Now implement the removeOdd method in Java. Can only edit code in the /* Your code goes here */ portion.
Now implement the removeOdd method in Java. Can only edit code in the /* Your code goes here */ portion.
Chapter11: Advanced Inheritance Concepts
Section: Chapter Questions
Problem 10PE
Related questions
Question
Now implement the removeOdd method in Java.
Can only edit code in the /* Your code goes here */ portion.
![0 src e RemoveTester
m main
roject
C PrintDemo.java X
e PasswordGenerator.java x
G
IntegerName.java x
C Grades.java
test.java x
C ArrayDemo,java x
x
© Num
cSC 1350 -/ldeaProjects/CSC 1350
import java.util.Arrays;
1
.idea
2
out
3 >
public class RemoveTester
src
C ArrayDemo
C Boxes
e Compare2
e Cubes
e DoLoop
© Doublelnvestment2
C DuplicatedCharacters
4
{
public static int removeodd (int[] values, int size)
6
/* Your code here */
8
public static void main(String[] args)
10
EarthquakeStrength
11
int(] a = { 22, 98, 95, 46, 31, 53, 82, 24, 11, 19 };
int sizeBefore = 8;
int sizeAfter = removeodd(a, sizeBefore);
System.out.print ("a: [ ");
for (int i = 0; i< sizeAfter; i++)
ElevatorSimulation2
12
Error1
13
C Error2
C Error3
© FindSpaces
FirstVowel.java
e Geometry
C Grades
HelloPrinter
eIntegerName
InvestmentTable
ljoh661.zip
© Numbers
e PasswordGenerator
PowerTable
14
15
16
17
System.out.print(a[i] +" ");
18
I }
19
System.out.println("]");
20
System.out.println("Expected: [ 22 98 46 82 24 ]");
21
22
int[] b = { 23, 97, 95, 45, 31, 53, 81, 24, 11, 19 };
23
sizeBefore = 7;
24
sizeAfter = removeodd(b, sizeBefore);
25
System.out.print("b: [ ");
for (int i = 0; i< sizeAfter; i++)
PrintDemo
e PrintTableDemo
C Prog06_PartyPooper
e Pyramids
G RandomDemo
C ReadTime
e ReciprocalSum
26
27
28
System.out.print(b[i] + " ");
29
}
30
System.out.println("]");
31
System.out.println("Expected: [ ]");
RemoveDuplicateWords
G RemoveTester
C Reverse
e SentinelDemo
e Shipping
G TaxCalculator
e test
e UniqueChars
32
33
e Volume1
csC 1350.im!
> l External Libraries
o Scratches and Consoles
E TODO
O Problems
A Build
|Terminal
MacBook Pro
esc
O Reverse
@
#3
$
&
1
4](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fd6e87594-a0d7-48c0-af39-cad58e8108aa%2Ff1a72ae9-c76e-4d20-b2cc-34a7d1a7e18b%2F0fq2qhu_processed.jpeg&w=3840&q=75)
Transcribed Image Text:0 src e RemoveTester
m main
roject
C PrintDemo.java X
e PasswordGenerator.java x
G
IntegerName.java x
C Grades.java
test.java x
C ArrayDemo,java x
x
© Num
cSC 1350 -/ldeaProjects/CSC 1350
import java.util.Arrays;
1
.idea
2
out
3 >
public class RemoveTester
src
C ArrayDemo
C Boxes
e Compare2
e Cubes
e DoLoop
© Doublelnvestment2
C DuplicatedCharacters
4
{
public static int removeodd (int[] values, int size)
6
/* Your code here */
8
public static void main(String[] args)
10
EarthquakeStrength
11
int(] a = { 22, 98, 95, 46, 31, 53, 82, 24, 11, 19 };
int sizeBefore = 8;
int sizeAfter = removeodd(a, sizeBefore);
System.out.print ("a: [ ");
for (int i = 0; i< sizeAfter; i++)
ElevatorSimulation2
12
Error1
13
C Error2
C Error3
© FindSpaces
FirstVowel.java
e Geometry
C Grades
HelloPrinter
eIntegerName
InvestmentTable
ljoh661.zip
© Numbers
e PasswordGenerator
PowerTable
14
15
16
17
System.out.print(a[i] +" ");
18
I }
19
System.out.println("]");
20
System.out.println("Expected: [ 22 98 46 82 24 ]");
21
22
int[] b = { 23, 97, 95, 45, 31, 53, 81, 24, 11, 19 };
23
sizeBefore = 7;
24
sizeAfter = removeodd(b, sizeBefore);
25
System.out.print("b: [ ");
for (int i = 0; i< sizeAfter; i++)
PrintDemo
e PrintTableDemo
C Prog06_PartyPooper
e Pyramids
G RandomDemo
C ReadTime
e ReciprocalSum
26
27
28
System.out.print(b[i] + " ");
29
}
30
System.out.println("]");
31
System.out.println("Expected: [ ]");
RemoveDuplicateWords
G RemoveTester
C Reverse
e SentinelDemo
e Shipping
G TaxCalculator
e test
e UniqueChars
32
33
e Volume1
csC 1350.im!
> l External Libraries
o Scratches and Consoles
E TODO
O Problems
A Build
|Terminal
MacBook Pro
esc
O Reverse
@
#3
$
&
1
4
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
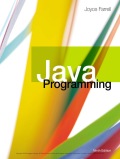
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
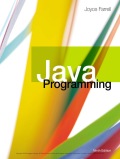
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT