Can you help me with a C++ program: To the class Song you created previously (included below) add functions that perform conversions between songs and strings. More precisely, create a function to_string(s) that takes a song as argument and returns a string that contains three lines with the song data. For example, the 2019 song September Blues by Quantic should convert to the following string: September Blues\nQuantic\n2019\n And create a function to_Song(s) that takes a string as argument and returns a Song object. The string argument is assumed to contain three lines with valid song data, as shown above. Use string streams #include #include using namespace std; class Song { private: string Title; string Artist; int Year; public: Song() : Title("invalid"), Artist("invalid"), Year(-1) {} Song(const string &Title) : Title(Title), Artist("unknown"), Year(-1) {} Song(const string &Title, const string &Artist, int Year) : Title(Title), Artist(Artist), Year(Year) {} void set_title(const string &title) { Title = title; } void set_artist(const string &artist) { Artist = artist; } void set_year(int year) { Year = year; } void set(const string &title, const string &artist, int year) { Title = title; Artist = artist; Year = year; } bool operator==(const Song &other) const { return Title == other.Title && Artist == other.Artist && Year == other.Year; } bool operator<(const Song &other) const { if (Title < other.Title) return true; else if (Title == other.Title) { if (Artist < other.Artist) return true; else if (Artist == other.Artist) { return Year < other.Year; } } return false; } friend std::ostream &operator<<(std::ostream &out, const Song &song) { out << song.Title << " by " << song.Artist << " (" << song.Year << ")"; return out; } friend std::istream &operator>>(std::istream &in, Song &song) { std::string title, artist; int year; in >> title >> artist >> year; song.set(title, artist, year); return in; } const std::string &get_title() const { return Title; } const std::string &get_artist() const { return Artist; } int get_year() const { return Year; } };
Can you help me with a C++ program:
To the class Song you created previously (included below)
add functions that perform conversions between songs and strings. More
precisely, create a function to_string(s) that takes a song as argument
and returns a string that contains three lines with the song data. For example, the 2019 song September Blues by Quantic should convert to the
following string:
September Blues\nQuantic\n2019\n
And create a function to_Song(s) that takes a string as argument and
returns a Song object. The string argument is assumed to contain three
lines with valid song data, as shown above.
Use string streams
#include <iostream>
#include <string>
using namespace std;
class Song {
private:
string Title;
string Artist;
int Year;
public:
Song() : Title("invalid"), Artist("invalid"), Year(-1) {}
Song(const string &Title) : Title(Title), Artist("unknown"), Year(-1) {}
Song(const string &Title, const string &Artist, int Year)
: Title(Title), Artist(Artist), Year(Year) {}
void set_title(const string &title) { Title = title; }
void set_artist(const string &artist) { Artist = artist; }
void set_year(int year) { Year = year; }
void set(const string &title, const string &artist, int year) {
Title = title;
Artist = artist;
Year = year;
}
bool operator==(const Song &other) const {
return Title == other.Title && Artist == other.Artist && Year == other.Year;
}
bool operator<(const Song &other) const {
if (Title < other.Title)
return true;
else if (Title == other.Title) {
if (Artist < other.Artist)
return true;
else if (Artist == other.Artist) {
return Year < other.Year;
}
}
return false;
}
friend std::ostream &operator<<(std::ostream &out, const Song &song) {
out << song.Title << " by " << song.Artist << " (" << song.Year << ")";
return out;
}
friend std::istream &operator>>(std::istream &in, Song &song) {
std::string title, artist;
int year;
in >> title >> artist >> year;
song.set(title, artist, year);
return in;
}
const std::string &get_title() const { return Title; }
const std::string &get_artist() const { return Artist; }
int get_year() const { return Year; }
};

Trending now
This is a popular solution!
Step by step
Solved in 4 steps

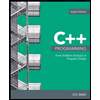
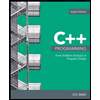