Could you please assist me with this problem? Thank you.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
100%
Could you please assist me with this problem?
Thank you.

Transcribed Image Text:### Module 2.7.1: Enter the Output of Modules
This section focuses on understanding the output of Python modules using functions across different files (`main.py`, `first.py`, and `second.py`).
#### `main.py`
```python
import first
def one(number):
return number ** 2
def two(number):
return number * 8
def three(number):
return one(number) - two(number)
print(three(2))
print(first.three(2))
print(first.four(2))
```
#### `first.py`
```python
import second
def one(number):
return number - 1
def two(number):
return number + 9
def three(number):
return one(number) * two(number)
def four(number):
return second.three(number)
```
#### `second.py`
```python
def one(number):
return number * 4
def two(number):
return number - 5
def three(number):
return number + 6
```
### Explanation
- **main.py**: The program defines three functions:
- `one(number)` calculates the square of `number`.
- `two(number)` multiplies `number` by 8.
- `three(number)` subtracts the result of `two(number)` from `one(number)`.
- It prints the result of calling `three(2)`, `first.three(2)`, and `first.four(2)`.
- **first.py**: The program imports `second.py` and defines four functions:
- `one(number)` subtracts 1 from `number`.
- `two(number)` adds 9 to `number`.
- `three(number)` multiplies `one(number)` and `two(number)`.
- `four(number)` calls `second.three(number)` and returns the result.
- **second.py**: The program defines three functions:
- `one(number)` multiplies `number` by 4.
- `two(number)` subtracts 5 from `number`.
- `three(number)` adds 6 to `number`.
This script helps in understanding the flow of data and function calls between different modules in a Python program.
Expert Solution

Question 1
In the above program, in the "first" module, the "second" module has been imported.
In the "main" module, the "first" module has been imported.
The function call of any module will be specified with the module name in the beginning.
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
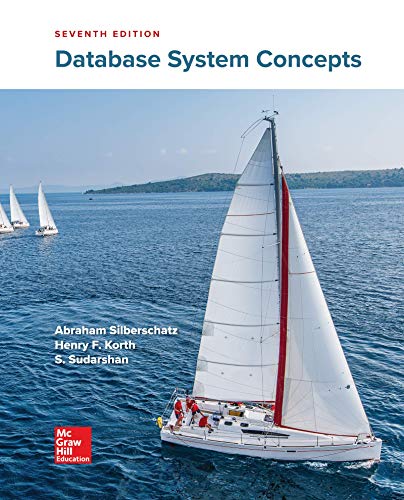
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
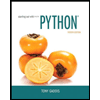
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
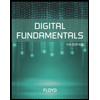
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
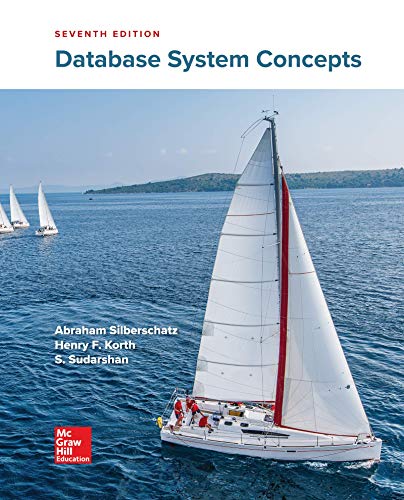
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
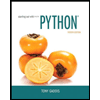
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
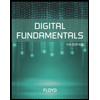
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
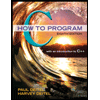
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
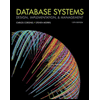
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
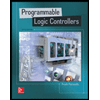
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education