I was provided with the following code. However, when executing it goes to an infite loop and cannot seem to ppass by that. public int compares(Card c1, Card c2){ // TODO: implement this method if(c1.getSuit() > c2.getSuit()) return 1; if(c1.getSuit() < c2.getSuit()) return -1; else { if(c1.getRank() > c2.getRank()) return 1; if (c1.getRank() < c2.getRank()) return -1; else return 0; } public void quickSortRec(Card[] cardArray, int first, int last) { if (first < last) { int splitPoint; splitPoint = split(cardArray, first, last); quickSortRec(cardArray, first, splitPoint); quickSortRec(cardArray, splitPoint + 1, last); } } public int split(Card[] cardArray, int first, int last){ Card splitValue = cardArray[first]; while(first <= last) { while(compares(cardArray[first],splitValue) == -1) { first++; } while(compares(cardArray[last],splitValue) == 1) { last--; } if (first <= last) { Card temp = cardArray[first]; cardArray[first] = cardArray[last]; cardArray[last] = temp; first++; last--; } }
I was provided with the following code. However, when executing it goes to an infite loop and cannot seem to ppass by that.
public int compares(Card c1, Card c2){
// TODO: implement this method
if(c1.getSuit() > c2.getSuit())
return 1;
if(c1.getSuit() < c2.getSuit())
return -1;
else
{
if(c1.getRank() > c2.getRank())
return 1;
if (c1.getRank() < c2.getRank())
return -1;
else
return 0;
}
public void quickSortRec(Card[] cardArray, int first, int last) {
if (first < last) {
int splitPoint;
splitPoint = split(cardArray, first, last);
quickSortRec(cardArray, first, splitPoint);
quickSortRec(cardArray, splitPoint + 1, last);
}
}
public int split(Card[] cardArray, int first, int last){
Card splitValue = cardArray[first];
while(first <= last) {
while(compares(cardArray[first],splitValue) == -1) {
first++;
}
while(compares(cardArray[last],splitValue) == 1) {
last--;
}
if (first <= last)
{
Card temp = cardArray[first];
cardArray[first] = cardArray[last];
cardArray[last] = temp;
first++;
last--;
}
}
return first - 1;
}


Trending now
This is a popular solution!
Step by step
Solved in 2 steps

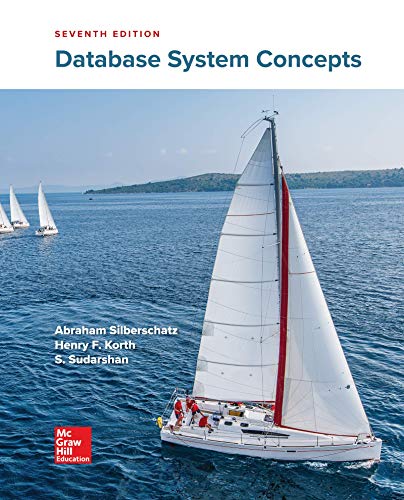
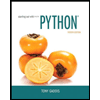
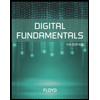
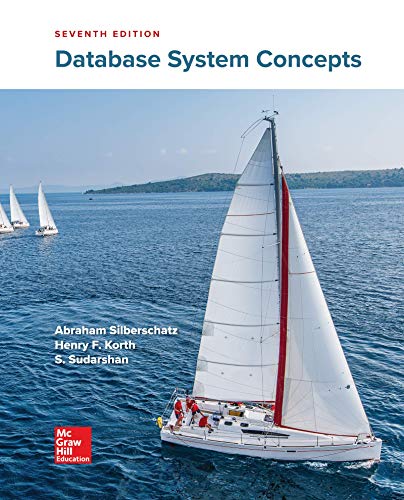
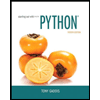
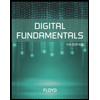
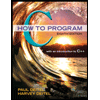
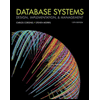
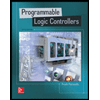