rotate function in C language
I am trying to write a rotate function in C language.


Program:
#include <stdio.h>
#include <stdlib.h>
// declaration of row and columns in square matrix
const int M = 3;
const int N = 3;
// function to rotate square matrix clock-wise
void rotate_right(char a[M][N])
{
int i,j;
for(i=0;i<3;i++)
{
for(j=2;j>=0;j--)
{
// print the result
printf("%c ",a[j][i]);
}
printf("\n");
}
}
// main function
int main()
{
// square matrix
char a[3][3] = {'a', 'b', 'c','d', 'e', 'f', 'h', 'i', 'j'};
int i,j;
// print the matrix
printf("Original Array\n");
for(i=0;i<3;i++)
{
for(j=0;j<3;j++)
{
printf("%c ",a[i][j]);
}
printf("\n");
}
// function call of rotate_right function and print the result
printf("\nRotate Matrix by 90 degrees\n");
rotate_right(a);
return 0;
}
Step by step
Solved in 2 steps with 1 images

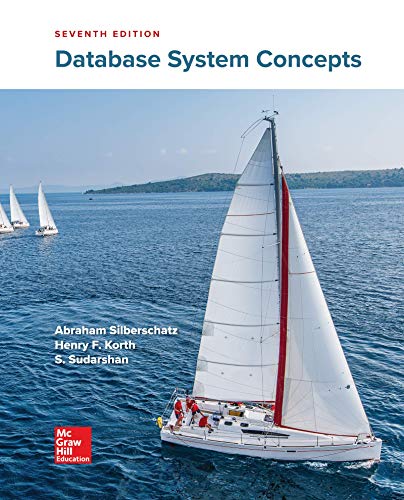
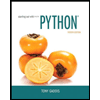
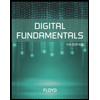
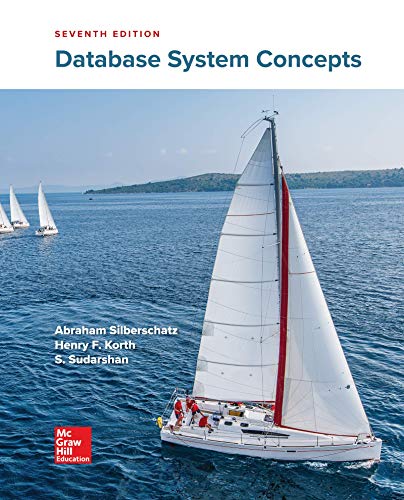
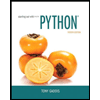
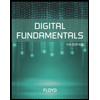
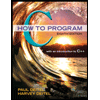
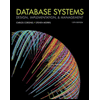
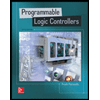