def sum ( lst ): """ Sums a list of numbers .""" if len ( lst ) == 0: return 0 return lst [0] + sum ( lst [1:]) def factorial ( number ): """ Returns the factorial value of any number """ if (( number == 0) or ( number == 1)): return 1 else : return number * factorial ( number - 1) Write down a tail recursive implementation of the function sum in python language. You can use the helper function in your solution. Write down a tail recursive implementation of the function factorial in python language.
def sum ( lst ):
""" Sums a list of numbers ."""
if len ( lst ) == 0:
return 0
return lst [0] + sum ( lst [1:])
def factorial ( number ):
""" Returns the factorial value of any number """
if (( number == 0) or ( number == 1)):
return 1
else :
return number * factorial ( number - 1)
Write down a tail recursive implementation of the function sum in python language. You can use the helper function in your solution. Write down a tail recursive implementation of the function factorial in python language.

def sum(lst):
Tail recursive sum of a list of numbers.
1. Define the `sum` function that takes a list, `lst`, as a parameter.
2. Define a helper function, `tail_recursive_sum`, which takes two parameters:
- `lst`: The list to sum.
- `accumulator`: An accumulator for the running total, initialized to 0.
3. Inside the `tail_recursive_sum` function:
a. Check if the length of the list `lst` is 0.
b. If the list is empty, return the `accumulator` as the result.
c. If the list is not empty:
- Add the first element of the list `lst[0]` to the `accumulator`.
- Call the `tail_recursive_sum` function recursively with the tail of the list `lst[1:]` and the updated `accumulator`.
4. Call the `tail_recursive_sum` function with the input list `lst` and an initial accumulator value of 0.
Step by step
Solved in 5 steps with 4 images

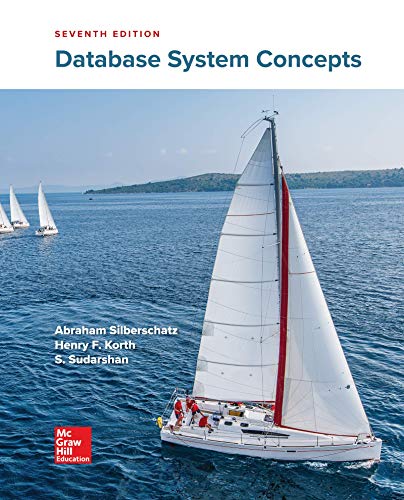
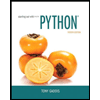
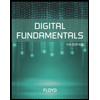
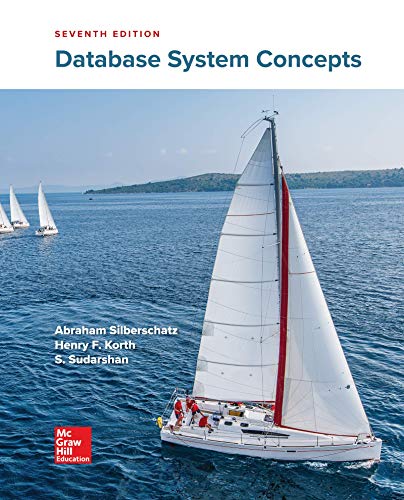
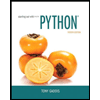
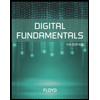
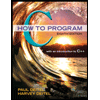
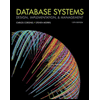
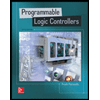