Overload the [] operator to return the ith digit in the biguint (use the array indexing; so if they do [0] you will give the digit in the ones place). Call [] from main; use it to see whether the constructors worked. Overload << as a non-member function. It’s fine to print out the leading 0s. Call it from main. Overload += as a member function. Call it from main
Overload the [] operator to return the ith digit in the biguint (use the array indexing; so if they do [0] you will give the digit in the ones place). Call [] from main; use it to see whether the constructors worked. Overload << as a non-member function. It’s fine to print out the leading 0s. Call it from main. Overload += as a member function. Call it from main
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Concept explainers
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Question
Initial code is attatched below.
Questions:
- Overload the [] operator to return the ith digit in the biguint (use the array indexing; so if they do [0] you will give the digit in the ones place). Call [] from main; use it to see whether the constructors worked.
- Overload << as a non-member function. It’s fine to print out the leading 0s. Call it from main.
- Overload += as a member function. Call it from main.
![#include <cstdlib>
#include <iostream>
#include <string>
// WANT: integers with CAPACITY digits, only non-negative
//
// support:
//
2 constructors: int, string
//
member functions: [] returns individual digits given position
//
+=
//
--
//
compare: return +1, 0, -1, depending on
whether this biguint >, =-, < than given biguint
- (binary), - (unary), <, <=, ==, !=, >=, >
//
//
+,
//
<<, >>
class biguint
{
public:
// CONSTANTS & TYPES
static const std::size_t CAPACITY = 20;
// CONSTRUCTORS
// pre: none
// post: creates a biguint with value 0
biguint ();
// pre: s contains only decimal digits
// post: creates a biguint whose value corresponds to given string of digits
biguint (const std::string &);
// CONSTANT MEMBER FUNCTIONS
// pre: pos < CAPACITY
// post: returns the digit at position pos
//
O is the least significant (units) position
unsigned short operator [](std::size_t pos) const;
// pre: none
// post: returns 1 if this biguint > b
//
O if this biguint -- b
-1 if this biguint < b
//
int compare (const biguint & b) const;
// MODIFICATION MEMBER FUNCTIONS
// pre: none
// post: b is added to this biguint; ignore last carry bit if any
void operator += (const biguint & b);
void operator -- (const biguint & b);
private:
unsigned short data_[CAPACITY];
// INVARIANTS:
//
data [i] holds the i^th digit of this biguint or 0 if not used
//
data_[0] holds the least significant (units) digit
} ;
// nonmember functions
biguint operator + (const biguint &, const biguint &);
biguint operator - (const biguint &, const biguint &);
bool operator < (const biguint &, const biguint &);
bool operator <- (const biguint &, const biguint &);
bool operator !- (const biguint &, const biguint &);
bool operator =- (const biguint &, const biguint &);
bool operator >= (const biguint &, const biguint &);
bool operator > (const biguint &, const biguint &);
std::ostream& operator <<(std::ostream&, const biguint& ) ;](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fbf5ffd78-7e14-4c4d-bb22-c53272263ab3%2F607e1d47-efdc-415b-a905-689bb704a593%2Fubh23uo_processed.png&w=3840&q=75)
Transcribed Image Text:#include <cstdlib>
#include <iostream>
#include <string>
// WANT: integers with CAPACITY digits, only non-negative
//
// support:
//
2 constructors: int, string
//
member functions: [] returns individual digits given position
//
+=
//
--
//
compare: return +1, 0, -1, depending on
whether this biguint >, =-, < than given biguint
- (binary), - (unary), <, <=, ==, !=, >=, >
//
//
+,
//
<<, >>
class biguint
{
public:
// CONSTANTS & TYPES
static const std::size_t CAPACITY = 20;
// CONSTRUCTORS
// pre: none
// post: creates a biguint with value 0
biguint ();
// pre: s contains only decimal digits
// post: creates a biguint whose value corresponds to given string of digits
biguint (const std::string &);
// CONSTANT MEMBER FUNCTIONS
// pre: pos < CAPACITY
// post: returns the digit at position pos
//
O is the least significant (units) position
unsigned short operator [](std::size_t pos) const;
// pre: none
// post: returns 1 if this biguint > b
//
O if this biguint -- b
-1 if this biguint < b
//
int compare (const biguint & b) const;
// MODIFICATION MEMBER FUNCTIONS
// pre: none
// post: b is added to this biguint; ignore last carry bit if any
void operator += (const biguint & b);
void operator -- (const biguint & b);
private:
unsigned short data_[CAPACITY];
// INVARIANTS:
//
data [i] holds the i^th digit of this biguint or 0 if not used
//
data_[0] holds the least significant (units) digit
} ;
// nonmember functions
biguint operator + (const biguint &, const biguint &);
biguint operator - (const biguint &, const biguint &);
bool operator < (const biguint &, const biguint &);
bool operator <- (const biguint &, const biguint &);
bool operator !- (const biguint &, const biguint &);
bool operator =- (const biguint &, const biguint &);
bool operator >= (const biguint &, const biguint &);
bool operator > (const biguint &, const biguint &);
std::ostream& operator <<(std::ostream&, const biguint& ) ;
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
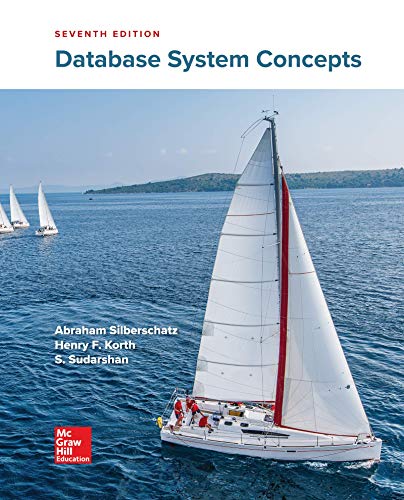
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
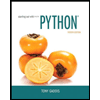
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
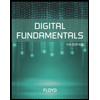
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
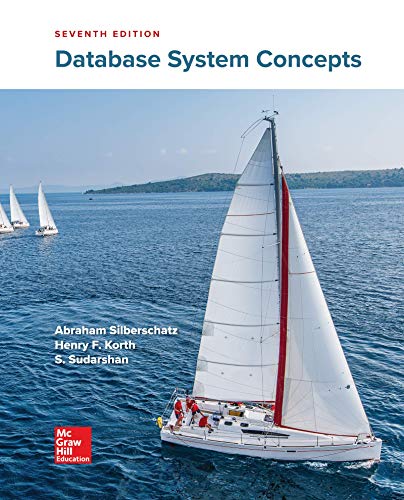
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
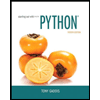
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
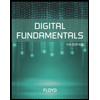
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
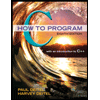
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
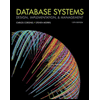
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
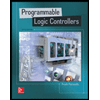
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education