// need for using JavaFx import javafx.application.Application; import javafx.fxml.FXMLLoader; import javafx.geometry.*; import javafx.scene.Parent; import javafx.scene.Scene; import javafx.scene.control.Label; import javafx.geometry.Insets; import javafx.geometry.Pos; import javafx.scene.Scene; import javafx.scene.control.Button; import javafx.scene.control.Label; import javafx.scene.control.TextField; import javafx.scene.layout.GridPane; import javafx.stage.Stage; public class Main extends Application { atOverride public void start(Stage primaryStage) throws Exception { primaryStage.setTitle("Tip Calculator"); //setting the title GridPane rootNode = new GridPane(); rootNode.setPadding(new Insets(15)); Scene myScene = new Scene(rootNode, 300, 200); //create a scene rootNode.add(new Label("Amount:"), 0, 0); TextField firstValue = new TextField(); rootNode.add(firstValue, 1, 0); rootNode.add(new Label("Toatal is:"), 0, 5); Button aButton = new Button("Calculate"); rootNode.add(aButton, 1, 2); GridPane.setHalignment(aButton, HPos.LEFT); //set the alignment TextField result = new TextField(); // label for result result.setEditable(false); rootNode.add(result, 1, 5); TextField tax = new TextField(); rootNode.add(new Label("Tax:"), 0, 3); // label for tax tax.setEditable(false); rootNode.add(tax,1,3); TextField tip = new TextField(); rootNode.add(new Label("Tip:"), 0, 4); //label for tip tip.setEditable(false); rootNode.add(tip,1,4); aButton.setOnAction(e ->{ Float value1 = Float.valueOf(firstValue.getText()); Float value2 =(value1*18)/100; //calculating tip Float value3 = (value1*7)/100; //calculating tax Float r = value1+value2+value3 ; // total of all three amounts tax.setText(value3.toString()); tip.setText(value2.toString()); result.setText(r.toString()); } } primaryStage.setScene(myScene); // add scene to stage primaryStage.show(); // show window public static void main(String[] args) { // launch the application launch(args); } }
Please check if there is any issue with my code and please upload a screenshot of result when it is working.
Please add proper comments as well.
// need for using JavaFx
import javafx.application.Application;
import javafx.fxml.FXMLLoader;
import javafx.geometry.*;
import javafx.scene.Parent;
import javafx.scene.Scene;
import javafx.scene.control.Label;
import javafx.geometry.Insets;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.Label;
import javafx.scene.control.TextField;
import javafx.scene.layout.GridPane;
import javafx.stage.Stage;
public class Main extends Application
{
atOverride
public void start(Stage primaryStage) throws Exception
{
primaryStage.setTitle("Tip Calculator"); //setting the title
GridPane rootNode = new GridPane();
rootNode.setPadding(new Insets(15));
Scene myScene = new Scene(rootNode, 300, 200); //create a scene
rootNode.add(new Label("Amount:"), 0, 0);
TextField firstValue = new TextField();
rootNode.add(firstValue, 1, 0);
rootNode.add(new Label("Toatal is:"), 0, 5);
Button aButton = new Button("Calculate");
rootNode.add(aButton, 1, 2);
GridPane.setHalignment(aButton, HPos.LEFT); //set the alignment
TextField result = new TextField(); // label for result
result.setEditable(false);
rootNode.add(result, 1, 5);
TextField tax = new TextField();
rootNode.add(new Label("Tax:"), 0, 3); // label for tax
tax.setEditable(false);
rootNode.add(tax,1,3);
TextField tip = new TextField();
rootNode.add(new Label("Tip:"), 0, 4); //label for tip
tip.setEditable(false);
rootNode.add(tip,1,4);
aButton.setOnAction(e ->{
Float value1 = Float.valueOf(firstValue.getText());
Float value2 =(value1*18)/100; //calculating tip
Float value3 = (value1*7)/100; //calculating tax
Float r = value1+value2+value3 ; // total of all three amounts
tax.setText(value3.toString());
tip.setText(value2.toString());
result.setText(r.toString());
}
}
primaryStage.setScene(myScene); // add scene to stage
primaryStage.show(); // show window
public static void main(String[] args)
{
// launch the application
launch(args);
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

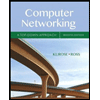
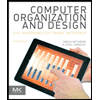
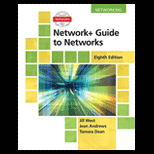
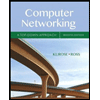
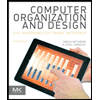
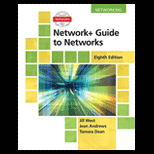
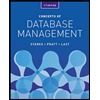
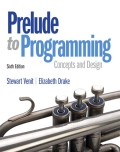
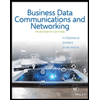