Please explain this Arduino code int redPin=11; //set red LED pin to 11 int bluePin=6; //set blue LED pin to 6 int brightness=255; //Set brightness to 255 String colorChoice; //Will hold users input of color choice void setup() { // put your setup code here, to run once: Serial.begin(9600); //Turn on Serial port pinMode(redPin, OUTPUT); //Set redPin to be an output pinMode(bluePin, OUTPUT); //set bluePin to be an output } void loop() { Serial.println("What color would you like the LED? (red, green, or blue)"); //Prompt user for color while (Serial.available()==0) { } //Wait for input colorChoice = Serial.readString(); if (colorChoice=="red") { analogWrite(redPin, brightness); //turn on red pin analogWrite(greenPin, 0); //turn off green pin analogWrite(bluePin, 0); //write off blue pin } if (colorChoice=="blue") { analogWrite(redPin, 0); //turn off red pin analogWrite(greenPin, 0); //turn off green pin analogWrite(bluePin, brightness); //write on blue pin } if (colorChoice!="red" && colorChoice != "blue") { Serial.println("That is not a valid color choice, please try again"); Serial.println(""); } }
Please explain this Arduino code
int redPin=11; //set red LED pin to 11
int bluePin=6; //set blue LED pin to 6
int brightness=255; //Set brightness to 255
String colorChoice; //Will hold users input of color choice
void setup() {
// put your setup code here, to run once:
Serial.begin(9600); //Turn on Serial port
pinMode(redPin, OUTPUT); //Set redPin to be an output
pinMode(bluePin, OUTPUT); //set bluePin to be an output
}
void loop() {
Serial.println("What color would you like the LED? (red, green, or blue)"); //Prompt user for color
while (Serial.available()==0) { } //Wait for input
colorChoice = Serial.readString();
if (colorChoice=="red") {
analogWrite(redPin, brightness); //turn on red pin
analogWrite(greenPin, 0); //turn off green pin
analogWrite(bluePin, 0); //write off blue pin
}
if (colorChoice=="blue") {
analogWrite(redPin, 0); //turn off red pin
analogWrite(greenPin, 0); //turn off green pin
analogWrite(bluePin, brightness); //write on blue pin
}
if (colorChoice!="red" && colorChoice != "blue") {
Serial.println("That is not a valid color choice, please try again");
Serial.println("");
}
}

Step by step
Solved in 2 steps with 2 images

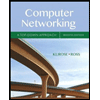
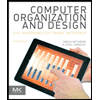
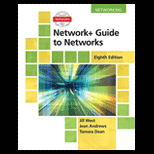
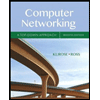
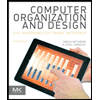
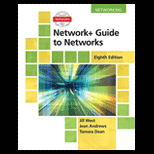
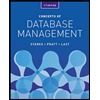
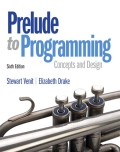
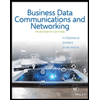