14.13 LAB: Matrix multiplication (2D arrays) A matrix is a rectangle of numbers in rows and columns. A 1xN matrix has one row and N columns. An NxN matrix has N rows and N columns. Multiplying a 1xN matrix A and an NxN matrix B produces a 1xN matrix C. To determine the Nth element of C multiply each element of A by each element of the Nth column of B and sum the results. Helpful information can be found at matrix multiplication. Write a program that reads a 1xN matrix A and an NxN matrix B from input and outputs the 1xN matrix product, C. The first integer input is N, followed by one row of N integers for matrix A and then N rows of N integers for matrix B. N can be of any size >= 2. For coding simplicity, follow each output integer by a space, even the last one. The output ends with a newline. Ex If the input is: 2 23 12 34 A contains 2 and 3, the first row of B contains 1 and 2, and the second row of B contains 3 and 4. The first element of C is (2* 1) + (3*3), and the second element of C is (2*2)+(3*4). The program output is: 11 16 Note: Store matrices A and C into one-dimensional arrays and matrix B into a two-dimensional array. 6257118:2790725 සංශියනුයි. LAB ACTIVITY 14.13.1: LAB: Matrix multiplication (2D arrays) 1 #include 2 using namespace std; 3 main.cpp 0/10 Load default template.....
14.13 LAB: Matrix multiplication (2D arrays) A matrix is a rectangle of numbers in rows and columns. A 1xN matrix has one row and N columns. An NxN matrix has N rows and N columns. Multiplying a 1xN matrix A and an NxN matrix B produces a 1xN matrix C. To determine the Nth element of C multiply each element of A by each element of the Nth column of B and sum the results. Helpful information can be found at matrix multiplication. Write a program that reads a 1xN matrix A and an NxN matrix B from input and outputs the 1xN matrix product, C. The first integer input is N, followed by one row of N integers for matrix A and then N rows of N integers for matrix B. N can be of any size >= 2. For coding simplicity, follow each output integer by a space, even the last one. The output ends with a newline. Ex If the input is: 2 23 12 34 A contains 2 and 3, the first row of B contains 1 and 2, and the second row of B contains 3 and 4. The first element of C is (2* 1) + (3*3), and the second element of C is (2*2)+(3*4). The program output is: 11 16 Note: Store matrices A and C into one-dimensional arrays and matrix B into a two-dimensional array. 6257118:2790725 සංශියනුයි. LAB ACTIVITY 14.13.1: LAB: Matrix multiplication (2D arrays) 1 #include 2 using namespace std; 3 main.cpp 0/10 Load default template.....
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
In C++

Transcribed Image Text:**14.13 LAB: Matrix multiplication (2D arrays)**
A matrix is a rectangle of numbers in rows and columns. A 1xN matrix has one row and N columns. An N×N matrix has N rows and N columns.
Multiplying a 1×N matrix A and an N×N matrix B produces a 1×N matrix C. To determine the Nth element of C, multiply each element of A by each element of the Nth column of B and sum the results. Helpful information can be found at matrix multiplication.
Write a program that reads a 1×N matrix A and an N×N matrix B from input and outputs the 1×N matrix product, C. The first integer input is N, followed by one row of N integers for matrix A and then N rows of N integers for matrix B. N can be of any size >= 2.
For coding simplicity, follow each output integer by a space, even the last one. The output ends with a newline.
**Ex: If the input is:**
```
2
2 3
1 2
3 4
```
A contains 2 and 3, the first row of B contains 1 and 2, and the second row of B contains 3 and 4. The first element of C is (2 * 1) + (3 * 3), and the second element of C is (2 * 2) + (3 * 4). The program output is:
```
11 16
```
**Note:** Store matrices A and C into one-dimensional arrays and matrix B into a two-dimensional array.
**Code:**
```cpp
#include <iostream>
using namespace std;
int main() {
int n;
// Code continues
```
![### Matrix Multiplication (2D Arrays) - Lab Activity
#### Example Input:
```
2
3
1 2
3 4
```
#### Explanation:
- Matrix A contains: 2 and 3
- The first row of Matrix B contains: 1 and 2
- The second row of Matrix B contains: 3 and 4
To compute Matrix C:
- The first element of C is calculated as (2 * 1) + (3 * 3) = 11
- The second element of C is (2 * 2) + (3 * 4) = 16
The program outputs:
```
11 16
```
#### Note:
- Store matrix A and matrix C in one-dimensional arrays.
