Modify LiveExample 12.12 EvaluateExpression.cpp to add operators ^ for exponent and % for modulus. For example, 3 ^ 2 is 9 and 3 % 2 is 1. The ^ operator has the highest precedence and the % operator has the same precedence as the * and / operators. For simplicity, assume the expression has at most one ^ operator. Sample Run: Enter an expression: (5 * 2 ^ 3 + 2 * 3 % 2) * 4 (5 * 2 ^ 3 + 2 * 3 % 2) * 4 = 160 Code posted below, c++ please! #include #include #include using namespace std; #ifndef STACK_H #define STACK_H template class Stack { public: Stack(); bool empty() const; T peek() const; void push(T value); T pop(); int getSize() const; private: vector elements; }; template Stack::Stack() { } template bool Stack::empty() const { return elements.size() == 0; } template T Stack::peek() const { return elements[elements.size() - 1]; } template void Stack::push(T value) { elements.push_back(value); } template T Stack::pop() { T temp = elements[elements.size() - 1]; elements.pop_back(); return temp; } template int Stack::getSize() const { return elements.size(); } #endif #include #include using namespace std; // Split an expression into numbers, operators, and parenthese vector split(const string& expression); // Evaluate an expression and return the result int evaluateExpression(const string& expression); // Perform an operation void processAnOperator(Stack& operandStack, Stack& operatorStack); int main() { string expression; cout << "Enter an expression: "; getline(cin, expression); cout << expression << " = " << evaluateExpression(expression) << endl; return 0; } vector split(const string& expression) { vector v; // A vector to store split items as strings string numberString; // A numeric string for (unsigned int i = 0; i < expression.length(); i++) { if (isdigit(expression[i])) numberString.append(1, expression[i]); // Append a digit else { if (numberString.size() > 0) { v.push_back(numberString); // Store the numeric string numberString.erase(); // Empty the numeric string } if (!isspace(expression[i])) { string s; s.append(1, expression[i]); v.push_back(s); // Store an operator and parenthesis } } } // Store the last numeric string if (numberString.size() > 0) v.push_back(numberString); return v; } // Evaluate an expression int evaluateExpression(const string& expression) { } // Process one operator: Take an operator from operatorStack and // apply it on the operands in the operandStack void processAnOperator( Stack& operandStack, Stack& operatorStack) { }
#include <vector>
#include <iostream>
#include <cmath>
using namespace std;
#ifndef STACK_H
#define STACK_H
template<typename T>
class Stack
{
public:
Stack();
bool empty() const;
T peek() const;
void push(T value);
T pop();
int getSize() const;
private:
vector<T> elements;
};
template<typename T>
Stack<T>::Stack()
{
}
template<typename T>
bool Stack<T>::empty() const
{
return elements.size() == 0;
}
template<typename T>
T Stack<T>::peek() const
{
return elements[elements.size() - 1];
}
template<typename T>
void Stack<T>::push(T value)
{
elements.push_back(value);
}
template<typename T>
T Stack<T>::pop()
{
T temp = elements[elements.size() - 1];
elements.pop_back();
return temp;
}
template<typename T>
int Stack<T>::getSize() const
{
return elements.size();
}
#endif
#include <string>
#include <cctype>
using namespace std;
// Split an expression into numbers, operators, and parenthese
vector<string> split(const string& expression);
// Evaluate an expression and return the result
int evaluateExpression(const string& expression);
// Perform an operation
void processAnOperator(Stack<int>& operandStack, Stack<char>& operatorStack);
int main()
{
string expression;
cout << "Enter an expression: ";
getline(cin, expression);
cout << expression << " = "
<< evaluateExpression(expression) << endl;
return 0;
}
vector<string> split(const string& expression)
{
vector<string> v; // A vector to store split items as strings
string numberString; // A numeric string
for (unsigned int i = 0; i < expression.length(); i++)
{
if (isdigit(expression[i]))
numberString.append(1, expression[i]); // Append a digit
else
{
if (numberString.size() > 0)
{
v.push_back(numberString); // Store the numeric string
numberString.erase(); // Empty the numeric string
}
if (!isspace(expression[i]))
{
string s;
s.append(1, expression[i]);
v.push_back(s); // Store an operator and parenthesis
}
}
}
// Store the last numeric string
if (numberString.size() > 0)
v.push_back(numberString);
return v;
}
// Evaluate an expression
int evaluateExpression(const string& expression)
{
}
// Process one operator: Take an operator from operatorStack and
// apply it on the operands in the operandStack
void processAnOperator(
Stack<int>& operandStack, Stack<char>& operatorStack)
{
}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 5 images

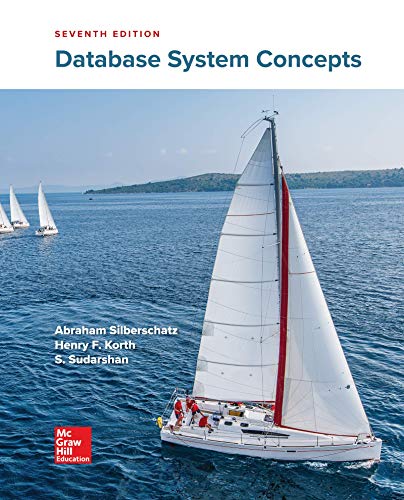
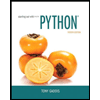
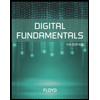
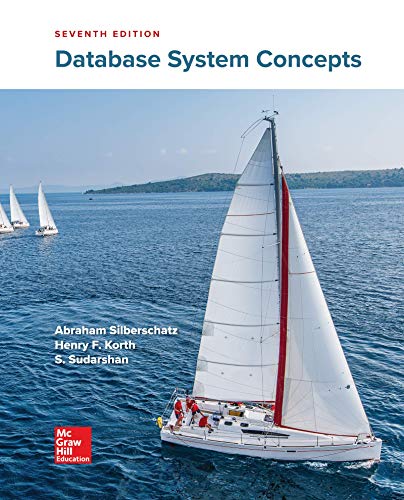
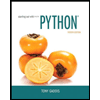
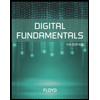
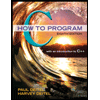
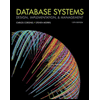
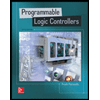