Python I have a class QueryBuilder: def QueryBuilder(Data_Base, Query_Type, Query_Tuple): ''' Build Query_String ''' if Query_Type == "version": query_string = "SELECT sqlite_version()" elif Query_Type == "delete": query_string = "DELETE FROM {0} WHERE id = ?".format(Data_Base) elif Query_Type == "select": query_string = "SELECT * FROM {0} WHERE name = ?".format(Data_Base) elif Query_Type == "insert": query_string = "INSERT INTO {0} (id, name, photo, html) VALUES (?, ?, ?, ?)".format(Data_Base) elif Query_Type == "table": query_string = '''CREATE TABLE Database ( id INTEGER PRIMARY KEY, name TEXT NOT NULL, photo text NOT NULL UNIQUE, html text NOT NULL UNIQUE)'''.format(Data_Base) else: raise ValueError("Invalid query type") return query_string Please use it to write more code below: Purpose: Create and use a Database Data Files: Use BS4, Regular Expressions or Pandas to read in the two data files for this assignment: Co2.html: # Total carbon emissions # (million metric tons of C) yearmonthdecimalaverageinterpolatedtrend#days 195911959.042315.62315.62315.70-1 195921959.125316.38316.38315.88-1 195931959.208316.71316.71315.62-1 195941959.292317.72317.72315.56-1 195951959.375318.29318.29315.50-1 195961959.458318.15318.15315.92-1 195971959.542316.54316.54315.66-1 195981959.625314.80314.80315.81-1 195991959.708313.84313.84316.55-1 1959101959.792313.26313.26316.19-1 1959111959.875314.80314.80316.78-1 ... SeaLevel.csv #title = mean sea level anomaly global ocean (66S to 66N) (Annual signals retained) #institution = NOAA/Laboratory for Satellite Altimetry #references = NOAA Sea Level Rise year,TOPEX/Poseidon,Jason-1,Jason-2,Jason-3 1992.9614,-16.27000, 1992.9865,-17.97000, 1993.0123,-14.87000, 1993.0407,-19.87000, 1993.0660,-25.27000, 1993.0974,-29.37000, 1993.1206,-27.67000, 1993.1493,-21.87000, 1993.1765,-18.97000, 1993.2037,-19.47000, 1993.2307,-22.97000, 1993.2851,-26.27000, 1993.3123,-20.07000, 1993.3394,-19.87000, 1998.8234,6.53000, 1998.8505,2.53000, 1998.8775,-4.07000, 1998.9046,-10.17000, 1998.9319,-3.97000, 1998.9591,-3.27000, 1998.9862,0.13000, 1999.0133,-4.17000, 1999.0405,-6.87000, 1999.0948,-11.17000, 1999.1256,3.73000, 1999.1491,-1.27000, 1999.1763,-6.37000, 1999.2034,-11.77000, 1999.2306,-10.37000, 1999.2577,-7.87000, 1999.2848,-5.37000, 1999.3392,-8.27000, 1999.3663,-13.77000, 2001.6738,5.43000, 2001.7010,15.73000, 2001.7283,16.73000, 2001.7553,14.93000, 2001.7825,7.73000, 2001.8096,4.03000, 2001.8368,11.63000, 2001.8639,16.53000, 2001.8918,14.53000, 2001.9182,10.93000, 2001.9454,4.73000, 2001.9725,3.63000, 2002.1083,-1.67000,6.23000, 2002.1352,6.33000, 2002.1354,-0.17000, 2002.1626,4.43000,4.93000, 2002.1897,4.93000, 2002.1898,-1.27000, ... Where necessary, reduce the data from either Monthly or Daily to Annual data. Use Python iterators and reducers to handle converting the data to Annual data. Store the data in a Pandas Dataframe. Database: Store the Dataframe in an SQLite data base. Design a class to interface to the SQLite database: class Database: def __init__(self): self.db = sqliteConnection() ... and add functionality for table creation, inserting, searching and deleting entries in the database. Use your QueryBuilder to build the SQLite database queries.
