public class Product { private String name; private double cost; public Product(String n, double c) { name=n; cost=c; } public String getName() { return name; } public double getCost() { return cost; } public String toString() { return (name + "$" + cost); } What is the output of the code on the right? } 3 Cereal $7.49 ArrayList cart1 = new ArrayList(); ArrayList cart2 = cart1; cart1.add(new Product("Shampoo",13.89)); cart1.add(new Product("Bread",4.99)); cart1.add(new Product("Cereal",7.49)); System.out.println(cart2.size()); cart2.remove(1); System.out.println(cart1.get(1));
public class Product { private String name; private double cost; public Product(String n, double c) { name=n; cost=c; } public String getName() { return name; } public double getCost() { return cost; } public String toString() { return (name + "$" + cost); } What is the output of the code on the right? } 3 Cereal $7.49 ArrayList cart1 = new ArrayList(); ArrayList cart2 = cart1; cart1.add(new Product("Shampoo",13.89)); cart1.add(new Product("Bread",4.99)); cart1.add(new Product("Cereal",7.49)); System.out.println(cart2.size()); cart2.remove(1); System.out.println(cart1.get(1));
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Concept explainers
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Question
can you give me a background explanation i can use to approach this problem?

Transcribed Image Text:public class Product {
private String name;
private double cost;
public Product(String n, double c) {
name=n;
cost=c;
}
public String getName() {
return name;
}
public double getCost() {
return cost;
What is the output of the code on the right? I
}
public String toString() {
return (name + "$" + cost);
}
3
Cereal $7.49
ArrayList<Product> cart1 = new ArrayList<Product>();
ArrayList<Product> cart2 = cart1;
cart1.add(new Product("Shampoo", 13.89));
cart1.add(new Product("Bread",4.99));
cart1.add(new Product("Cereal",7.49));
System.out.println(cart2.size());
cart2.remove(1);
System.out.println(cart1.get(1));
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
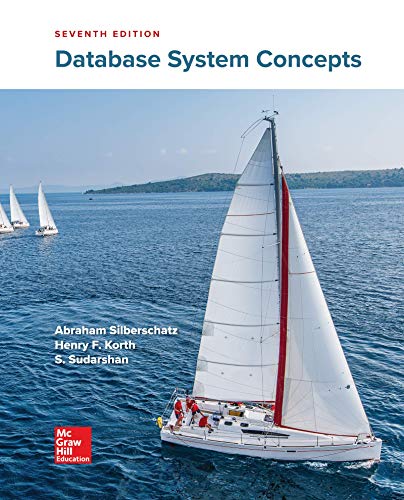
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
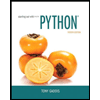
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
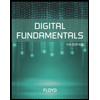
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
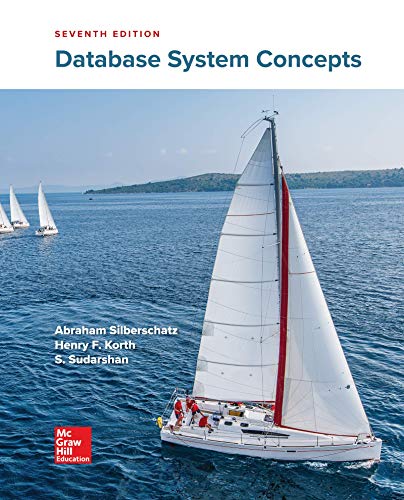
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
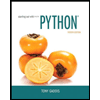
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
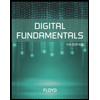
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
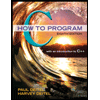
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
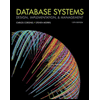
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
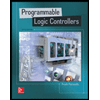
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education