I need help with an assignment that deals with converting characters stored in a character array to all uppercase or all lowercase in C++. The textbook is Programming Logic and Design, Comprehensive 9th Edition (ISBN 978-1-337-10207-0). The following is the code that I have so far, and I would like to know what I am doing wrong: // ChangeCase.cpp - This program converts characters to lowercase and uppercase. // Input: Interactive // Output: Uppercase and lowercase versions of the user-entered string #include #include using namespace std; int main() { const int LEN = 9; char sample1[LEN]; char sample2[LEN]; char result1; char result2; int i; cout << "Enter 9 lowercase characters: "; for(i = 0; i < LEN; i++) { cin >> sample1[i]; // Convert sample1[i] to uppercase and assign it to result1 sample1[i] = toupper(sample1[i]); sample1[i] = result1; cout << result1 << endl; } cin.ignore(256,'\n'); cout << "Enter 9 uppercase characters: "; for(i = 0; i < LEN; i++) { cin >> sample2[i]; // Convert sample2[i] to lowercase and assign it to result2 sample2[i] = tolower(sample2[i]); sample2[i] = result2; cout << result2 << endl; } cin.ignore(256,'\n'); return 0; } // End of main() function
I need help with an assignment that deals with converting characters stored in a character array to all uppercase or all lowercase in C++. The textbook is Programming Logic and Design, Comprehensive 9th Edition (ISBN 978-1-337-10207-0). The following is the code that I have so far, and I would like to know what I am doing wrong: // ChangeCase.cpp - This program converts characters to lowercase and uppercase. // Input: Interactive // Output: Uppercase and lowercase versions of the user-entered string #include #include using namespace std; int main() { const int LEN = 9; char sample1[LEN]; char sample2[LEN]; char result1; char result2; int i; cout << "Enter 9 lowercase characters: "; for(i = 0; i < LEN; i++) { cin >> sample1[i]; // Convert sample1[i] to uppercase and assign it to result1 sample1[i] = toupper(sample1[i]); sample1[i] = result1; cout << result1 << endl; } cin.ignore(256,'\n'); cout << "Enter 9 uppercase characters: "; for(i = 0; i < LEN; i++) { cin >> sample2[i]; // Convert sample2[i] to lowercase and assign it to result2 sample2[i] = tolower(sample2[i]); sample2[i] = result2; cout << result2 << endl; } cin.ignore(256,'\n'); return 0; } // End of main() function
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
100%
I need help with an assignment that deals with converting characters stored in a character array to all uppercase or all lowercase in C++. The textbook is
// ChangeCase.cpp - This program converts characters to lowercase and uppercase.
// Input: Interactive
// Output: Uppercase and lowercase versions of the user-entered string
#include <iostream>
#include <string>
using namespace std;
int main()
{
const int LEN = 9;
char sample1[LEN];
char sample2[LEN];
char result1;
char result2;
int i;
cout << "Enter 9 lowercase characters: ";
for(i = 0; i < LEN; i++)
{
cin >> sample1[i];
// Convert sample1[i] to uppercase and assign it to result1
sample1[i] = toupper(sample1[i]);
sample1[i] = result1;
cout << result1 << endl;
}
cin.ignore(256,'\n');
cout << "Enter 9 uppercase characters: ";
for(i = 0; i < LEN; i++)
{
cin >> sample2[i];
// Convert sample2[i] to lowercase and assign it to result2
sample2[i] = tolower(sample2[i]);
sample2[i] = result2;
cout << result2 << endl;
}
cin.ignore(256,'\n');
return 0;
} // End of main() function
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
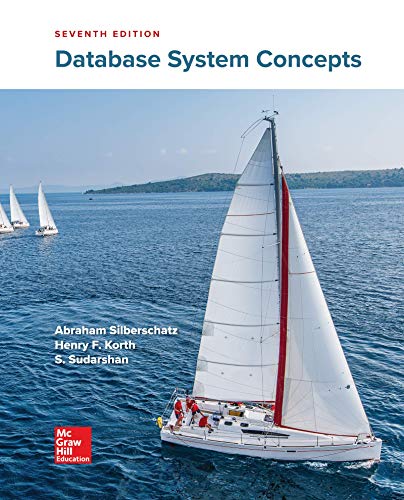
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
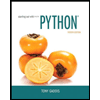
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
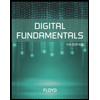
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
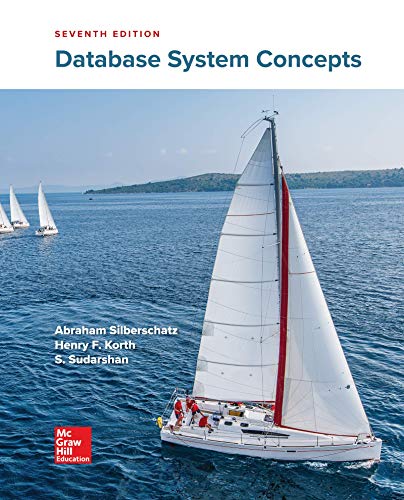
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
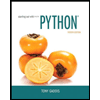
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
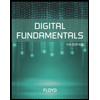
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
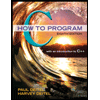
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
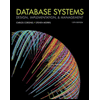
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
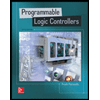
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education