Rewrite Program 3 where the following operators are overloaded: >> << + - * == != Also overload the preincrement and postincrement operators ++ which will change the value of the complex number to its cube.
I'm working on c++ and I've been given the following instructions to modify a program:
Rewrite Program 3 where the following operators are overloaded:
>> << + - * == !=
Also overload the preincrement and postincrement operators ++ which will change the value of the complex number to its cube.
I really only need help understanding the last two operators and the pre/postincrement.
Here is the program I already have:
#include <iostream>
using namespace std;
//This program uses a class to work with complex numbers
// create complex number class
class ComplexNum
{
//private variables
private:
float realNum;
float imaginaryNum;
//public section
public:
//default constructor
ComplexNum(float r = 0, float i = 0) :realNum(r), imaginaryNum(i) {};
//sets the complex numbers
void setComplex(void)
{
cout << "Enter the real part: ";
cin >> this->realNum;
cout << "Enter the imaginary part: ";
cin >> this->imaginaryNum;
}
//adds the complex numbers
ComplexNum operator +(const ComplexNum c)
{
ComplexNum complex;
complex.realNum = realNum + c.realNum;
complex.imaginaryNum = imaginaryNum + c.imaginaryNum;
return complex;
}
//subract the complex numbers
ComplexNum operator -(const ComplexNum& c)
{
ComplexNum complex;
complex.realNum = this->realNum - c.realNum;
complex.imaginaryNum = this->imaginaryNum - c.imaginaryNum;
return complex;
}
//multiply the numbers
ComplexNum multiply(const ComplexNum& c)
{
ComplexNum complex;
complex.realNum = (this->realNum * c.realNum) - (this->imaginaryNum * c.imaginaryNum);
complex.imaginaryNum = (this->realNum * c.imaginaryNum) + (this->imaginaryNum * c.realNum);
return complex;
}
//print numbers
void printComplexNum(void)
{
if (this->imaginaryNum >= 0)
cout << this->realNum << " + " << this->imaginaryNum << "i" << endl;
else
cout << this->realNum << " " << this->imaginaryNum << "i" << endl;
}
//cube the numbers
void cube()
{
float ogreal = this->realNum;
float ogimaginary = this->imaginaryNum;
float temp1 = ogreal * ogreal - ogimaginary * ogimaginary;
float temp2 = 2 * ogimaginary * ogreal;
this->realNum = temp1 * ogreal - temp2 * ogimaginary;
this->imaginaryNum = (3 * (ogreal * ogreal) * ogimaginary) - (ogimaginary * ogimaginary * ogimaginary);
}
};
//main
int main()
{
// create complex number objects
ComplexNum first, second, sum, difference, product;
//
cout << "First complex number " << endl;
first.setComplex();
cout << "Second complex number " << endl;
second.setComplex();
//Add
cout << "Sum of a and b: " << endl;
sum = first + second;
sum.printComplexNum();
//Subtract
cout << "Difference of a and b : " << endl;
difference = first - second;
difference.printComplexNum();
//Multiply
cout << "Product of a and b : " << endl;
product = first.multiply(second);
product.printComplexNum();
//Cube
cout << "Cube of a: " << endl;
first.cube();
first.printComplexNum();
/*change the complex number to its cube*/
cout << "Cube of b: " << endl;
second.cube();
second.printComplexNum();
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 7 images

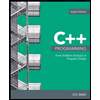
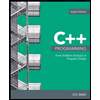