multithreaded Java program
Write a multithreaded Java program that uses synchronized methods (Section 7.4.1), reentrant locks (Section 7.4.2), or semaphores (Section 7.4.3) to control access to a one- lane bridge. One thread should simulate westbound vehicles and another thread should simulate eastbound vehicles. The vehicles do not have a reverse gear, so the bridge would become deadlocked if both a westbound vehicle and an eastbound vehicle were allowed to drive onto the bridge at the same time. Therefore, mutual exclusion must be enforced on the use of the bridge. Your solution should avoid both deadlock and starvation (e.g. the bridge being monopolized by westbound vehicles while the eastbound vehicles never get to cross). Vehicles traveling in either direction should wait (sleep) for some amount of time, then attempt to cross. Once a vehicle is on the bridge, it should sleep for some amount of time to simulate how long it takes to drive across the bridge.
Output a message when each vehicle drives onto the bridge and another message when that vehicle has completed the crossing. Simulate several (at least five) vehicles traveling in each direction.
Per the images below, I have most of the code finished. But for some reason the output is only giving me the threads entering the bridge sequentially and not from both sides. There is an issue with the mutual exclusion that I cannot figure out. Please help.
![O SynchronizedTrafficTester.java > q$ SynchronizedTrafficTester > O main(String[])
import java.util.concurrent.Semaphore;
1.
2
public class SynchronizedTrafficTester {
4
Run | Debug
public static void main(String [] args) {
final int NUM_CARS = 5;
//SynchronizedTraffic syncTraf = new SynchronizedTraffic();
Semaphore bridge
6
9
new Semaphore(1);
10
Thread [] eastBoundCars = new Thread [NUM_CARS]; //creates an array of threads for east bound cars
for (int i = 0; i < eastBoundCars.length; i++) {
11
12
13
eastBoundCars [i]
new Thread (new Car());
//5 cars in the threads
eastBoundCars [i].start();
14
15
16
Thread [] westBoundCars = new Thread [NUM_CARS]; //creates an array of threads for west bound cars
for (int i = 0; i < westBoundCars.length; i++) {
17
18
19
westBoundCars[i]
new Thread (new Car());
//5 cars in the threads
20
westBoundCars [i].start();
21
22
23
24
25
26
}
27
}
28](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fac160166-f98b-4282-b387-48f44a1bf16f%2F656c69be-e6f9-4a65-8983-c736975987ac%2Fw014jz_processed.png&w=3840&q=75)


Trending now
This is a popular solution!
Step by step
Solved in 3 steps

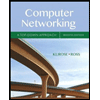
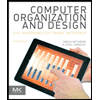
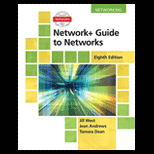
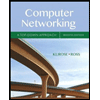
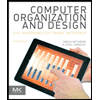
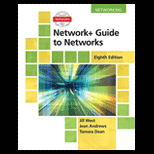
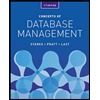
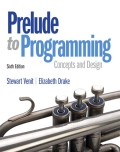
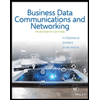