This question is in java The Sorts.java file is the sorting program we looked at . You can use either method listed in the example when coding. You want to add to the grades.java and calculations.java files so you put the list in numerical order. Write methods to find the median and the range. Have a toString method that prints the ordered list, the number of items in the list, the mean, median and range. Ex List: 20 , 30 , 40 , 75 , 93 Number of elements: 5 Mean: 51.60 Median: 40 Range: 73 Grades.java is the main file import java.util.Scanner; public class grades { public static void main(String[] args) { int n; calculation c = new calculation(); int array[] = new int[20]; System.out.print("Enter number of grades that are to be entered: "); Scanner scan = new Scanner(System.in); n = scan.nextInt(); System.out.println("Enter the grades: "); for(int i=0; i 0 && numbers[position-1] > key) { numbers[position] = numbers[position-1]; position--; } numbers[position] = key; } } }
This question is in java
The Sorts.java file is the sorting program we looked at . You can use either method listed in the example when coding.
You want to add to the grades.java and calculations.java files so you put the list in numerical order. Write methods to find the median and the range.
Have a toString method that prints the ordered list, the number of items in the list, the mean, median and range.
Ex
List: 20 , 30 , 40 , 75 , 93
Number of elements: 5
Mean: 51.60
Median: 40
Range: 73
Grades.java is the main file
import java.util.Scanner;
public class grades
{
public static void main(String[] args)
{
int n;
calculation c = new calculation();
int array[] = new int[20];
System.out.print("Enter number of grades that are to be entered: ");
Scanner scan = new Scanner(System.in);
n = scan.nextInt();
System.out.println("Enter the grades: ");
for(int i=0; i<n; i++)
{
array[i] = scan.nextInt();
if(array[i] < 0)
{
System.out.println("Invalid: enter again");
i--;
}
}
System.out.println("\nThe average of your " +n+" grades is "+c.average(array, n));
}
}
calculations.java is used to find the average
import java.util.*;
class calculation
{
double average(int[] arr, int n)
{
double av;
int sum=0;
for(int i=0; i<n; i++)
{
sum = sum + arr[i];
}
av = (double)sum / (double) n;
return av;
}
}
sorts.java is an example file, in which the question asks to use either method from this code.
//********************************************************************
// Sorts.java Author: Lewis/Loftus/Cocking
//
// Demonstrates the selection sort and insertion sort algorithms,
// as well as a generic object sort.
//********************************************************************
public class Sorts
{
//-----------------------------------------------------------------
// Sorts the specified array of integers using the selection
// sort
//-----------------------------------------------------------------
public static void selectionSort (int[] numbers)
{
int min, temp;
for (int index = 0; index < numbers.length-1; index++)
{
min = index;
for (int scan = index+1; scan < numbers.length; scan++)
if (numbers[scan] < numbers[min])
min = scan;
// Swap the values
temp = numbers[min];
numbers[min] = numbers[index];
numbers[index] = temp;
}
}
//-----------------------------------------------------------------
// Sorts the specified array of integers using the insertion
// sort algorithm.
//-----------------------------------------------------------------
public static void insertionSort (int[] numbers)
{
for (int index = 1; index < numbers.length; index++)
{
int key = numbers[index];
int position = index;
// shift larger values to the right
while (position > 0 && numbers[position-1] > key)
{
numbers[position] = numbers[position-1];
position--;
}
numbers[position] = key;
}
}
}

Trending now
This is a popular solution!
Step by step
Solved in 6 steps with 3 images

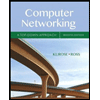
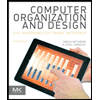
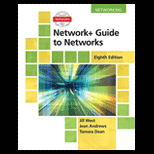
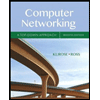
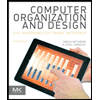
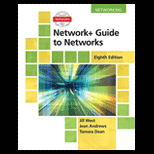
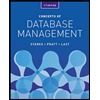
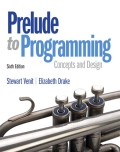
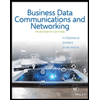