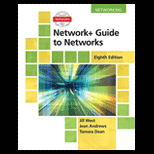
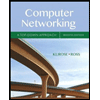
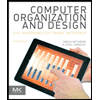
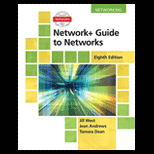
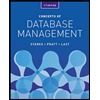
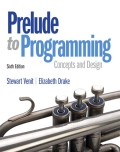
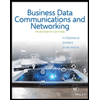