Python code: code I have so far: def print_menu(usr_str: str): aamenu_options = ['c', 'w', 'f', 'r', 's', 'q'] print('\nMENU') print('c - Number of non-whitespace characters') print('w - Number of words') print('f - Fix capitalization') print('r - Replace punctuation') print('s - Shorten spaces') print('q - Quit\n') aachoice = input('Choose an option: ').lower() while aachoice not in aamenu_options: print('Invalid choice') aachoice = input('Choose an option:') if aachoice == 'c': print('Number of non-whitespace characters:', get_num_of_non_WS_characters(usr_str)) elif aachoice == 'w': print('Number of words:', get_num_of_words(usr_str)) elif aachoice == 'f': usr, count = fix_capitalization(usr_str) print('Number of letters capitalized:', count) print('Edited text:', usr_str) elif aachoice == 'r': usr_str = replaced_punctuation(usr_str) print('Edited text:', usr_str) elif aachoice == 's': usr_str = shorten_space(usr_str) print('Edited text:', usr_str) return aachoice, usr_str def get_num_of_non_WS_characters(usr_str:str): count = len(usr_str.replace('','')) return count def get_num_of_words(usr_str: str): num_words = len(usr_str.split()) return num_words def fix_capitalization(usr_str): count = 0 edited_str = '' check = False for i, s in enumerate(usr_str): if i == 0: if s.islower(): count += 1 edited_str += s.upper() else: edited_str += s continue if check: if s.isspace(): edited_str +=s elif s.islower(): count += 1 edited_str += s.upper() check = False else: check = False edited_str += s if s == '.': check = True return usr_str, count def replace_punctuation(usr_str: str, **kwargs): exclamation_count = 0 semicolon_count = 0 result = '' for i in usr_str: if i == '!': i = '.' exclamation_count += 1 elif i == ';': i = ',' semicolon_count += 1 result += i print('Punctuation replaced', exclamation_count) print('Exclamation Count:', semicolon_count) return result def shorten_space(usr_str:str): usr_str = usr_str.strip() result = '' prev = False for i in usr_str: if i.isspace(): if not prev: result += i prev = True else: result += i prev = False return result def main(): usr_str = input('Enter a sample text: \n') print('\nYou entered:', usr_str) choice = '' while choice != 'q': choice, usr_str = print_menu(usr_str) if __name__ == '__main__': main() used input in the problem: we'll continue our quest in space. there will be more shuttle flights and more shuttle crews and, yes; more volunteers, more civilians, more teachers in space. nothing ends here; our hopes and our journeys continue! problems: c(whitespace): mine = 221; correct = 181 f(Fix capitalization): mine = 1, correct = 3 s(Shorten spaces): mine = (no output); correct: Edited text: we'll continue our quest in space. there will be more shuttle flights and more shuttle crews and, yes; more volunteers, more civilians, more teachers in space. nothing ends here; our hopes and our journeys continue! it tells me to use .isspace()?? I have attatched a picture of my code that did not work. Thank you so much and have a great day
Python code:
code I have so far:
def print_menu(usr_str: str):
aamenu_options = ['c', 'w', 'f', 'r', 's', 'q']
print('\nMENU')
print('c - Number of non-whitespace characters')
print('w - Number of words')
print('f - Fix capitalization')
print('r - Replace punctuation')
print('s - Shorten spaces')
print('q - Quit\n')
aachoice = input('Choose an option: ').lower()
while aachoice not in aamenu_options:
print('Invalid choice')
aachoice = input('Choose an option:')
if aachoice == 'c':
print('Number of non-whitespace characters:', get_num_of_non_WS_characters(usr_str))
elif aachoice == 'w':
print('Number of words:', get_num_of_words(usr_str))
elif aachoice == 'f':
usr, count = fix_capitalization(usr_str)
print('Number of letters capitalized:', count)
print('Edited text:', usr_str)
elif aachoice == 'r':
usr_str = replaced_punctuation(usr_str)
print('Edited text:', usr_str)
elif aachoice == 's':
usr_str = shorten_space(usr_str)
print('Edited text:', usr_str)
return aachoice, usr_str
def get_num_of_non_WS_characters(usr_str:str):
count = len(usr_str.replace('',''))
return count
def get_num_of_words(usr_str: str):
num_words = len(usr_str.split())
return num_words
def fix_capitalization(usr_str):
count = 0
edited_str = ''
check = False
for i, s in enumerate(usr_str):
if i == 0:
if s.islower():
count += 1
edited_str += s.upper()
else:
edited_str += s
continue
if check:
if s.isspace():
edited_str +=s
elif s.islower():
count += 1
edited_str += s.upper()
check = False
else:
check = False
edited_str += s
if s == '.':
check = True
return usr_str, count
def replace_punctuation(usr_str: str, **kwargs):
exclamation_count = 0
semicolon_count = 0
result = ''
for i in usr_str:
if i == '!':
i = '.'
