In c++ i need to make a function that removes the modifies the original string to remove the third occurence of a character to only have max of 2 occurences
In c++ i need to make a function that removes the modifies the original string to remove the third occurence of a character to only have max of 2 occurences
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
In c++ i need to make a function that removes the modifies the original string to remove the third occurence of a character to only have max of 2 occurences for example
"aabacdbbddccccc" would be modified to
"aabcdbdc"
in the image below is what i have so far
![```cpp
#include <iostream>
#include <cstring>
void condense(char s[]);
int main()
{
char s[] = {"abcadmmdefagdbfffbdm"};
condense(s);
std::cout << s;
return 0;
}
// INPUT = abcadmmdefagdbfffbdm
// OUTPUT = abcadmmdefgbf
void condense(char s[])
{
int counter;
char *i, *j;
i = s;
j = s;
while (*i != '\0')
{
i++;
while (*j != '\0')
{
j++;
}
}
return;
}
// std::cout << *i;
// i++;
```
This C++ program aims to demonstrate a function named `condense` that is intended to process a character array (string) `s[]`. The initial string is `"abcadmmdefagdbfffbdm"`, and according to the comments, the expected output after processing should be `"abcadmmdefgbf"`. However, the implementation of the `condense` function is incomplete. The inner logic for condensing or modifying the string has not been fully implemented.
### Explanation:
1. **Headers:** The program begins by including the `<iostream>` and `<cstring>` libraries to handle input and output operations and string manipulations.
2. **Function Declaration:** The `condense` function is declared to take a `char` array as an argument.
3. **Main Function:**
- A character array `s[]` is defined with the string `"abcadmmdefagdbfffbdm"`.
- The `condense` function is called with `s` as an argument.
- The (intended) modified string is output using `std::cout`.
4. **Condense Function (Incomplete):**
- Two pointers `i` and `j` are initialized to iterate through the string `s`.
- The outer `while` loop runs until the pointer `i` reaches the null character `'\0'` at the end of the string.
- The inner `while` loop aims to move the pointer `j` in a similar fashion, but lacks logic to modify the string.
5. **Comments:**
- There are comments showing the intended input and the desired output, although this doesn't match the incomplete logic.
- Two commented-out](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Ffedcb44c-84a8-400b-a03e-cc7fbcfb040d%2F77244294-d9d8-412a-bf68-03555815a846%2Frnpk11o_processed.png&w=3840&q=75)
Transcribed Image Text:```cpp
#include <iostream>
#include <cstring>
void condense(char s[]);
int main()
{
char s[] = {"abcadmmdefagdbfffbdm"};
condense(s);
std::cout << s;
return 0;
}
// INPUT = abcadmmdefagdbfffbdm
// OUTPUT = abcadmmdefgbf
void condense(char s[])
{
int counter;
char *i, *j;
i = s;
j = s;
while (*i != '\0')
{
i++;
while (*j != '\0')
{
j++;
}
}
return;
}
// std::cout << *i;
// i++;
```
This C++ program aims to demonstrate a function named `condense` that is intended to process a character array (string) `s[]`. The initial string is `"abcadmmdefagdbfffbdm"`, and according to the comments, the expected output after processing should be `"abcadmmdefgbf"`. However, the implementation of the `condense` function is incomplete. The inner logic for condensing or modifying the string has not been fully implemented.
### Explanation:
1. **Headers:** The program begins by including the `<iostream>` and `<cstring>` libraries to handle input and output operations and string manipulations.
2. **Function Declaration:** The `condense` function is declared to take a `char` array as an argument.
3. **Main Function:**
- A character array `s[]` is defined with the string `"abcadmmdefagdbfffbdm"`.
- The `condense` function is called with `s` as an argument.
- The (intended) modified string is output using `std::cout`.
4. **Condense Function (Incomplete):**
- Two pointers `i` and `j` are initialized to iterate through the string `s`.
- The outer `while` loop runs until the pointer `i` reaches the null character `'\0'` at the end of the string.
- The inner `while` loop aims to move the pointer `j` in a similar fashion, but lacks logic to modify the string.
5. **Comments:**
- There are comments showing the intended input and the desired output, although this doesn't match the incomplete logic.
- Two commented-out
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
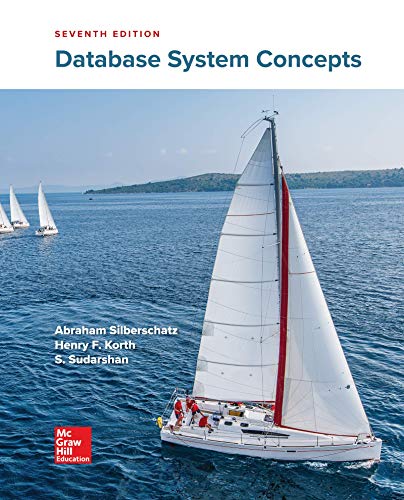
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
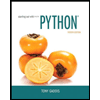
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
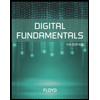
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
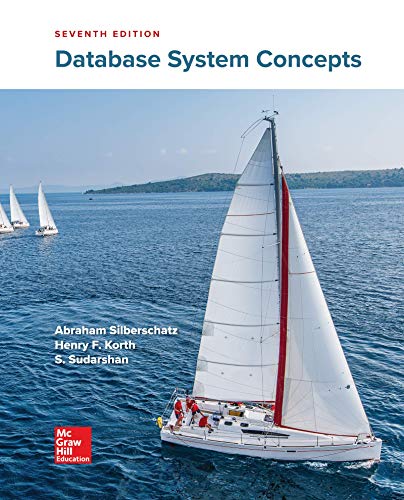
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
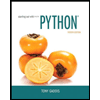
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
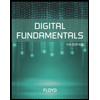
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
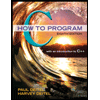
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
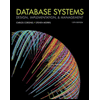
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
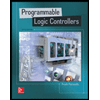
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education