Java Program using Replit, please help. I circled the problem with red, because whenever I run the program, the entire output doesn't show and it tells me that "./Main.java uses unchecked or unsafe operations". How can I stop that from happening? Program in the photos.
Java Program using Replit, please help. I circled the problem with red, because whenever I run the program, the entire output doesn't show and it tells me that "./Main.java uses unchecked or unsafe operations". How can I stop that from happening? Program in the photos.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Java Program using Replit, please help. I circled the problem with red, because whenever I run the program, the entire output doesn't show and it tells me that "./Main.java uses unchecked or unsafe operations". How can I stop that from happening?
Program in the photos.
![```java
57 for (int u = 0; u < V; u++)
58
59 // Doesn't recur if already visited
60 if (!visited[u])
61 if (isCyclicUtil(u, visited, -1))
62 return true;
63 return false;
64 }
65
66 // Driver Method
67 public static void main(String[] args) {
68 // Creates a graph
69 Main g1 = new Main(5);
70 g1.addEdge(1, 0);
71 g1.addEdge(0, 2);
72 g1.addEdge(2, 0);
73 g1.addEdge(0, 3);
74 g1.addEdge(3, 4);
75 if (g1.isCyclic())
76 System.out.println("Graph contains a cycle");
77 else
78 System.out.println("Graph doesn't contain a cycle");
79 Main g2 = new Main(3);
80 g2.addEdge(0, 1);
81 g2.addEdge(1, 2);
82 if (g2.isCyclic())
83 System.out.println("Graph contains a cycle");
84 else
85 System.out.println("Graph doesn't contain a cycle");
86 }
87 }
88
```
### Description
This Java code snippet is part of a program designed to detect cycles in a graph. The cycle detection is performed using Depth First Search (DFS).
- **Lines 57-64:** This loop iterates through all vertices and checks if a cycle exists in the graph using a utility function `isCyclicUtil`.
- **Lines 66-88:** The `main` method is the entry point of the program. It creates two graphs, `g1` and `g2`, using the `Main` class constructor which seems to represent the number of vertices.
- **Graph `g1`:** Contains 5 vertices. Edges are added between vertices, creating potential cycles.
- **Graph `g2`:** Contains 3 vertices. Edges are added sequentially, making it acyclic.
After adding edges, the `isCyclic` method checks each graph for cycles and outputs the results to the console.
This example demonstrates](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F4cd5838b-3f34-487e-9642-7770197fe05c%2F79634c60-80ea-4b68-a935-181b01cf9d6b%2Ffz1z65k_processed.png&w=3840&q=75)
Transcribed Image Text:```java
57 for (int u = 0; u < V; u++)
58
59 // Doesn't recur if already visited
60 if (!visited[u])
61 if (isCyclicUtil(u, visited, -1))
62 return true;
63 return false;
64 }
65
66 // Driver Method
67 public static void main(String[] args) {
68 // Creates a graph
69 Main g1 = new Main(5);
70 g1.addEdge(1, 0);
71 g1.addEdge(0, 2);
72 g1.addEdge(2, 0);
73 g1.addEdge(0, 3);
74 g1.addEdge(3, 4);
75 if (g1.isCyclic())
76 System.out.println("Graph contains a cycle");
77 else
78 System.out.println("Graph doesn't contain a cycle");
79 Main g2 = new Main(3);
80 g2.addEdge(0, 1);
81 g2.addEdge(1, 2);
82 if (g2.isCyclic())
83 System.out.println("Graph contains a cycle");
84 else
85 System.out.println("Graph doesn't contain a cycle");
86 }
87 }
88
```
### Description
This Java code snippet is part of a program designed to detect cycles in a graph. The cycle detection is performed using Depth First Search (DFS).
- **Lines 57-64:** This loop iterates through all vertices and checks if a cycle exists in the graph using a utility function `isCyclicUtil`.
- **Lines 66-88:** The `main` method is the entry point of the program. It creates two graphs, `g1` and `g2`, using the `Main` class constructor which seems to represent the number of vertices.
- **Graph `g1`:** Contains 5 vertices. Edges are added between vertices, creating potential cycles.
- **Graph `g2`:** Contains 3 vertices. Edges are added sequentially, making it acyclic.
After adding edges, the `isCyclic` method checks each graph for cycles and outputs the results to the console.
This example demonstrates
![```java
import java.util.LinkedList;
import java.util.Iterator;
class Main {
// Number of Vertices
private int V;
// Adjacency Lists
private LinkedList<Integer> adj[];
// Constructor
Main(int v) {
V = v;
adj = new LinkedList[v];
for (int i = 0; i < v; ++i)
adj[i] = new LinkedList();
}
// Function that adds an edge to the graph
void addEdge(int v, int w) {
adj[v].add(w);
adj[w].add(v);
}
// A recursive function that uses visited[] and parent to detect cycle in subgraph reachable from vertex v.
