Python Programming I would like to change the program below to where the output is changed to look more like a table like this example below: Part Num Description Quantity Price Total 1001 Hammer 20 15 300 1002 locks 15 5 75 Program: - #Defining the class Invoice class Invoice(object): #Declaring the Variables part_num = ' ' part_description = ' ' quantity = 0 price = 0 #Defining the __init__ method to initialize the variables def __init__(self,part_num,part_description,quantity,price): self.part_num = part_num self.part_description = part_description self.quantity = quantity self.price = price #Defining the method that returns the entered information def display(self): return 'Part number is: ' + self.part_num + '\nPart description is: ' + self.part_description + '\nQuantity of item is: ' + str(self.quantity) + '\nPrice per item is: ' + str(self.price) #Defining the method that calculates and return the Invoice amount def calculate_invoice(self): return self.quantity*self.price #Defining the class method that ask the user to enter the details @classmethod def get_user_input(self): while 1: part_num = input("\nPlease enter the part number: ") part_description = input("Please enter the part description: ") quantity = int(input("Please enter the quantity of item: ")) price = float(input("Please enter the price of item: ")) #Checking the validation if(quantity < 0 or price <= 0): print("Invalid input! Please try again") continue else: return self(part_num,part_description,quantity,price) #Creating the object of Class and calling the class method ob = Invoice.get_user_input() #Calling the method to display the entered data print(ob.display()) #Calling the method to display the Invoice amount print('\nInvoice amount is: {:.2f}'.format(ob.calculate_invoice()))
Python
I would like to change the program below to where the output is changed to look more like a table like this example below:
Part Num Description Quantity Price Total
1001 Hammer 20 15 300
1002 locks 15 5 75
Program: -
#Defining the class Invoice
class Invoice(object):
#Declaring the Variables
part_num = ' '
part_description = ' '
quantity = 0
price = 0
#Defining the __init__ method to initialize the variables
def __init__(self,part_num,part_description,quantity,price):
self.part_num = part_num
self.part_description = part_description
self.quantity = quantity
self.price = price
#Defining the method that returns the entered information
def display(self):
return 'Part number is: ' + self.part_num + '\nPart description is: ' + self.part_description + '\nQuantity of item is: ' + str(self.quantity) + '\nPrice per item is: ' + str(self.price)
#Defining the method that calculates and return the Invoice amount
def calculate_invoice(self):
return self.quantity*self.price
#Defining the class method that ask the user to enter the details
@classmethod
def get_user_input(self):
while 1:
part_num = input("\nPlease enter the part number: ")
part_description = input("Please enter the part description: ")
quantity = int(input("Please enter the quantity of item: "))
price = float(input("Please enter the price of item: "))
#Checking the validation
if(quantity < 0 or price <= 0):
print("Invalid input! Please try again")
continue
else:
return self(part_num,part_description,quantity,price)
#Creating the object of Class and calling the class method
ob = Invoice.get_user_input()
#Calling the method to display the entered data
print(ob.display())
#Calling the method to display the Invoice amount
print('\nInvoice amount is: {:.2f}'.format(ob.calculate_invoice()))

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 3 images

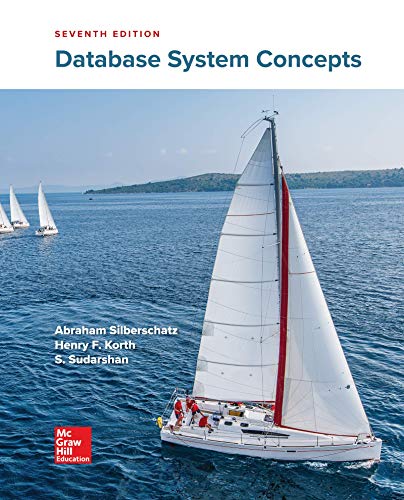
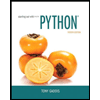
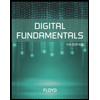
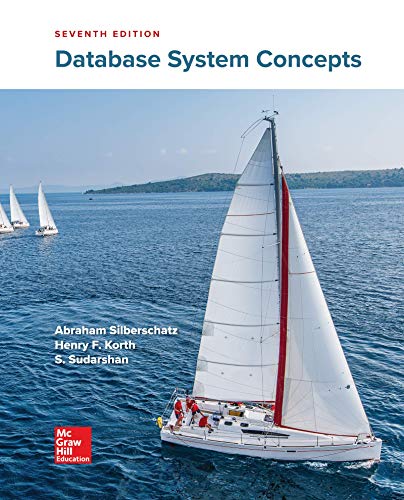
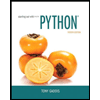
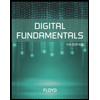
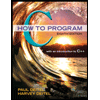
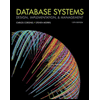
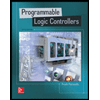