Program 1 Two files are required, a data class named Book and an executable class named TestBook. Class Book has instance data members (all private) String title, String author, int pages, double price. has a public static int variable named numBooks with an initial value of zero. has a parameterized constructor that will be used to make a Book object and assign values to its data members, and increment numBooks. has a no-arg constructor that increments numBooks. has getters and setters for all instance data members. has a toString() method that returns a string displaying the state of a Book instance. Use the numBooks variable to report the number of books instantiated. Class TestBook This class needs a main method and two more methods.
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Hello! I need help with the first problem, here are the requirements!
Please use Eclipse if possible! and showing a screenshot of the output would also be helpful. Also comments displaying what the code is doing is also very helpful so I understand it. I am asking this question for a second time because the last person did not even post the right answer :( like it was totally no related at all. Thank you so much!!!
Assignment Requirements
- Create a new Java Project in your Eclipse workspace named as before, smith3 or jones3 for example.
- Create a package with the very same name as your project name.
- In this one package, write one Java
program for each exercise as required below. - Choose descriptive variable names and identifiers in all programs
Program 1
Two files are required, a data class named Book and an executable class named TestBook.
Class Book
- has instance data members (all private) String title, String author, int pages, double price.
- has a public static int variable named numBooks with an initial value of zero.
- has a parameterized constructor that will be used to make a Book object and assign values to its data members, and increment numBooks.
- has a no-arg constructor that increments numBooks.
- has getters and setters for all instance data members.
- has a toString() method that returns a string displaying the state of a Book instance.
- Use the numBooks variable to report the number of books instantiated.
Class TestBook
This class needs a main method and two more methods.
In main:
- create an array capable of holding six Book objects.
- use the parameterized constructor to specify the data in the first four elements of this array
- use the no-arg constructor to create the two remaining books in the array.
- process the array with a foreach loop to display the array at this point.
- call the finishArray() method with the array as the only argument.
- call the reduceBooks() method with the array as the sole argument.
- repeat the code needed by Step 4 above.
- display the most expensive book after the discounts.
In finishArray():
- this is a void method.
- use the setter methods to specify the data in all fields of the last two books in the array.
In reduceBooks():
- this method returns a Book instance.
- use a loop (any type) to reduce the price of every book in the array by 40%.
- determine the most expensive book after the discounts and return this book to main.
I will attach the sample output:
Thank you so much for the help!!


Trending now
This is a popular solution!
Step by step
Solved in 7 steps with 5 images

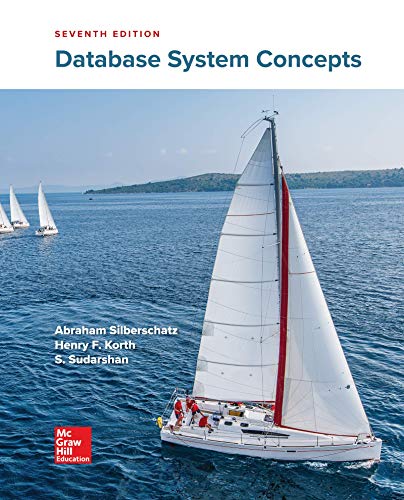
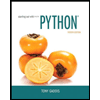
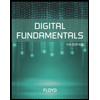
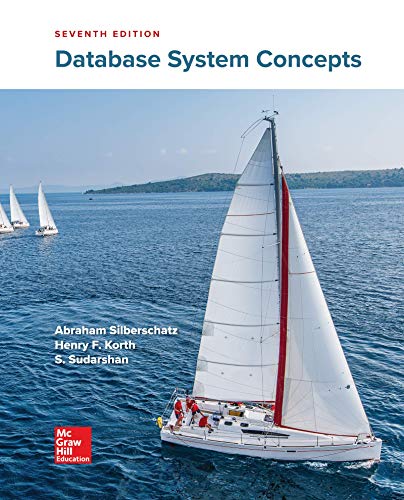
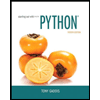
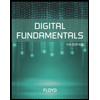
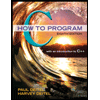
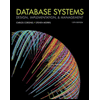
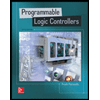