need help on my c++ code i dont know how to properly use " tokennize " #include #include #include #include "Date.h" #include "Person.h" #include "Student.h" #include "Faculty.h" using namespace std; void addStudent(vector& students); void addFaculty(vector& faculty); void ListCourse(string fName, vectorstudents, vectorfaculty); void tokenizeDate(char* cDate, int& month, int& day, int& year); char menu(); int main() { //vectors for storage vectorstudents; vectorfaculty; string courseName; //name of course cout << "Enter a name for your course: "; getline(cin, courseName); cout << endl; // getmenu char ch = menu(); //Loop repeat until quit while (ch !='Q') { if (ch=='I'){ addFaculty (faculty); } else if (ch =='S'){ addStudent(students); } else if (ch=='L'){ ListCourse(courseName, students, faculty); } // loop repetition cout<>ch; return toupper(ch); } void addStudent() //example function that uses tokenizeDate { Date m, d, y; char charDate[20]; //holds the date the user entered in char array int pID; Date bDate; Date sEnrolled; string fName, lName, sMajor, sLevel; double sGPA; Date realDate; //date object; holds date after tokenization cout << "\nEnter a date (mm/dd/yyyy): "; cin >> charDate; cout<<"ID\n:"; cin>>pID; cout<<"\nFirst Name:"; cin>>fName; cout<<"\nLast Name:"; cin>>lName; cout<<"\nMajor:"; cin>>sMajor; cout<<"\nLevel (Freshman, Sophmore, Junior, Senior):"; cin>>sLevel; cout<<"\nGPA:"; cin>>sGPA; } tokenizeDate(Date, m, d, y); Date.setDate(m,d, y); } //Split date into day,month and date void tokenizeDate(char* cDate, int& month, int& day, int& year) { char seps[] = "/"; char* token = NULL; char* next_token = NULL; token = NULL; next_token = NULL; // Establish string and get the tokens: token = strtok_s(cDate, seps, &next_token); month = atoi(token); token = strtok_s(NULL, seps, &next_token); day = atoi(token); token = strtok_s(NULL, seps, &next_token); year = atoi(token); }
I need help on my c++ code
i dont know how to properly use " tokennize "
#include <iostream>
#include <vector>
#include <algorithm>
#include "Date.h"
#include "Person.h"
#include "Student.h"
#include "Faculty.h"
using namespace std;
void addStudent(vector<Student>& students);
void addFaculty(vector<Faculty>& faculty);
void ListCourse(string fName, vector<Student>students, vector<Faculty>faculty);
void tokenizeDate(char* cDate, int& month, int& day, int& year);
char menu();
int main()
{
//vectors for storage
vector<Student>students;
vector<Faculty>faculty;
string courseName;
//name of course
cout << "Enter a name for your course: ";
getline(cin, courseName);
cout << endl;
// getmenu
char ch = menu();
//Loop repeat until quit
while (ch !='Q')
{
if (ch=='I'){
addFaculty (faculty);
}
else if (ch =='S'){
addStudent(students);
}
else if (ch=='L'){
ListCourse(courseName, students, faculty);
}
// loop repetition
cout<<endl;
ch = menu();
}
return 0;
}
//display choices and return choice
char menu()
{
char ch;
cout << " Main Menu " << endl;
cout << "I -- Add Instructor" << endl;
cout << "S -- Add Student" << endl;
cout << "L -- List Course Information" << endl;
cout << "Q -- Quit\n\n" << endl;
cout << "Selection: ";
cin>>ch;
return toupper(ch);
}
void addStudent() //example function that uses tokenizeDate
{
Date m, d, y;
char charDate[20]; //holds the date the user entered in char array
int pID;
Date bDate;
Date sEnrolled;
string fName, lName, sMajor, sLevel;
double sGPA;
Date realDate; //date object; holds date after tokenization
cout << "\nEnter a date (mm/dd/yyyy): ";
cin >> charDate;
cout<<"ID\n:";
cin>>pID;
cout<<"\nFirst Name:";
cin>>fName;
cout<<"\nLast Name:";
cin>>lName;
cout<<"\nMajor:";
cin>>sMajor;
cout<<"\nLevel (Freshman, Sophmore, Junior, Senior):";
cin>>sLevel;
cout<<"\nGPA:";
cin>>sGPA;
}
tokenizeDate(Date, m, d, y);
Date.setDate(m,d, y);
}
//Split date into day,month and date
void tokenizeDate(char* cDate, int& month, int& day, int& year)
{
char seps[] = "/";
char* token = NULL;
char* next_token = NULL;
token = NULL;
next_token = NULL;
// Establish string and get the tokens:
token = strtok_s(cDate, seps, &next_token);
month = atoi(token);
token = strtok_s(NULL, seps, &next_token);
day = atoi(token);
token = strtok_s(NULL, seps, &next_token);
year = atoi(token);
}

Step by step
Solved in 2 steps with 2 images

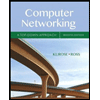
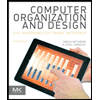
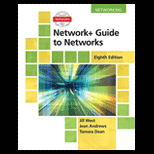
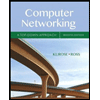
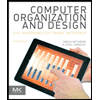
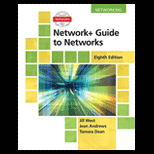
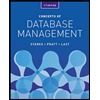
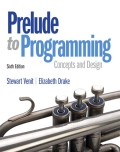
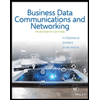