Python
I have a class QueryBuilder:
''' Build Query_String '''
if Query_Type == "version":
query_string = "SELECT sqlite_version()"
elif Query_Type == "delete":
query_string = "DELETE FROM {0} WHERE id = ?".format(Data_Base)
elif Query_Type == "select":
query_string = "SELECT * FROM {0} WHERE name = ?".format(Data_Base)
elif Query_Type == "insert":
query_string = "INSERT INTO {0} (id, name, photo, html) VALUES (?, ?, ?, ?)".format(Data_Base)
elif Query_Type == "table":
query_string = '''CREATE TABLE
id INTEGER PRIMARY KEY,
name TEXT NOT NULL,
photo text NOT NULL UNIQUE,
html text NOT NULL UNIQUE)'''.format(Data_Base)
else:
raise ValueError("Invalid query type")
return query_string
Purpose: Create and use a Database
Data Files: Use BS4, Regular Expressions or Pandas to read in the two data files for this assignment:
Co2.html:
<TBODY><TR><TD># Total carbon emissions </TD></TR></TBODY>
<TBODY><TR><TD># (million metric tons of C)</TD></TR></TBODY>
<TBODY><TR><TD>year</TD><TD>month</TD><TD>decimal</TD><TD>average</TD><TD>interpolated</TD><TD>trend</TD><TD>#days</TD></TR></TBODY>
<TBODY><TR><TD>1959</TD><TD>1</TD><TD>1959.042</TD><TD>315.62</TD><TD>315.62</TD><TD>315.70</TD><TD>-1</TD></TR></TBODY>
<TBODY><TR><TD>1959</TD><TD>2</TD><TD>1959.125</TD><TD>316.38</TD><TD>316.38</TD><TD>315.88</TD><TD>-1</TD></TR></TBODY>
<TBODY><TR><TD>1959</TD><TD>3</TD><TD>1959.208</TD><TD>316.71</TD><TD>316.71</TD><TD>315.62</TD><TD>-1</TD></TR></TBODY>
<TBODY><TR><TD>1959</TD><TD>4</TD><TD>1959.292</TD><TD>317.72</TD><TD>317.72</TD><TD>315.56</TD><TD>-1</TD></TR></TBODY>
<TBODY><TR><TD>1959</TD><TD>5</TD><TD>1959.375</TD><TD>318.29</TD><TD>318.29</TD><TD>315.50</TD><TD>-1</TD></TR></TBODY>
<TBODY><TR><TD>1959</TD><TD>6</TD><TD>1959.458</TD><TD>318.15</TD><TD>318.15</TD><TD>315.92</TD><TD>-1</TD></TR></TBODY>
<TBODY><TR><TD>1959</TD><TD>7</TD><TD>1959.542</TD><TD>316.54</TD><TD>316.54</TD><TD>315.66</TD><TD>-1</TD></TR></TBODY>
<TBODY><TR><TD>1959</TD><TD>8</TD><TD>1959.625</TD><TD>314.80</TD><TD>314.80</TD><TD>315.81</TD><TD>-1</TD></TR></TBODY>
<TBODY><TR><TD>1959</TD><TD>9</TD><TD>1959.708</TD><TD>313.84</TD><TD>313.84</TD><TD>316.55</TD><TD>-1</TD></TR></TBODY>
<TBODY><TR><TD>1959</TD><TD>10</TD><TD>1959.792</TD><TD>313.26</TD><TD>313.26</TD><TD>316.19</TD><TD>-1</TD></TR></TBODY>
