Write the program that allows the user to sort using the Bubble Sort, Selection Sort, Insertion Sort and Shell Short The program should be able to read in data from a binary file. The first element of the binary file will be used to tell how many elements to read in. Once all the data has been read in, the program should sort the data. The user should be able to choose which algorithm to use to sort the data. The program should print the time before and after the sort. The last part of the program should prompt the user for a lower and upper bound. These two value should then be used to decide how much and which part of the array will be display. You should test all of the sorts on each of the data files. There are a number of files (10numbers.zip, etc.) of increasing size that you can utilize for testing purposes.
Using these codes as guide
BinaryFileRead.cpp
// BinaryFileRead.cpp : Defines the entry point for the console application. //
#include "stdafx.h"
#include <iostream>
using namespace std;
int main()
{
int *arrayToSort;
char fileName[50];
int size,readVal;
cout << "Enter a filename to sort => ";
cin >> fileName;
FILE *inFile;
fopen_s(&inFile,fileName, "rb");
fread(&size, sizeof(size), 1, inFile);
arrayToSort = new int[size];
for (int i = 0; i < size; i++) {
fread(&readVal, sizeof(readVal), 1, inFile);
arrayToSort[i] = readVal;
}
fclose(inFile);
return 0;
}
Timing.cpp
// Timing.cpp : Defines the entry point for the console application. //
#include "stdafx.h"
#include <iostream>
#include <time.h> //ctime
#include <sys/timeb.h> //_timeb _ftime_s
using namespace std;
int main()
{
struct _timeb timebuffer;
char timeline[26];
_ftime_s(&timebuffer);
ctime_s(timeline,sizeof(timeline), &(timebuffer.time));
printf("The time is %.19s.%hu %s", timeline, timebuffer.millitm, &timeline[20]);
system("pause");
return 0;
}
Insertion Sort:

Introduction to Binary File Sorting Program
The Binary File Sorting Program is a C++ application designed to efficiently read data from a binary file, apply various sorting algorithms to organize the data, and allow the user to specify a range of elements to display from the sorted array. The program provides a comprehensive tool for evaluating and comparing the performance of different sorting algorithms while managing binary file data.
Key Features of the Program:
1. Binary File Input: The program begins by requesting the user to provide the name of a binary file. It opens this file and retrieves the size of the data array, determining how many elements to read.
2. Dynamic Array Allocation: To store the data from the binary file, the program dynamically allocates an integer array of the appropriate size.
3. Sorting Algorithms: The program offers four sorting algorithms for organizing the data: Bubble Sort, Selection Sort, Insertion Sort, and Shell Sort. These sorting methods are implemented as separate functions, making it easy to compare their performance.
4. Execution Time Measurement: Before and after the sorting process, the program measures the execution time using the `clock` function. This provides a quantifiable measure of each sorting algorithm's efficiency.
5. User Interaction: Users are prompted to input lower and upper bounds, specifying the range of sorted elements they want to display. The program then presents the sorted elements within the specified range.
6. Memory Cleanup: After sorting and displaying the data, the program takes care of deallocating the memory used by the dynamically allocated array, preventing memory leaks.
7. Algorithm Analysis: The program encourages users to provide a write-up for each sorting algorithm, describing how they perform on various data scenarios (e.g., random and sorted data). Users are also expected to discuss the "Big O" time complexity of each algorithm, facilitating a comparative analysis of their efficiency.
In summary, this Binary File Sorting Program is a versatile utility that simplifies the process of sorting data from binary files and offers insights into the relative performance of different sorting algorithms. It can be a valuable tool for students and professionals studying algorithm efficiency and data sorting applications.
Trending now
This is a popular solution!
Step by step
Solved in 3 steps

for the timing.
can you do similar to this:
Timing.cpp
// Timing.cpp : Defines the entry point for the console application. //
#include "stdafx.h"
#include <iostream>
#include <time.h> //ctime
#include <sys/timeb.h> //_timeb _ftime_s
using namespace std;
int main()
{
struct _timeb timebuffer;
char timeline[26];
_ftime_s(&timebuffer);
ctime_s(timeline,sizeof(timeline), &(timebuffer.time));
printf("The time is %.19s.%hu %s", timeline, timebuffer.millitm, &timeline[20]);
system("pause");
return 0;
}
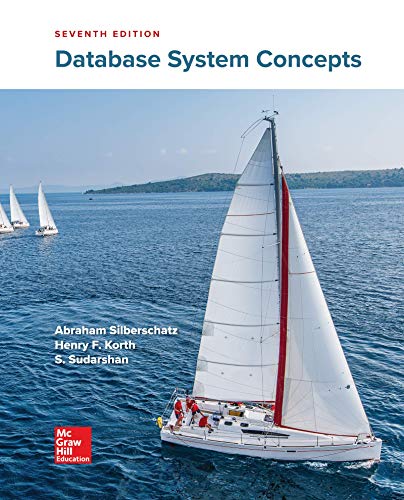
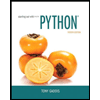
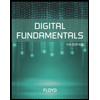
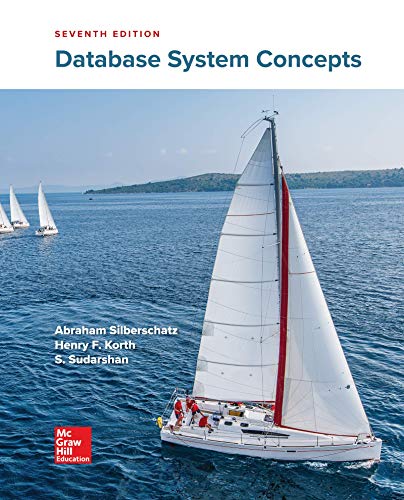
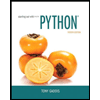
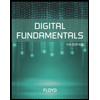
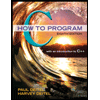
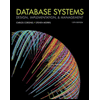
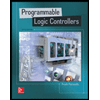