/** * Returns the value associated with the given key in this symbol table. * Takes advantage of the fact that the keys appear in increasing order to terminate * early when possible. * * @param key the key * @return the value associated with the given key if the key is in the symbol table * and {@code null} if the key is not in the symbol table * @throws IllegalArgumentException if {@code key} is {@code null} */ public Value get(Key key) { // TODO // Change this code to make use of the fact the list is sorted to terminate early // when possible. Also, solve this using recursion. To do this, you will need to // add a recursive helper function that takes the front of a list (Node) as an argument // and returns the correct value. if (key == null) throw new IllegalArgumentException("argument to get() is null"); for (Node x = first; x != null; x = x.next) { if (key.equals(x.key)) return x.val; } return null; } Use recusion for this method.
/**
* Returns the value associated with the given key in this symbol table.
* Takes advantage of the fact that the keys appear in increasing order to terminate
* early when possible.
*
* @param key the key
* @return the value associated with the given key if the key is in the symbol table
* and {@code null} if the key is not in the symbol table
* @throws IllegalArgumentException if {@code key} is {@code null}
*/
public Value get(Key key) {
// TODO
// Change this code to make use of the fact the list is sorted to terminate early
// when possible. Also, solve this using recursion. To do this, you will need to
// add a recursive helper function that takes the front of a list (Node) as an argument
// and returns the correct value.
if (key == null) throw new IllegalArgumentException("argument to get() is null");
for (Node x = first; x != null; x = x.next) {
if (key.equals(x.key))
return x.val;
}
return null;
}
Use recusion for this method.

Step by step
Solved in 3 steps

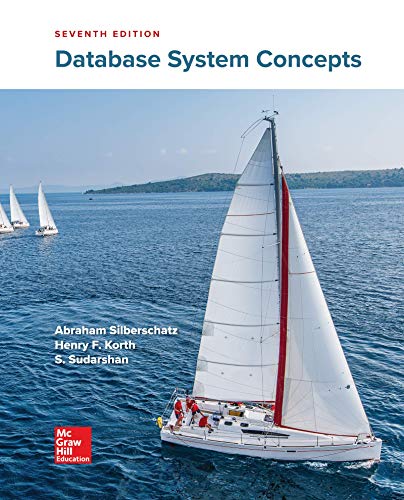
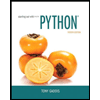
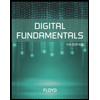
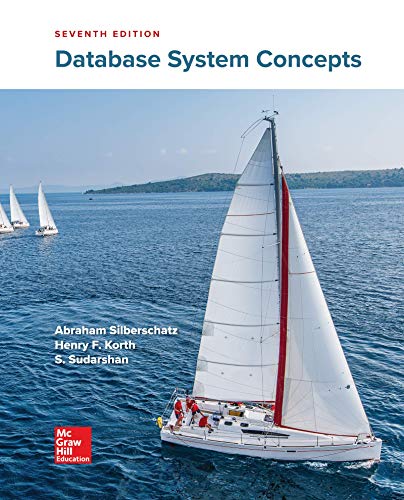
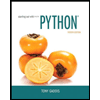
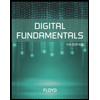
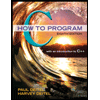
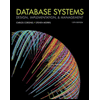
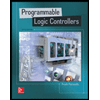