<TBODY><TR><TD>1959</TD><TD>11</TD><TD>1959.875</TD><TD>314.80</TD><TD>314.80</TD><TD>316.78</TD><TD>-1</TD></TR></TBODY>
SeaLevel.csv
#institution = NOAA/Laboratory for Satellite Altimetry
#references = NOAA Sea Level Rise
year,TOPEX/Poseidon,Jason-1,Jason-2,Jason-3
1992.9614,-16.27000,
1992.9865,-17.97000,
1993.0123,-14.87000,
1993.0407,-19.87000,
1993.0660,-25.27000,
1993.0974,-29.37000,
1993.1206,-27.67000,
1993.1493,-21.87000,
1993.1765,-18.97000,
1993.2037,-19.47000,
1993.2307,-22.97000,
1993.2851,-26.27000,
1993.3123,-20.07000,
1993.3394,-19.87000,
1998.8234,6.53000,
1998.8505,2.53000,
1998.8775,-4.07000,
1998.9046,-10.17000,
1998.9319,-3.97000,
1998.9591,-3.27000,
1998.9862,0.13000,
1999.0133,-4.17000,
1999.0405,-6.87000,
1999.0948,-11.17000,
1999.1256,3.73000,
1999.1491,-1.27000,
1999.1763,-6.37000,
1999.2034,-11.77000,
1999.2306,-10.37000,
1999.2577,-7.87000,
1999.2848,-5.37000,
1999.3392,-8.27000,
1999.3663,-13.77000,
2001.6738,5.43000,
2001.7010,15.73000,
2001.7283,16.73000,
2001.7553,14.93000,
2001.7825,7.73000,
2001.8096,4.03000,
2001.8368,11.63000,
2001.8639,16.53000,
2001.8918,14.53000,
2001.9182,10.93000,
2001.9454,4.73000,
2001.9725,3.63000,
2002.1083,-1.67000,6.23000,
2002.1352,6.33000,
2002.1354,-0.17000,
2002.1626,4.43000,4.93000,
2002.1897,4.93000,
2002.1898,-1.27000,
Where necessary, reduce the data from either Monthly or Daily to Annual data. Use Python iterators and reducers to handle converting the data to Annual data. Store the data in a Pandas Dataframe.
Database:
Store the Dataframe in an SQLite data base. Design a class to interface to the SQLite database:
class Database:
def __init__(self):
self.db = sqliteConnection()
...
and add functionality for table creation, inserting, searching and deleting entries in the database. Use your QueryBuilder to build the SQLite database queries.

Trending now
This is a popular solution!
Step by step
Solved in 4 steps

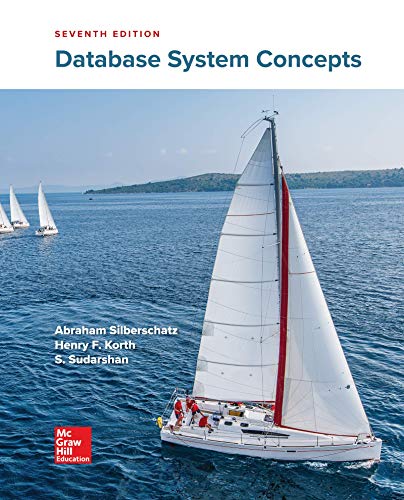
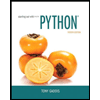
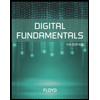
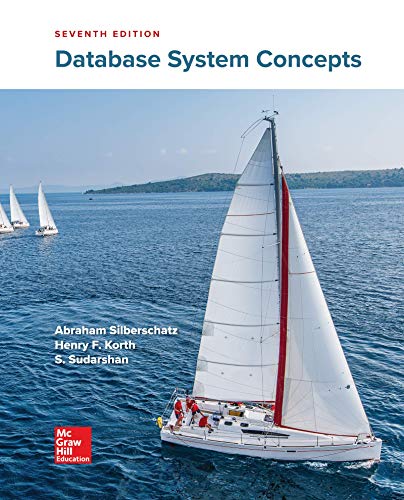
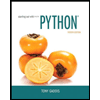
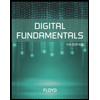
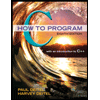
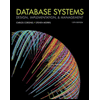
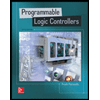