POEM_LINE = str POEM = List[POEM_LINE] PHONEMES = Tuple[str] PRONUNCIATION_DICT = Dict[str, PHONEMES] def all_lines_rhyme(poem_lines: POEM, lines_to_check: List[int], word_to_phonemes: PRONUNCIATION_DICT) -> bool: """Return True if and only if the lines from poem_lines with index in lines_to_check all rhyme, according to word_to_phonemes. Precondition: lines_to_check != [] >>> poem_lines = ['The mouse', 'in my house', 'electric.'] >>> lines_to_check = [0, 1] >>> word_to_phonemes = {'THE': ('DH', 'AH0'), ... 'MOUSE': ('M', 'AW1', 'S'), ... 'IN': ('IH0', 'N'), ... 'MY': ('M', 'AY1'), ... 'HOUSE': ('HH', 'AW1', 'S'), ... 'ELECTRIC': ('IH0', 'L', 'EH1', 'K', ... 'T', 'R', 'IH0', 'K')} >>> all_lines_rhyme(poem_lines, lines_to_check, word_to_phonemes) True >>> lines_to_check = [0, 1, 2] >>> all_lines_rhyme(poem_lines, lines_to_check, word_to_phonemes) False >>> lines_to_check = [2] >>> all_lines_rhyme
POEM_LINE = str
POEM = List[POEM_LINE]
PHONEMES = Tuple[str]
PRONUNCIATION_DICT = Dict[str, PHONEMES]
def all_lines_rhyme(poem_lines: POEM, lines_to_check: List[int],
word_to_phonemes: PRONUNCIATION_DICT) -> bool:
"""Return True if and only if the lines from poem_lines with index in
lines_to_check all rhyme, according to word_to_phonemes.
Precondition: lines_to_check != []
>>> poem_lines = ['The mouse', 'in my house', 'electric.']
>>> lines_to_check = [0, 1]
>>> word_to_phonemes = {'THE': ('DH', 'AH0'),
... 'MOUSE': ('M', 'AW1', 'S'),
... 'IN': ('IH0', 'N'),
... 'MY': ('M', 'AY1'),
... 'HOUSE': ('HH', 'AW1', 'S'),
... 'ELECTRIC': ('IH0', 'L', 'EH1', 'K',
... 'T', 'R', 'IH0', 'K')}
>>> all_lines_rhyme(poem_lines, lines_to_check, word_to_phonemes)
True
>>> lines_to_check = [0, 1, 2]
>>> all_lines_rhyme(poem_lines, lines_to_check, word_to_phonemes)
False
>>> lines_to_check = [2]
>>> all_lines_rhyme(poem_lines, lines_to_check, word_to_phonemes)
True
"""

Step by step
Solved in 4 steps with 2 images

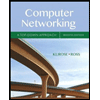
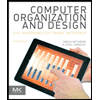
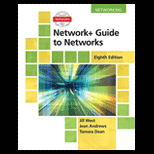
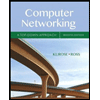
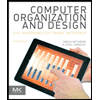
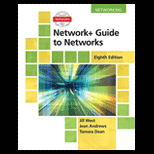
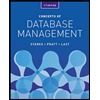
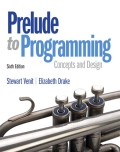
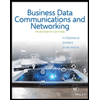