- Store matrix B in a two-dimensional array.
---
### Lab Activity: Matrix Multiplication Code Setup
This is the initial code setup for performing matrix multiplication:
```cpp
#include <iostream>
using namespace std;
int main() {
int n;
cin >> n;
int matrixA[n]; // Matrix A
int matrixB[n][n]; // Matrix B
int matrixC[n]; // Matrix C
/* Type your code here. */
return 0;
}
```
**Instructions:**
- Use the provided code template.
- Implement the logic for matrix multiplication based on the input format.
**Development Environment:**
- Use the "Develop mode" to program and test your code as often as needed.
- After ensuring your program works, click "Submit mode" to submit for grading.
- To run the program, input your test values and click "Run program" to view the output.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fda17b6d9-f7ff-4a56-9763-458bee0c35b1%2Fbb451e0b-bdc9-45c3-a00c-10320fe11648%2Fqu1br9a_processed.png&w=3840&q=75)
Transcribed Image Text:### Matrix Multiplication (2D Arrays) - Lab Activity
#### Example Input:
```
2
3
1 2
3 4
```
#### Explanation:
- Matrix A contains: 2 and 3
- The first row of Matrix B contains: 1 and 2
- The second row of Matrix B contains: 3 and 4
To compute Matrix C:
- The first element of C is calculated as (2 * 1) + (3 * 3) = 11
- The second element of C is (2 * 2) + (3 * 4) = 16
The program outputs:
```
11 16
```
#### Note:
- Store matrix A and matrix C in one-dimensional arrays.
- Store matrix B in a two-dimensional array.
---
### Lab Activity: Matrix Multiplication Code Setup
This is the initial code setup for performing matrix multiplication:
```cpp
#include <iostream>
using namespace std;
int main() {
int n;
cin >> n;
int matrixA[n]; // Matrix A
int matrixB[n][n]; // Matrix B
int matrixC[n]; // Matrix C
/* Type your code here. */
return 0;
}
```
**Instructions:**
- Use the provided code template.
- Implement the logic for matrix multiplication based on the input format.
**Development Environment:**
- Use the "Develop mode" to program and test your code as often as needed.
- After ensuring your program works, click "Submit mode" to submit for grading.
- To run the program, input your test values and click "Run program" to view the output.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
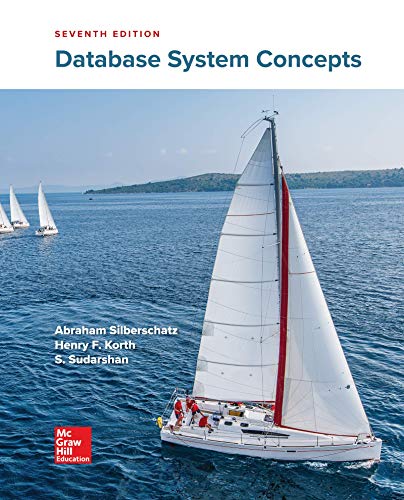
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
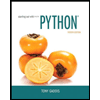
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
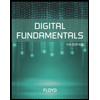
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
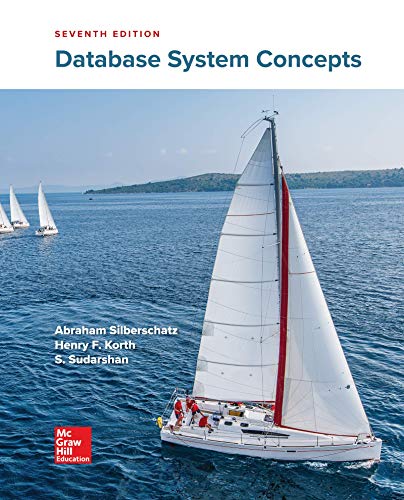
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
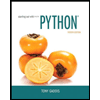
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
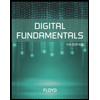
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
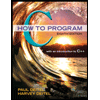
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
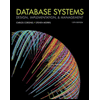
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
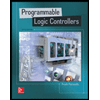
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education