Part 1: Design and implement a class called Sphere that contains instance data that represents the sphere's diameter. The Sphere also includes the following: • A constructor to accept and initialize the diameter, • A default constructor to initialize sphere's diameter to 1, • A copy constructor • getter and setter methods for the diameter • Methods that o calculate and return the volume and surface area of the sphere o A toString method that returns a one-line description of the sphere to include diameter, Surface Area and Volume o An equals method to compare diameters of the spheres and if they are the same the spheres are identical otherwise not. Needed formulas: • volume =, n where r Radius and Radius = Diameter / 2 %3D • Surface area = 4 nr Where r = Radius art 2: eate a driver class called MultiSphere, whose main method instantiates and updates several Sphere objects and prints them to the nitor. the toolhar press ALT+E10(PC)or ALT+EN+E10 (Mac) ave and Submit to save and submit. Click Save All Answers to save all answers.
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:



public class Sphere {
//a Diameter define
double diameter;
//define a constructor to define sphere object
Sphere(double d) {
diameter = d;
}
// a getter method to get the Sphere diameter
double getDiameter() {
return diameter;
}
// Volume: formula is 4/3 Pi * R3
double getVolume() {
double radius = diameter / 2.0;
double volume = 4.0 / 3.0 * Math.PI * radius * radius * radius;
return volume;
}
// Surface: formula is 4 Pi * R2
double getSurface() {
double radius = diameter / 2.0;
double surface = 4.0 * Math.PI * radius * radius;
return surface;
}
public static void main(String[] arg) {
//2 sphere objects by "instantiating" the class Sphere
Sphere sphere1 = new Sphere(1.0);
Sphere sphere2 = new Sphere(2.0);
// display the surface and the volume
System.out.println("Sphere 1: Diameter: " + sphere1.getDiameter() + " Surface: " + sphere1.getSurface() + " Volume: " + sphere1.getVolume());
System.out.println("Sphere 2: Diameter: " + sphere2.getDiameter() + " Surface: " + sphere2.getSurface() + " Volume: " + sphere2.getVolume());
}
}
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

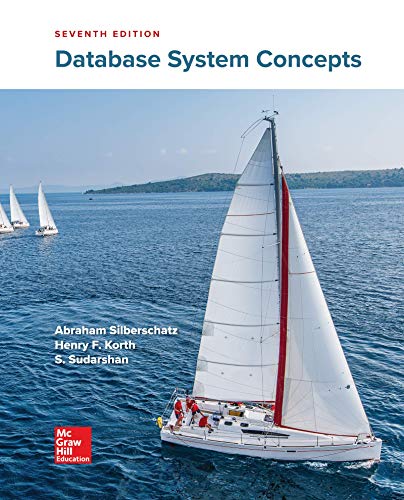
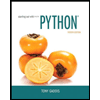
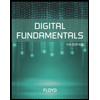
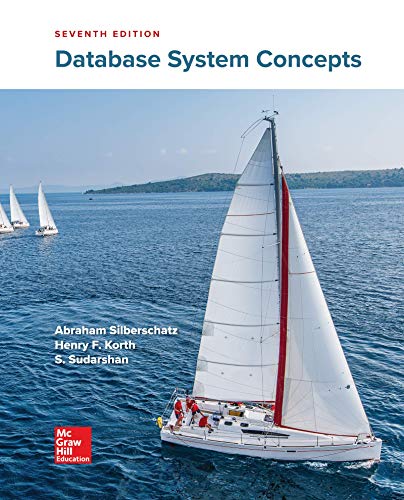
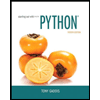
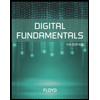
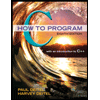
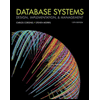
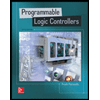