exclamation_count += 1
elif i == ';':
i = ','
semicolon_count += 1
result += i
print('Punctuation replaced', exclamation_count)
print('Exclamation Count:', semicolon_count)
return result
def shorten_space(usr_str:str):
usr_str = usr_str.strip()
result = ''
prev = False
for i in usr_str:
if i.isspace():
if not prev:
result += i
prev = True
else:
result += i
prev = False
return result
def main():
usr_str = input('Enter a sample text: \n')
print('\nYou entered:', usr_str)
choice = ''
while choice != 'q':
choice, usr_str = print_menu(usr_str)
if __name__ == '__main__':
main()
used input in the problem:
we'll continue our quest in space. there will be more shuttle flights and more shuttle crews and, yes; more volunteers, more civilians, more teachers in space. nothing ends here; our hopes and our journeys continue!
problems:
c(whitespace): mine = 221; correct = 181
f(Fix capitalization): mine = 1, correct = 3
s(Shorten spaces): mine = (no output); correct: Edited text: we'll continue our quest in space. there will be more shuttle flights and more shuttle crews and, yes; more volunteers, more civilians, more teachers in space. nothing ends here; our hopes and our journeys continue!
it tells me to use .isspace()??
I have attatched a picture of my code that did not work.
Thank you so much and have a great day


Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

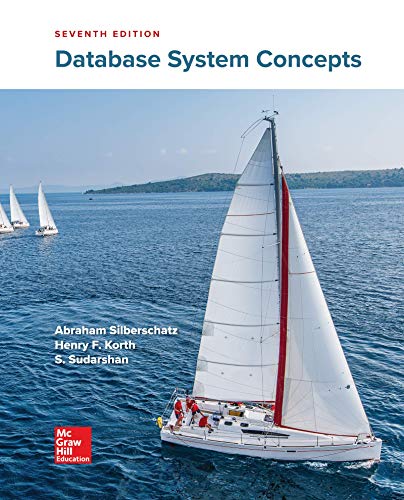
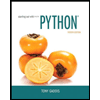
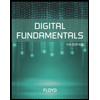
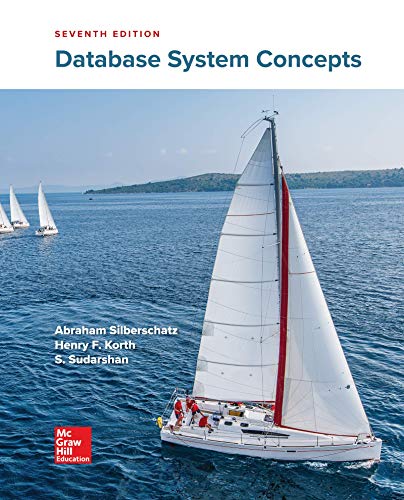
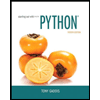
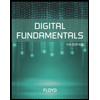
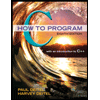
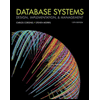
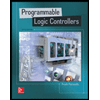