Boolean isCyclicUtil(int v, Boolean visited[], int parent) {
// Marks the current node as visited
visited[v] = true;
Integer i;
// Recur for all the vertices adjacent to this vertex
Iterator<Integer> it = adj[v].iterator();
while (it.hasNext()) {
i = it.next();
// If an adjacent is not visited, then recur for that adjacent
if (!visited[i]) {
if (isCyclicUtil(i, visited, v))
return true;
}
// If an adjacent is visited and not parent of current vertex, then there is a cycle
else if (i != parent)
return true;
}
return false;
}
// Returns true if the graph contains a cycle, else false.
Boolean isCyclic() {
// Mark all the vertices as not visited and not part of recursion stack
Boolean visited[] = new Boolean[V];
for (int i = 0; i < V; i++)
visited[i] = false;
// Call the recursive helper function to detect cycle in different DFS trees
```
### Explanation
This code defines a structure and methods to represent a graph using adjacency lists and includes functionality to detect cycles within the graph. Key components include:
- **Vertices and Adjacency Lists:** The graph's vertices and their connections are stored using an array of `LinkedList` objects where each index represents a vertex and the linked list at that index contains the vertices adjacent to it.
- **Constructor:** Initializes the graph with a specified number of vertices and creates adjacency lists for each vertex.
- **addEdge](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F4cd5838b-3f34-487e-9642-7770197fe05c%2F79634c60-80ea-4b68-a935-181b01cf9d6b%2Flqtpvw_processed.png&w=3840&q=75)
Transcribed Image Text:```java
import java.util.LinkedList;
import java.util.Iterator;
class Main {
// Number of Vertices
private int V;
// Adjacency Lists
private LinkedList<Integer> adj[];
// Constructor
Main(int v) {
V = v;
adj = new LinkedList[v];
for (int i = 0; i < v; ++i)
adj[i] = new LinkedList();
}
// Function that adds an edge to the graph
void addEdge(int v, int w) {
adj[v].add(w);
adj[w].add(v);
}
// A recursive function that uses visited[] and parent to detect cycle in subgraph reachable from vertex v.
Boolean isCyclicUtil(int v, Boolean visited[], int parent) {
// Marks the current node as visited
visited[v] = true;
Integer i;
// Recur for all the vertices adjacent to this vertex
Iterator<Integer> it = adj[v].iterator();
while (it.hasNext()) {
i = it.next();
// If an adjacent is not visited, then recur for that adjacent
if (!visited[i]) {
if (isCyclicUtil(i, visited, v))
return true;
}
// If an adjacent is visited and not parent of current vertex, then there is a cycle
else if (i != parent)
return true;
}
return false;
}
// Returns true if the graph contains a cycle, else false.
Boolean isCyclic() {
// Mark all the vertices as not visited and not part of recursion stack
Boolean visited[] = new Boolean[V];
for (int i = 0; i < V; i++)
visited[i] = false;
// Call the recursive helper function to detect cycle in different DFS trees
```
### Explanation
This code defines a structure and methods to represent a graph using adjacency lists and includes functionality to detect cycles within the graph. Key components include:
- **Vertices and Adjacency Lists:** The graph's vertices and their connections are stored using an array of `LinkedList` objects where each index represents a vertex and the linked list at that index contains the vertices adjacent to it.
- **Constructor:** Initializes the graph with a specified number of vertices and creates adjacency lists for each vertex.
- **addEdge
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 5 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
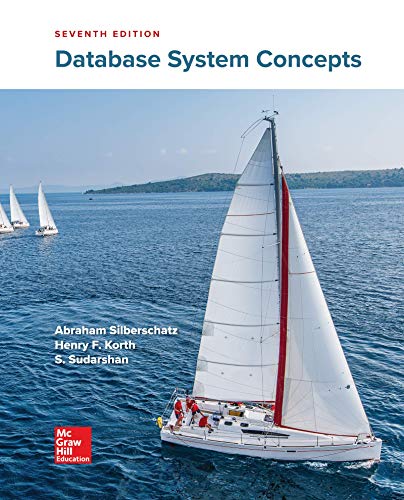
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
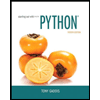
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
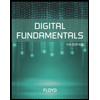
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
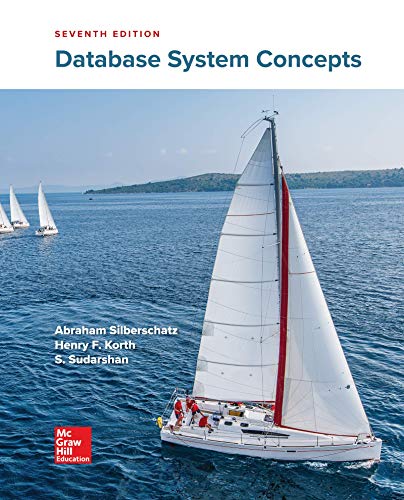
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
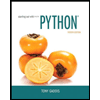
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
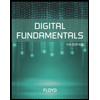
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
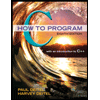
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
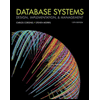
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
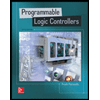
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education