The three possible ratings are 3, 2, 1 and they represent “Excellent”, “Good”, and “Poor”. An employee who is rated excellent will receive a 6% raise, one rated good will receive a 4% raise, and one rated poor will receive a 1.5% raise. At last, the program computes the raise for the employee based on the performance. Use if statements to complete the program. Test your program several times with different inputs.
Utilize Salary.java. The file contains a
Two input values are read in:
-
an employee’s current salary
-
a rating of the employee’s performance
// ************************************************************
// Salary.java
// ************************************************************
import java.util.Scanner;
import java.text.NumberFormat;
public class Salary
{
public static void main (String[] args)
{
double currentSalary; // employee's current salary
double raise; // amount of the raise
double newSalary; // new salary for the employee
int rating; // performance rating
Scanner scan = new Scanner(System.in);
System.out.print ("Enter the current salary: ");
currentSalary = scan.nextDouble();
System.out.print ("Enter the performance rating (3 is Excellent, 2 is Good, or 1 is Poor): ");
rating = scan.nextInt();
// Enter the code here: compute the raise using if/else-if/else statements to deal with three rating levels
newSalary = currentSalary + raise;
// Print the results
NumberFormat money = NumberFormat.getCurrencyInstance();
System.out.println();
System.out.println("Current Salary: " + money.format(currentSalary));
System.out.println("Amount of your raise: " + money.format(raise));
System.out.println( "Your new salary: " + money. format (newSalary) );
System.out.println();
}
}
The three possible ratings are 3, 2, 1 and they represent “Excellent”, “Good”, and “Poor”. An employee who is rated excellent will receive a 6% raise, one rated good will receive a 4% raise, and one rated poor will receive a 1.5% raise.
At last, the program computes the raise for the employee based on the performance.
Use if statements to complete the program. Test your program several times with different inputs.

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 5 images

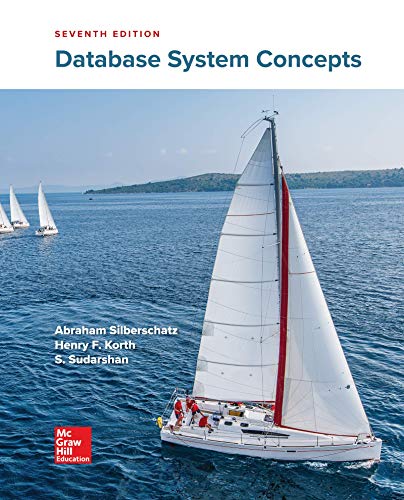
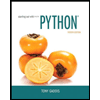
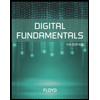
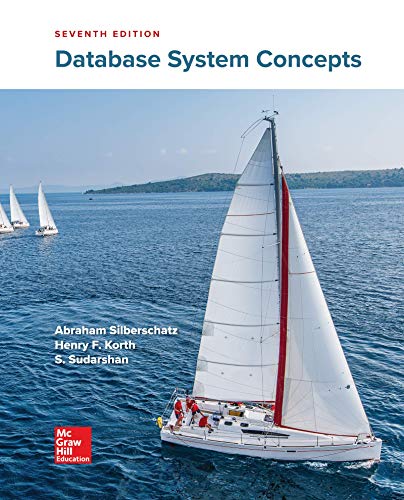
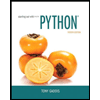
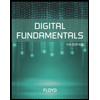
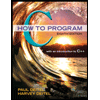
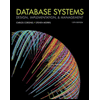
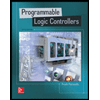