Exercises 1. Copy your code into another cell, and modify to plot the 10th order Taylor series approximation and theoretical max error of f(x) = cos(3x) with expansion point x = 0.5, on the interval [-0.5, 2.5] with h-increment 0.1. NOTES i. '10th order' means that you use up to the 10th derivatives (so the series has 11 terms). ii. Since the expansion point is not zero, in your graph you will need to plot x + h on the x-axis versus the Taylor series value on the y axis. iii. When calculating the theoretical max error, use the fact that the absolute value of the sine function is always less than 1 (same is true for the cosine function). So everywhere that the sine function appears in your remainder expression, you may replace with 1.
### Import section
# 3 necessary statements for numerical plotting in Jupyter
import numpy as np
import matplotlib.pyplot as plt
%matplotlib inline
# Factorial comes from 'math' package
from math import factorial
### Initialization section
#array of derivatives at 0 compute by hand
deriv = np.array([1, 0, -1, 0, 1, 0, -1, 0])
# degree of Taylor polynomial
N=len(deriv)-1
# Max abs. value of N+1 deriv on interval (Compute using calculus)
# (used in the remainder formula)
# @@@ The N+1 derivative will be proportional to cosine, and |cos| is always
# @@@ less than 1
maxAbsNplus1deriv=1
#array with h values
h = np.arange(-3,3.005,0.01)
# Give the expansion point for the Taylor series
expansion=0
# Create an array to contain Taylor series
taylor = np.zeros(len(h))
### Calculation section
#calculate Taylor series for all h at once using a loop
for n in range(N+1):
taylor = taylor + (h**n)/factorial(n)*deriv[n]
#actual function values
actualFn = np.cos(expansion+h)
#error actual and taylor series
actualAbsError = abs(taylor-actualFn)
#theoretical max error=|h^(deg+1)/(deg+1)!*max|n+1 deriv||
theoAbsError = abs(h**(N+1)/factorial(N+1)*maxAbsNplus1deriv)
#Compute actual x values (displaced by expansion point)
xActual = expansion+h
### Output section
#plot figures for cos and errors using object-oriented style
# Two subplots in a row
fig, axes = plt.subplots(nrows=1, ncols=2, figsize=(8,4))
# objects for the left subplot (attached to axes #0)
axes[0].plot(xActual, taylor, 'r',label='Taylor series')
axes[0].plot(xActual, actualFn, 'b--', label='cos(x)')
axes[0].legend(loc=2)#legend in upper left
axes[0].set_title('Taylor Series for cos(x)')
# objects for the right subplot (attached to axes #1)
axes[1].plot(xActual, actualAbsError,label='Actual Absolute Error')
axes[1].plot(xActual, theoAbsError, label='Theoretical Max Absolute Error')
axes[1].legend(loc=2)#legend in upper left
axes[1].set_title('Actual and Theoretical Max Errors')
HERE IS THE CODE FOR THE WARM UP EXCERISE. I NEED HELP WITH EXERCISE 1 PLEASE DO IN PYTHON
![Exercises
1. Copy your code into another cell, and modify to plot the 10th order Taylor series approximation and theoretical max error of f(x) = cos(3x) with
expansion point x = 0.5, on the interval [-0.5, 2.5] with h-increment 0.1.
NOTES
i. '10th order' means that you use up to the 10th derivatives (so the series has 11 terms).
ii. Since the expansion point is not zero, in your graph you will need to plot x + h on the x-axis versus the Taylor series value on the y axis.
iii. When calculating the theoretical max error, use the fact that the absolute value of the sine function is always less than 1 (same is true the cosine
function). So everywhere that the sine function appears in your remainder expression, you may replace with 1.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F892e817a-9b32-4eeb-b8fc-5dd7ffde6479%2Fbc41e69f-d45a-42b2-877d-c08bab51409d%2Fm54tzu_processed.png&w=3840&q=75)
![Taylor Series Python Assignment
The Nth order Taylor series formula is:
h¹
f(x + h)
// ƒ®) (x) + 1/{ ƒ^² (x) + ... -
+
1!
where f(x) denotes the jth derivative of the function f at the point x.
The error in the formula will be less than the Taylor error bound, which is given by:
f(x) +
RN (x + h) =
hN
VIS(N) (x),
N!
|h|N+1
max f(N+¹)(y)].
(N + 1)! y=[x,x+h|
Warm-up exercise:
Complete the following code that computes and plots the 7th order Taylor series approximation and Taylor error bound for f(x) = cos(x) with expansion point
x = 0 on the interval [-3, 3] with increment 0.01.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F892e817a-9b32-4eeb-b8fc-5dd7ffde6479%2Fbc41e69f-d45a-42b2-877d-c08bab51409d%2Fxefje4p_processed.png&w=3840&q=75)

Step by step
Solved in 4 steps with 1 images

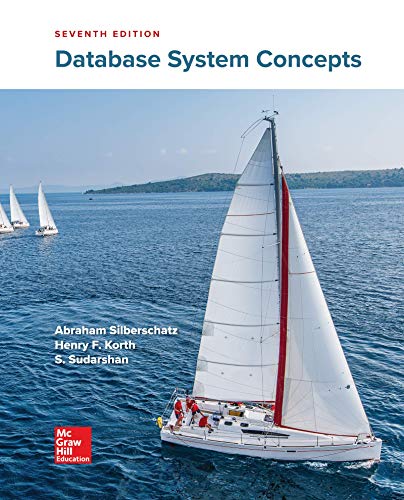
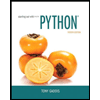
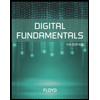
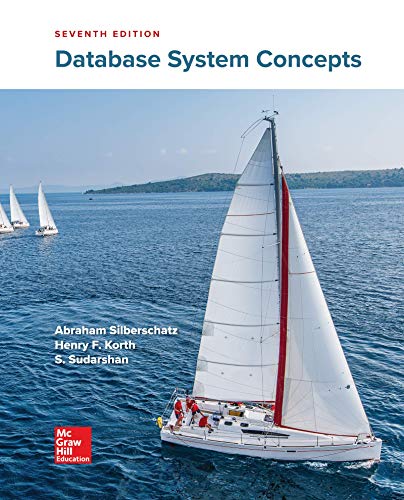
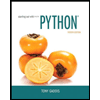
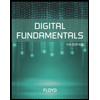
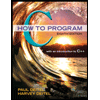
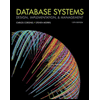
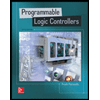